Run Automation Tests on HyperExecute using TestNG
This page outlines how to execute your tests on HyperExecute using TestNG with YAML 0.2
HyperExecute uses YAML 0.2 to perform the tests using TestNG.
Overview
HyperExecute is a smart test orchestration platform that allows you to run end-to-end Selenium tests as quickly as possible by providing a test infrastructure with optimal speed, test orchestration, and detailed execution logs.
HyperExecute offers features like detailed logs, Smart CI, network insights, video recording, and access to various browsers and platforms.
Running Specific TestNG Tests
When working with TestNG, you may sometimes want to run specific tests instead of executing the entire test suite. Here's how you can achieve this with the provided Java-TestNG-Selenium repository on LambdaTest:
All the code samples in this documentation can be found on LambdaTest's Github Repository. You can either download or clone the repository to quickly run your tests. View on GitHub
Pre-requisite
- Install the Java Development Environment.
- Install Maven, which supports the TestNG framework out of the box. Maven can also be installed easily on Linux/MacOS using Homebrew package manager.
Cloning Repo and Installing Dependencies
Clone the LambdaTest's Java-TestNG-Selenium repository:
git clone https://github.com/LambdaTest/Java-TestNG-Selenium
cd Java-TestNG-Selenium
Setup Environment Variable
Export the environment variables LT_USERNAME and LT_ACCESS_KEY that are available in the LambdaTest Profile page. Run the below mentioned commands in the terminal to setup the CLI and the environment variables.
For macOS / Linux:
export LT_USERNAME=YOUR_LT_USERNAME
export LT_ACCESS_KEY=YOUR_LT_ACCESS_KEY
For Windows:
set LT_USERNAME=YOUR_LT_USERNAME
set LT_ACCESS_KEY=YOUR_LT_ACCESS_KEY
Integrate your 1st Test Case on LambdaTest
The Java-TestNG-Selenium repository provides a sample TestNG test that you can execute.
Step 1: Open the TestNGTodo.java file and update the username and accesskey variables with your LambdaTest credentials.
NOTE: Make sure to update the Hub endpoint using the variable gridURL.
By setting up the Hub endpoint, you establish the communication channel between your tests and the browser nodes, enabling effective test distribution and execution.
import org.openqa.selenium.By;
import org.openqa.selenium.Platform;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.remote.DesiredCapabilities;
import org.openqa.selenium.remote.RemoteWebDriver;
import org.testng.Assert;
import org.testng.annotations.AfterClass;
import org.testng.annotations.BeforeClass;
import org.testng.annotations.Test;
import java.net.MalformedURLException;
import java.net.URL;
public class TestNGTodo{
public String username = "YOUR_LAMBDATEST_USERNAME";
public String accesskey = "YOUR_LAMBDATEST_ACCESS_KEY";
public static RemoteWebDriver driver = null;
public String gridURL = "@hub.lambdatest.com/wd/hub"; //hub endpoint
boolean status = false;
@BeforeClass
public void setUp() throws Exception {
DesiredCapabilities capabilities = new DesiredCapabilities();
capabilities.setCapability("browserName", "chrome");
capabilities.setCapability("version", "70.0");
capabilities.setCapability("platform", "win10"); // If this cap isn't specified, it will just get the any available one
capabilities.setCapability("build", "LambdaTestSampleApp");
capabilities.setCapability("name", "LambdaTestJavaSample");
try {
driver = new RemoteWebDriver(new URL("https://" + username + ":" + accesskey + gridURL), capabilities);
} catch (MalformedURLException e) {
System.out.println("Invalid grid URL");
} catch (Exception e) {
System.out.println(e.getMessage());
}
}
@Test
public void testSimple() throws Exception {
try {//Change it to production page
driver.get("https://lambdatest.github.io/sample-todo-app/");
//Let's mark done first two items in the list.
driver.findElement(By.name("li1")).click();
driver.findElement(By.name("li2")).click();
// Let's add an item in the list.
driver.findElement(By.id("sampletodotext")).sendKeys("Yey, Let's add it to list");
driver.findElement(By.id("addbutton")).click();
// Let's check that the item we added is added in the list.
