Integrate SmartUI SDK with Testcafe Tests
Welcome to the world of simplified visual testing with the SmartUI SDK.
Integrating seamlessly into your existing Testcafe testing suite, SmartUI SDK revolutionizes the way you approach visual regression testing. Our robust solution empowers you to effortlessly capture, compare, and analyze screenshots across a multitude of browsers and resolutions, ensuring comprehensive coverage and accuracy in your visual testing endeavors.
Prerequisites
- Basic understanding of Command Line Interface and Testcafe is required.
- Login to LambdaTest SmartUI with your credentials.
The following steps will guide you in running your first Visual Regression test on LambdaTest platform using SmartUI Testcafe SDK integration.
Create a SmartUI Project
The first step is to create a project with the application in which we will combine all your builds run on the project. To create a SmartUI Project, follow these steps:
- Go to Projects page
- Click on the
new project
button - Select the platform as CLI for executing your
SDK
tests. - Add name of the project, approvers for the changes found, tags for any filter or easy navigation.
- Click on the Submit.
Steps to run your first test
Once you have created a SmartUI Project, you can generate screenshots by running automation scripts. Follow the below steps to successfully generate screenshots
Step 1: Create/Update your test
You can clone the sample repository to run Testcafe
tests with SmartUI
and use the testcafeSDKLocal.js
file.
git clone https://github.com/LambdaTest/smartui-testcafe-sample
cd smartui-testcafe-sample
Step 2: Install the Dependencies
Install required NPM modules for LambdaTest Smart UI Testcafe SDK
in your Frontend project.
npm i @lambdatest/smartui-cli @lambdatest/testcafe-driver testcafe
Step 3: Configure your Project Token
Setup your project token show in the SmartUI app after, creating your project.
- MacOS/Linux
- Windows - CMD
- Windows-PS
export PROJECT_TOKEN="123456#1234abcd-****-****-****-************"
set PROJECT_TOKEN="123456#1234abcd-****-****-****-************"
$Env:PROJECT_TOKEN="123456#1234abcd-****-****-****-************"
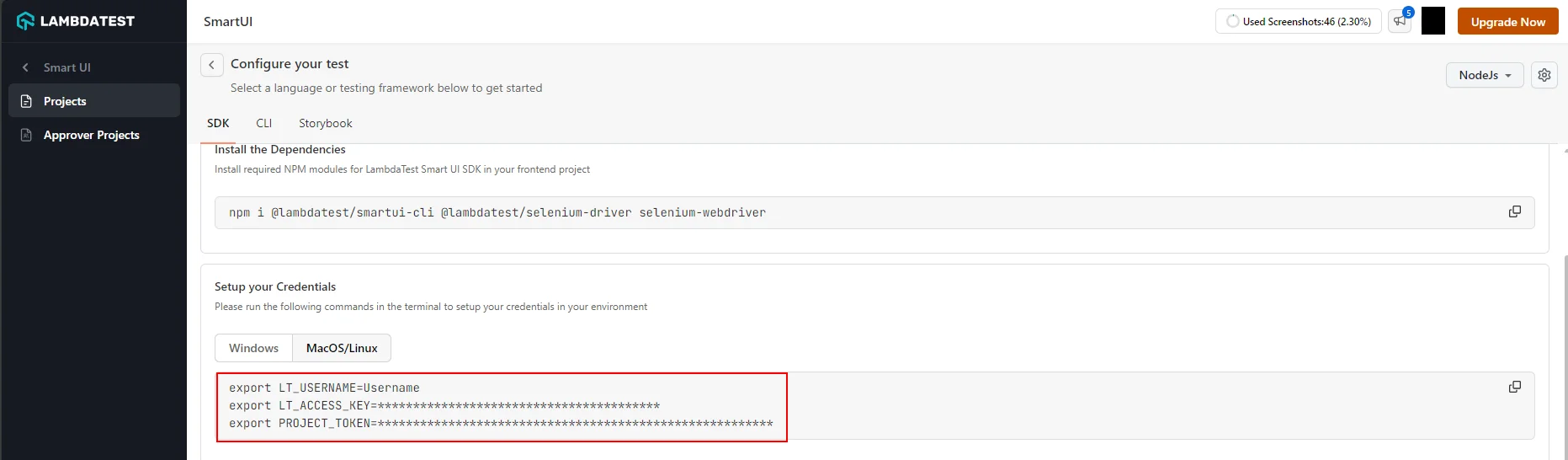
Step 4: Create and Configure SmartUI Config
You can now configure your project configurations on using various available options to run your tests with the SmartUI integration. To generate the configuration file, please execute the following command:
npx smartui config:create .smartui.json
