- Test that the components render without errors: In this Angular test case check that an Angular component renders correctly, you can use a basic test called a smoke test. This test verifies that the component can be created without generating errors. It's a mini and rapid regression test for major functionality.
- Test that the components render with props: Testing whether a component is rendered within Angular involves writing unit tests that verify that the component displays the information given by the input property. To test a component rendered with props in Angular, you can use Angular's built-in test tool and follow these steps:
Import the necessary modules and components: In the test file, import the module containing the component you want to test, and any other required modules or components.
- Create test component: Create a test component with the component to be tested as the underlying component. You can pass the necessary props to child components in the test component template.
- Build Test Components: Use TestBed to create test components.
- Access to subcomponents: Use the fixture object to access subcomponent instances.
- Verify component behaviour: Use Jasmine's assertions to verify that child components render as expected with the given props.
- Test that the components render with the state:In this section, we will look at the angular test case where that the component renders with the state. It involves writing a unit test that verifies that the component correctly displays data derived from its internal state or state management system.
- Test that the component updates state when an event is triggered:When it comes to checking the component update state when an event is triggered we can combine the following techniques to test we can set up the component and its dependencies to Create an instance of the component and any necessary dependencies such as services and other components in your test file.
Trigger the event to call the method or simulate the event that triggers the state change in the component. Maintain the state changes to Check the component's state to ensure that it has been updated as expected.
- Test that the component renders with conditional logic:In this section, we will see how rendering components, a component may have a conditional logic that determines which parts of the model are rendered depending on certain conditions or input values. Testing that a component renders with conditional logic means checking that the expected elements are rendered according to the values of the conditions.
For example, a component can have a boolean input property that determines whether a certain element should be displayed in the model or not. In this case, the component test must verify that the element is displayed when the input is true and not when the input is false.
Similarly, a component might have a conditional statement in the template that renders different elements based on a condition. This Angular test case for the component should verify that the correct element is rendered based on the value of the condition. Testing a component's rendering with conditional logic ensures that the component behaves correctly and consistently in a variety of scenarios and can catch potential bugs or regression.
- Test that the component calls a function when a button is clicked: In Angular, to check if a component calls a function when a button is clicked, you need to write a Angular test case that checks if a specific method or function of the component is called when the button is clicked. These types of tests are important to ensure that components work properly and respond correctly to user interactions. To test this functionality, I usually create a dummy call detection function and check that function to see if it gets called when the button is clicked.
Then simulate a button click event and verify that the function is called as expected. In Angular, you can use the Angular Testing Framework to create and run unit tests for your components.
The framework provides utilities for instantiating and manipulating components, selecting elements from component templates, and simulating user interactions (such as buttons). These utilities make it easy to write Angular test cases to verify that your components are working properly.
- Test that the component renders a list of items: To verify that a component renders a list of elements, you need to write a Angular test case that verifies that the component's template renders the correct elements in the correct order. These types of tests are important to ensure that the component renders the data it receives correctly and that the layout and style of the list items match our expectations. To test this functionality, you typically create a test fixture and a bean instance, then set the bean's input properties to simulate the data. You can then use the Angular Testing Framework debug utility to inspect the component's template and verify that the list items are rendering correctly.
- Test that the component updates the list when a new item is added: To verify that the component updates the list when a new item is added, we need to write a Angular test case to verify that the component behaves correctly when a new item is added to the list. To test this functionality, you typically instantiate a bean and provide a dummy input containing the original list of elements. Then update the component model by simulating the addition of a new item to the list and enabling change detection. Asserts that the list of last seen items now contains the newly added items.
- Test that the component removes an item from the list when a delete button is clicked: In this section, we'll look at how components remove items from the list when a delete button is clicked. To do this, you must first render the component that includes the list and the delete button. Then, you need to make a list of items and pass it as a prop to the component. Create a click event on the delete button of one of the list items, then declare that the item has been eliminated.
- Test that the component sorts a list of items in ascending order: The goal of testing a component that sorts a list of items in ascending order is to ensure that it sorts the given list correctly and in the expected order. We can ensure that the sorting functionality is functioning correctly and that it is accurate by testing it.
Testing the component can help identify any potential issues with the sorting algorithm or any flaws in the implementation that may affect the output. We can catch any issues early on and prevent them from causing problems in production. Furthermore, testing the sorting component can help ensure that it meets any performance requirements, such as efficiently sorting large lists or gracefully handling edge cases.