String enteredText = driver.findElementByXPath("/html/body/div/div/div/ul/li[6]/span").getText();
if (enteredText.equals("Yey, Let's add it to list")) {
status = true;
}
} catch (Exception e) {
System.out.println(e.getMessage());
}
}
@AfterClass
public void tearDown() throws Exception {
if (driver != null) {
((JavascriptExecutor) driver).executeScript("lambda-status=" + status);
driver.quit();
}
}
}
Step 2: Configure the desired capabilities based on your test requirements. For example:
DesiredCapabilities capabilities = new DesiredCapabilities();
capabilities.setCapability("browserName", "chrome");
capabilities.setCapability("version", "70.0");
capabilities.setCapability("platform", "win10"); // If this cap isn't specified, it will just get the any available one
capabilities.setCapability("build", "LambdaTestSampleApp");
capabilities.setCapability("name", "LambdaTestJavaSample");
You can generate capabilities for your test requirements with the help of our inbuilt 🔗 Capabilities Generator Tool.
Step 3: Save the changes to the TestNGTodo.java file.
Step 4: In the terminal, execute the test using the following command to run the test using the single.xml TestNG suite configuration.
mvn test -D suite=single.xml
Your test results would be displayed on the test console (or command-line interface if you are using terminal/cmd) and on LambdaTest automation dashboard. LambdaTest Automation Dashboard will help you view all your text logs, screenshots and video recording for your entire automation tests.
Running TestNG Tests on HyperExecute
TestNG Tests can be executed on HyperExecute Grid using 2 methods:
- Using Local System - Requires HyperExecute CLI to execute tests from your Local System.
- Using Gitpod - Execute tests using GitPod. (Requires a Gitpod account)
1. Testing Using Local System
Pre-requisites:
To run the Tests on HyperExecute from your Local System, you are required:
- Your lambdatest Username and Access key
- HyperExecute CLI in order to initiate a test execution Job.
- Setup the Environmental Variable
- HyperExecute YAML file which contains all the necessary instructions.
All the code samples in this documentation can be found on LambdaTest's Github Repository. You can either download or clone the repository to quickly run your tests. View on GitHub
Download HyperExecute CLI
The HyperExecute CLI is used for triggering tests on HyperExecute Grid. It is recommend to download the HyperExecute CLI binary on the host system to perform the tests on HyperExecute. The CLI download site for various platforms is displayed below:
Platform | HyperExecute CLI download location |
---|---|
Windows | https://downloads.lambdatest.com/hyperexecute/windows/hyperexecute.exe |
macOS | https://downloads.lambdatest.com/hyperexecute/darwin/hyperexecute |
Linux | https://downloads.lambdatest.com/hyperexecute/linux/hyperexecute |
Setup Environment Variable
Export the environment variables LT_USERNAME and LT_ACCESS_KEY that are available in the LambdaTest Profile page. Run the below mentioned commands in the terminal to setup the CLI and the environment variables.
For macOS:
export LT_USERNAME=YOUR_LT_USERNAME
export LT_ACCESS_KEY=YOUR_LT_ACCESS_KEY
For Linux:
export LT_USERNAME=YOUR_LT_USERNAME
export LT_ACCESS_KEY=YOUR_LT_ACCESS_KEY
For Windows:
set LT_USERNAME=YOUR_LT_USERNAME
set LT_ACCESS_KEY=YOUR_LT_ACCESS_KEY
Steps to execute the Tests
Follow the below steps to run Test using Local System:
Step 1: Download or Clone the TestNG HyperExecute GitHub repository for the code samples to run tests on the HyperExecute (skip this step if you are performing tests on your own project).
Step 2: Download the HyperExecute CLI as per your host Operating System. The CLI must be in the root directory of the project.
Step 3: Configure your YAML file as per your use cases using key value pairs.
version: 0.2
globalTimeout: 150
testSuiteTimeout: 150
testSuiteStep: 150
runson: win
autosplit: true
retryOnFailure: true
maxRetries: 1
concurrency: 5
pre:
# Skip execution of the tests in the pre step
- mvn dependency:resolve
post:
- ls target/surefire-reports/
mergeArtifacts: true
uploadArtefacts:
- name: ExecutionSnapshots
path:
- target/surefire-reports/html/**
framework:
name: maven/testng
defaultReports: false
flags:
- "-Dplatname=win"
Step 4: Run the below command in your terminal at the root folder of the project:
./hyperexecute --config yaml/win/testng_hyperexecute_autosplit_sample.yaml --download-artifacts --force-clean-artifacts
Shown below is the execution screenshot when the YAML file is triggered from the terminal:


Step 5: Monitor the test execution status on the HyperExecute Dashboard.