Once, the configuration file will be created, you will be seeing the default configuration pre-filled in the configuration file:
{
"web": {
"browsers": [
"chrome",
"firefox",
"safari",
"edge"
],
"viewports": [
[
1920
],
[
1366
],
[
1028
]
] // Full Page screenshots are captured by default for web viewports
},
"mobile": {
"devices": [
"iPhone 14", //iPhone 14 viewport
"Galaxy S24" //Galaxy S24 viewport
],
"fullPage": true, //Full Page is true by default for mobile viewports
"orientation": "portrait" //Change to "landscape" for landscape snapshot
},
"waitForTimeout": 1000, //Optional (Should only be used in case lazy-loading/async components are present)
"waitForPageRender": 50000, //Optional (Should only be used in case of websites which take more than 30s to load)
"enableJavaScript": false, //Enable javascript for all the screenshots of the project
"allowedHostnames": [] //Additional hostnames to capture assets from
}
- For capturing fullpage or viewport screenshots, please refer to this documentation
- For the list of available mobile viewports, please refer to this documentation
- For more information about SmartUI config global options, please refer to this documentation.
Step 5: Adding SmartUI function to take screenshot
- You can incorporate SmartUI into your custom
Testcafe
automation test (any platform) script by adding thesmartuiSnapshot
function in the required segment of testcafe script of which we would like to take the screenshot, as shown below:
import { Selector } from 'testcafe';
import { smartuiSnapshot } from '@lambdatest/testcafe-driver';
fixture('Amazon Test')
.page('https://www.lambdatest.com');
test('Take Amazon Homepage Screenshot', async (t) => {
// Take a screenshot using LambdaTest's TestCafe driver
await smartuiSnapshot(t, 'LT-Homepage');
});
Step 6: Execute the Tests on SmartUI Cloud
Execute visual regression tests
on SmartUI using the following commands
npx smartui exec testcafe chrome testcafeSDKLocal.js --config .smartui.json
You may use the npx smartui --help
command in case you are facing issues during the execution of SmartUI commands in the CLI.
View SmartUI Results
You have successfully integrated SmartUI SDK with your Testcafe tests. Visit your SmartUI project to view builds and compare snapshots between different test runs.
You can see the Smart UI dashboard to view the results. This will help you identify the Mismatches from the existing Baseline
build and do the required visual testing.