- Test that the component sorts a list of items in descending order: The goal of testing a component that sorts a list of items in descending order is to ensure that it sorts the given list correctly and in descending order. We can ensure that the sorting functionality is functioning correctly and that it is accurate by testing it.
- Test that the component filters a list of items based on a search term: Testing the component that filters a list of items based on a search term involves creating Angular test cases to ensure that the element correctly filters a given list of items based on the search term provided by the user.
The component typically receives the list of items and the search term as inputs and then uses these inputs to filter the list and display the filtered items to the user. To test this component, we need to create a mock list of items, set the search term, and then check that the component displays the correct filtered items. We should also add test edge cases, such as when the search term is longer than any item name or when the list of items is empty.
- Test that the component renders with nested components: When testing rendered components with nested components in Angular, there are a few things to keep in mind. These are some options for action.
- Setting up the test environment: You must first prepare the test environment before you can test your component. This requires setting up a TestBed setup with your component and any dependencies, as well as importing the required Angular test modules
- Mock the nested components: If your component has nested components, you should mimic them to separate the functionality of your component. To achieve this, you can make a stub component that copies the real nested component's behaviour.
- The TestBed.overrideComponent() method can then be used to replace the real nested component with the stub component and the component's behaviour should be tested after the testing environment has been set up and the nested components have been mocked.
- The component's input, output and any events it emits should all be tested.
- Testing the nested components is important if you mocked the behaviour of the nested components. This can be accomplished by either writing individual tests for each nested component or including them in the tests for the parent component.
- Utilize the Angular testing tools: Angular offers several testing tools that might make it easier for you to test your components. To detect changes and update the component's view, for instance, use the ComponentFixture.detectChanges() method. To access the component's DOM element and test its properties and behaviour, you can also use the ComponentFixture.nativeElement property.
- Test that the component renders with children's components: To test whether an Angular component renders with its child components, one must confirm that the HTML elements of the child components are present in the parent component's template. In another word, when the parent component is rendered, it's also necessary that the child components should also be rendered within the parent component's view.
For instance, when the parent component is rendered, the button should also be displayed if a child component of the parent component displays a button. you can use the unit tests that utilize the TestBed and ComponentFixture utilities to test that a component renders with children components, these tests create instances of the parent component and confirmed that the child components are correctly displayed in the parent component's view.
- Test that the component passes props down to its children's components: In this section, we will cover the Angular test case which is related to the components that are correctly passing props down to its children components which involves verifying that the data passed as input properties by the parent component is correctly received by the child component. This type of test usually involves creating an instance of the parent component, setting the input property values that should be passed down to the child component, and then checking that the child component has received the expected values. Testing input properties can be done in isolation or within a larger integration test that tests multiple components working together.
In general, testing input properties is important to ensure that data is flowing correctly between components and that the application behaves as expected.
- Test that the component passes functions down to its children's components: In this Angular test case, a parent component can pass down functions to its child components as inputs. To ensure that the parent component is correctly passing the function down to its child components, we can write a test that checks if the function is being called by the child component.
The test involves creating a parent component that has a function and a child component that accepts a function as an input. The function in the parent component is passed down to the child component through the input binding. The test then simulates a call to the function in the child component and checks if the function in the parent component was called.
To write the test, we use the Angular testing utilities such as ComponentFixture, TestBed, and By to create the parent and child components and to access their elements in the DOM. We can then use the spyOn() function to create a spy for the function in the parent component. The spy allows us to track whether the function was called or not.
This Angular test case involves the following steps:
- Create the parent component with a function that will be passed down to its child components.
- Create the child component that accepts the function as an input.
- In the test, create a spy for the function in the parent component.
- Get the child component instance using fixture.debugElement.query(By.directive(ChildComponent)).componentInstance.
- Call the function in the child component.
- Assert that the function in the parent component was called using expect(component.functionName).toHaveBeenCalled().
- If the test passes, it means that the function was successfully passed down to the child component and called when triggered by the child component. This ensures that the parent component is correctly passing functions down to its child components.
- Test that the component renders with styles: In this Angular test case, we will be testing that a component renders with styles in angular which involve verifying that the styles defined in the component's style are correctly applied when the component is rendered. This ensures that the component appears as intended and that the visual design is consistent with the design specification.