HyperExecute also facilitates the provision to download the artifacts on your local machine. To download the artifacts, click on Artifacts button corresponding to the associated TestID.

2. Testing Using Gitpod
Follow the below steps to run Test using Gitpod:
Step 1: Click 'Open in Gitpod' button. You will be redirected to Login/Signup page.
Step 2: Login with Lambdatest credentials. Once logged in, a pop-up confirmation will appear, asking you to 'Proceed' to the Gitpod editor in a new tab. The current tab will display the hyperexecute dashboard.
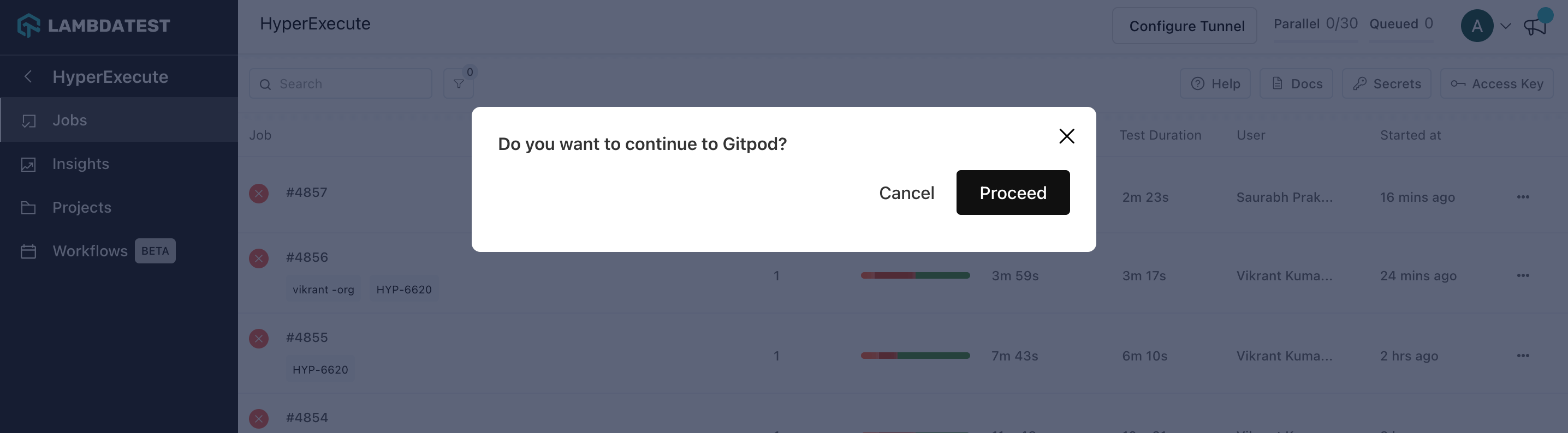
Step 3: Choose your preferred editor (we recommend VS Code Editor)
Step 4: As you are running a sample project, Fetching of the Test Scripts, HyperExecute YAML, HyperExecute CLI and Triggering your tests using the Execution Command
will be automated.