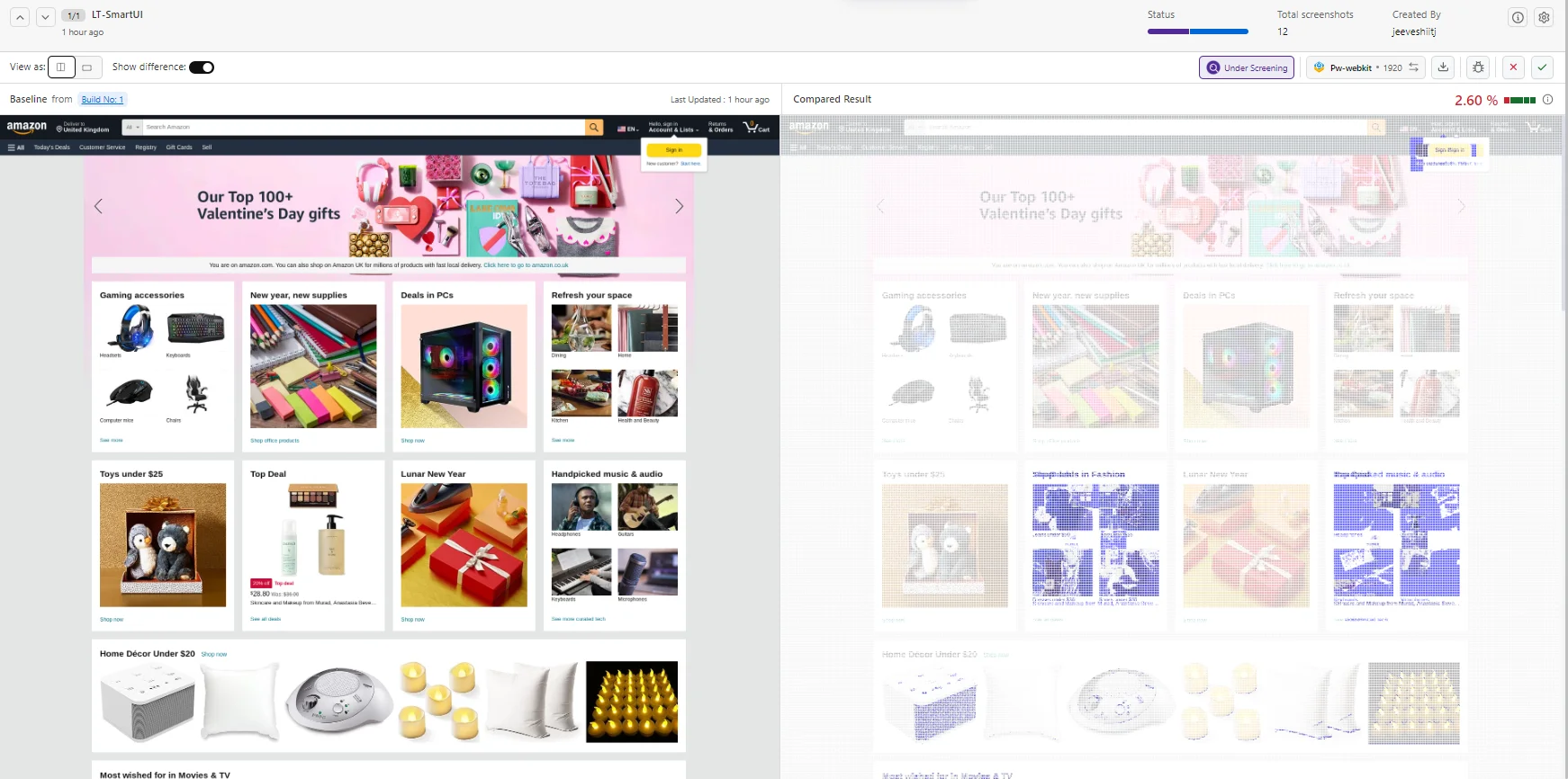
Arguments supported in the smartUISnapshot
function
The following are the different options which are currently supported:
Key | Description |
---|---|
t (test controller) | This object allows you to control the test execution and interact with the web page under test. |
"Screenshot Name" (string) | Specify a name for the screenshot in your tests to match the same screenshot with the name from your baseline. |
options (object) | Specify one or a combination of selectors in the ignoreDOM or selectDOM objects. These selectors can be based on HTML DOM IDs, CSS classes, CSS selectors, or XPaths used by your webpage. They define elements that should be excluded from or included in the visual comparison. |
Handling Dynamic Data in SmartUI SDK New
When conducting visual tests, you may encounter scenarios where certain elements within your application change between test runs. These changes might introduce inconsistencies in your test results.You can ignore / select specific element(s) to be removed from the comparison by parsing the options in the smartuiSnapshot
function in the following way
- Ignore ID
- Ignore Class
- Ignore XPath
- Ignore CSS Selector
let options = {
ignoreDOM: {
id: ["ID-1", "ID-2"],
}
}
await smartuiSnapshot(t, 'Screenshot Name', options);
let options = {
ignoreDOM: {
class: ["Class-1", "Class-2"],
}
}
await smartuiSnapshot(t, 'Screenshot Name', options);
let options = {
ignoreDOM: {
xpath: ["Xpath-1", "Xpath-2"],
}
}
await smartuiSnapshot(t, 'Screenshot Name', options);
let options = {
ignoreDOM: {
cssSelector: ["CSS-Selector-1", "CSS-Selector-2"],
}
}
await smartuiSnapshot(t, 'Screenshot Name', options);
- Select ID
- Select Class
- Select XPath
- Select CSS Selector
let options = {
selectDOM: {
id: ["ID-1", "ID-2"],
}
}
await smartuiSnapshot(t, 'Screenshot Name', options);
let options = {
selectDOM: {
class: ["Class-1", "Class-2"],
}
}
await smartuiSnapshot(t, 'Screenshot Name', options);
let options = {
selectDOM: {
xpath: ["Xpath-1", "Xpath-2"],
}
}
await smartuiSnapshot(t, 'Screenshot Name', options);
let options = {
selectDOM: {
cssSelector: ["CSS-Selector-1", "CSS-Selector-2"],
}
}
await smartuiSnapshot(t, 'Screenshot Name', options);
For capturing the screenshot of a specific element