You may use a combination of Angular's testing tools and assertions to make sure the desired styles are present in the component's generated output when checking that it renders with styles.
By testing that a component renders with styles, you can catch issues such as missing or incorrect styles early on in the development process and ensure that your UI is consistent and visually appealing.
- Test that the component renders with classes: This is a very simple explanation to test that the component renders with classes, suppose that you have a component called MyComponent that has a div element with a class of my-class. You want to write a Angular test case to ensure that this class is present when the component is rendered. By using this test case you can ensure the correct styling, accessibility and maintainability.
- Test that the component renders with dynamic classes: Test the rendering of an Angular component with dynamic classes involves designed to ensure that dynamically generated CSS classes in the component's logic or templates are correctly applied when rendering the component. This ensures that components look as expected and are styled according to design specifications.
To test if a component is rendered with dynamic classes, you can use Angular's test harness with assertions to verify that the expected classes are present in the component's rendered output. Some common techniques for testing dynamic component classes in Angular include:
- To create a testing environment and access the rendered output of the component, use the TestBed and ComponentFixture.
- Setting the properties or inputs of the component that affect the dynamic classes.
- Using the component's fixture. To detect changes and update the rendered output of the component, use the detect changes () method.
- Using Angular's testing utilities, such as the By directive or the native element property of the ComponentFixture, you can query the rendered output for specific elements or classes or assertions to ensure that the expected dynamic classes are present in the component's rendered output, such as by using the fixture to check for specific CSS classes.
- Test that the component renders with dynamic styles : In this section, we will be Testing that a component with dynamic styles renders in Angular involves verifying that dynamically generated CSS styles in the component's logic or templates are applied correctly when rendering the component this ensures that components look as expected and are styled according to design specifications.You can use Angular's testing tools with assertions to verify that the expected styles are present in the component's rendered output to see if it is rendered with dynamic styles. By testing that a component renders with dynamic styles, you can ensure that the component responds correctly to changes in its inputs or properties and that the UI remains consistent and visually appealing.
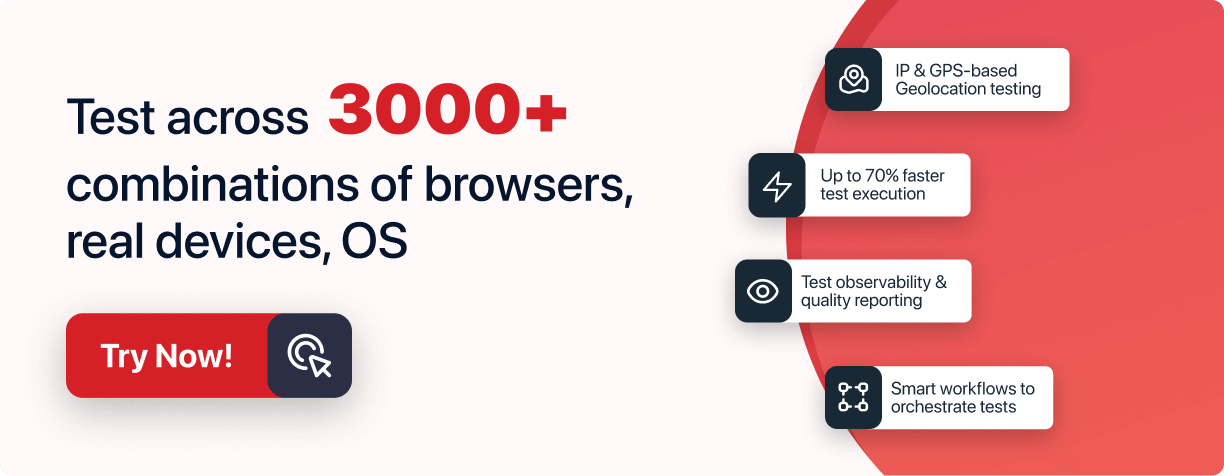
- Test that the component renders with conditional styles: In this particular Angular test case, testing whether a component renders with conditional styles includes verifying that the CSS styles that are conditionally applied based on the component's state or inputs are applied correctly when the component is rendered. This ensures that the component looks as intended and that the styles adhere to the design specifications.
To test that a component renders with conditional styles, you can use a combination of Angular's testing utilities and assertions to verify that the expected styles are present in the component's rendered output.