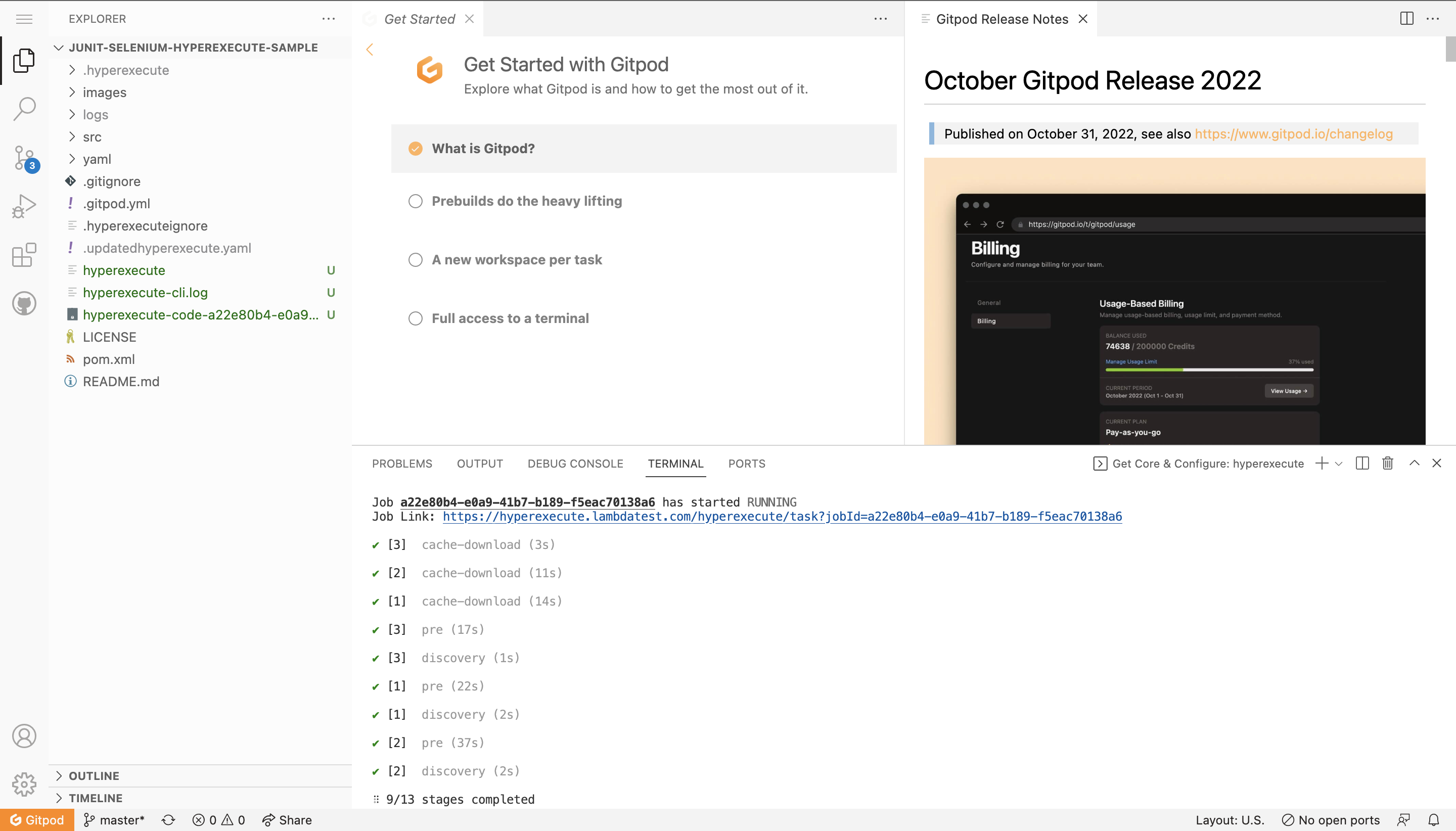
Step 5: Once you see the Job Link
in the logs, you can visit the HyperExecute dashboard to see the tests getting executed.
You can also implement Secret Keys in your YAML file.
Sample Yaml Version 0.2
---
version: 0.2
globalTimeout: 150
testSuiteTimeout: 150
testSuiteStep: 150
runson: win
autosplit: true
retryOnFailure: true
maxRetries: 1
concurrency: 5
pre:
# Skip execution of the tests in the pre step
- mvn dependency:resolve
post:
- ls target/surefire-reports/
mergeArtifacts: true
uploadArtefacts:
- name: ExecutionSnapshots
path:
- target/surefire-reports/html/**
framework:
name: maven/testng
defaultReports: false
flags:
- "-Dplatname=win"
Navigation in Automation Dashboard
Every test run on the HyperExecute Grid has a unique jobId associated with it. Each jobId can in turn constitute single (or multiple) groupId(s). You can visit HyperExecute automation dashboard for checking the status of the test execution.
HyperExecute lets you seamlessly navigate between jobId's and taskId's. It can be done by navigating to Automation -> HyperExecute logs -> Corresponding jobId on the HyperExecute automation dashboard.