You can capture screenshots of targeted elements by leveraging various locator mechanisms such as XPath, CSS ID, class, and selectors. This precision-driven approach ensures accurate and specific visual regression testing for your web application's components.
- Capture Element by ID
- Capture Element by Class
- Capture Element by XPath
- Capture Element by Selector
let options = {
element: {
id: 'Required ID',
}
};
await smartuiSnapshot(t, 'Screenshot Name', options);
let options = {
element: {
class: 'Required Class',
}
};
await smartuiSnapshot(t, 'Screenshot Name', options);
let options = {
element: {
xpath: 'Required Xpath',
}
};
await smartuiSnapshot(t, 'Screenshot Name', options);
let options = {
element: {
cssSelector: 'Required CSS Selector',
}
};
await smartuiSnapshot(t, 'Screenshot Name', options);
For capturing interactive lazy loading elements
If you encounter difficulties loading interactive elements that appear on scroll in full-page screenshots, consider functionally incorporating a full-page scroll into your script before capturing the screenshot. This approach ensures the elements load first, facilitating the screenshot processing.
import { Selector } from 'testcafe';
import { smartuiSnapshot } from '@lambdatest/testcafe-driver';
// Function to scroll through the page and then back to the top
async function scrollToBottomAndTop(t, lastPageWait = 100) {
const getScrollHeight = Selector(() => document.body.scrollHeight);
const heightOfPage = await getScrollHeight();
let size = 200;
let noOfLoop = Math.floor(heightOfPage / size);
for (let i = 1; i <= noOfLoop; i++) {
await t.scrollBy({ x: 0, y: size });
await t.wait(1000);
if (i === noOfLoop) {
await t.scrollTo({ x: 0, y: heightOfPage });
await t.wait(lastPageWait);
}
}
// Scroll to the top
await t.scrollTo({ x: 0, y: 0 });
await t.wait(10000);
console.log("Scroll Completed");
}
fixture `Page Scroll Test`
.page `Required URL`; // Replace `Required URL` with the actual URL
test('Scroll through the page, then take a smartUI Snapshot', async t => {
await scrollToBottomAndTop(t, 100); // Adjust wait time as needed
await smartuiSnapshot(t, 'Screenshot Name'); // Adjust the screenshot name as needed
});
For additional information about SmartUI APIs please explore the documentation here