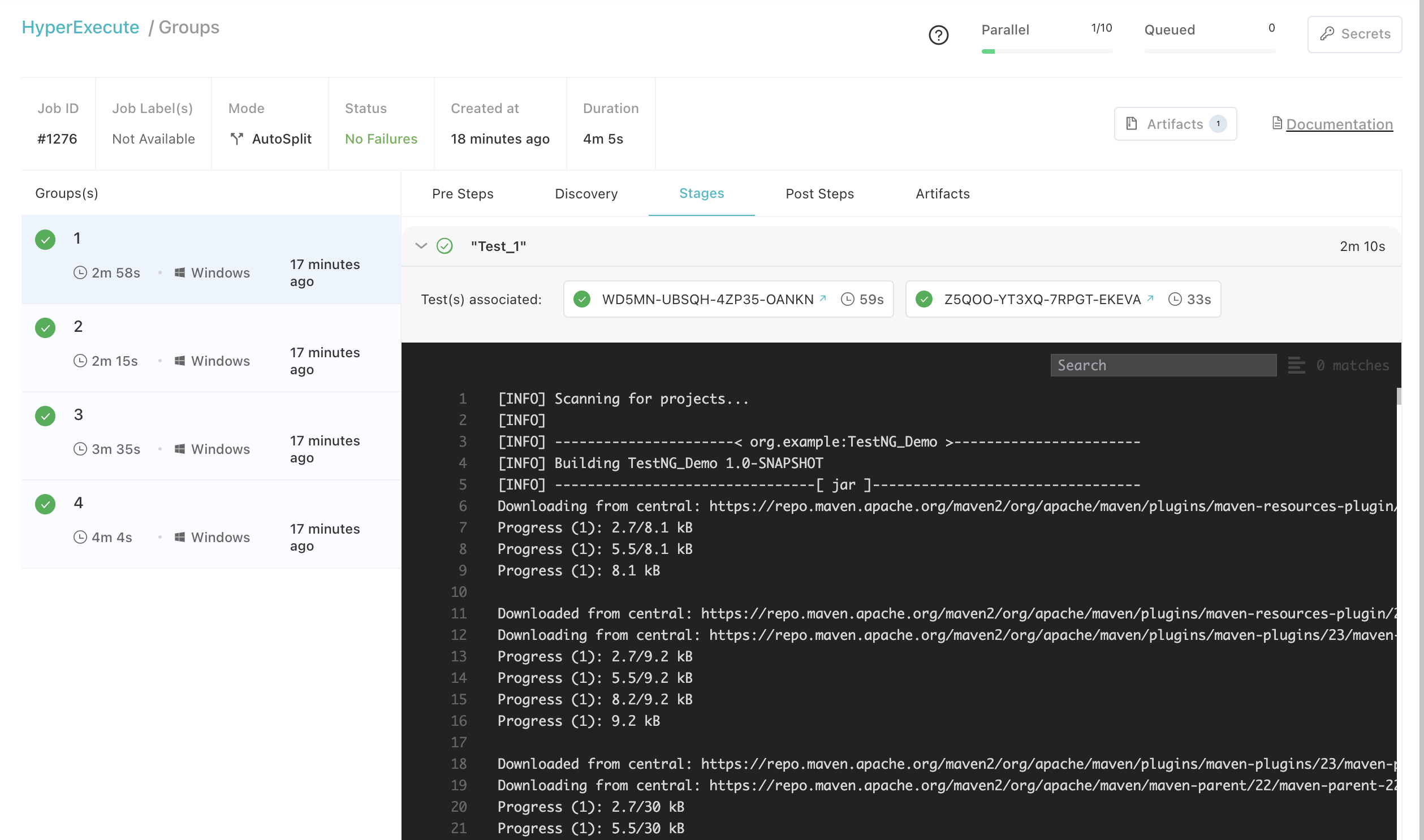
The snapshot below shows how to navigate to the respective testID for viewing the Selenium logs:

Conclusion
By following the instructions in this documentation, you can seamlessly execute the TestNG tests on HyperExecute, leveraging its secure cloud infrastructure, advanced features, and optimized test execution workflow.
For any query or doubt, please feel free to contact us via 24×7 chat support or you can also drop a mail to support@lambdatest.com.
Happy testing!