These are some common techniques for testing conditional component styles:
- Using the TestBed and ComponentFixture to create a testing (surrounding conditions) and access the component's given output.
- Setting the component's properties or inputs that affect the conditional styles.
- Using the component's fixture changes () method to trigger change detection and update the component's rendered output.
- Querying the rendered output for specific elements or inline styles using Angular's testing utilities, such as the By directive or the nativeElement property of the ComponentFixture.
- Using assertions to verify that the expected conditional styles are present in the component's rendered output, such as by checking for specific inline styles using the fixture.debugElement.query() method and the element's nativeElement.style property.
- By testing that a component renders with conditional styles, you can ensure that the component responds correctly to changes in its inputs or properties and that the UI remains consistent and visually appealing
- Test that the component renders with animations: In this section, we will see the Angular test cases that are related to the component renders with animations. This includes verifying that the animations that are defined in the component's logic or template are properly applied when the component is rendered. This ensures that the component behaves as intended and that the animations are consistent with the design specification.
To test that a component renders with animations, you can use a combination of Angular's testing utilities and assertions to verify that the expected animations are present in the component's rendered output. you can ensure that the component behaves as intended and that the UI is visually appealing. It also helps to verify that the animations are triggered correctly in response to changes in the components.
- Test that the component renders with dynamic animations: In this section, we will see the Angular test case related to a component that renders with dynamic animation which involves creating unit tests to ensure that the animations work properly and don't introduce any unexpected behaviour or bugs, to test these components you can use Angular Testing Utilities to create a test bed for your component and its associated services and dependencies. These are some basic steps to follow
- You have to Import the necessary modules and components for your test bed, including any animation-related modules or services.
- Create a test bed using TestBed.configureTestingModule() method, passing in any necessary configuration options, including declarations, providers, and imports.
- Use the compileComponents() method to compile your component and its associated templates.
- Create a fixture for your component using the TestBed.createComponent() method.
- Use the detectChanges() method to trigger change detection for your component, which will cause any animations to be initialized and executed.
- You can use the By utility to locate specific elements in the DOM, and the triggerEventHandler() method to simulate user interactions or other events that would trigger animations.
- Test that the component renders with conditional animations: In Angular, conditional animations are used to apply animations based on specific conditions, such as when a component is added or removed from the DOM, or when a property changes its value. To test a component that renders with conditional animations, you can follow these steps:
- Write the test case: Start by writing a test case for the component that contains the conditional animations. This Angular test case should cover all the possible scenarios that trigger the animations, such as adding or removing the component from the DOM, changing the value of a property, etc.
- Create a test component: To test the component with animations, you will need to create a test component that contains the component being tested as a child. This allows you to manipulate the test component's state and properties to trigger the animations.
- Set up the test bed: Use Angular TestBed to create an instance of the test component and compile its template.
- Trigger the animations: Once the test component is set up, you can manipulate its state and properties to trigger the animations. For example, you could add or remove the component from the DOM, or change the value of a property that controls the animations.
- Assert the expected results: Finally, you should assert that the expected animations were triggered and that the component is rendering correctly. You can use the Angular Testing Utilities, such as expect() and toMatchInlineSnapshot(), to make assertions about the component's behaviour and appearance.
Overall, testing an Angular component that renders with conditional animations generally requires careful consideration of all possible scenarios that can trigger the animations, as well as the component's expected behaviour and appearance.
- Test that the component renders with transitions: In this Angular test case we will be discussing the components that render with animation, transitions are used to animate changes in the state of a component, such as when a property changes its value or when a component is added or removed from the DOM. To test a component that renders with transitions, you can follow these steps:
- Write a test case: First write a test case for the component that contains the transition. This test case should cover all scenarios that can cause transitions, such as changing the value of a property, adding or removing a component in the DOM, etc.
- Creating a test component: To use a transformation test component, you must create a test component that contains the components to be tested as subcomponents. It allows you to manipulate the state and properties of test components to trigger transitions.
- Configure the testbed: Use Angular TestBed to create an instance of the test component and compile its model.
- Enable Transitions: After configuring a test component, you can manipulate its state and properties to enable transitions. For example, you can change the value of a property that controls transitions, or add or remove DOM components. Finally, you must affirm that the expected transitions are enabled and that the component renders correctly.
Overall, testing a component rendered using transitions in Angular requires careful consideration of all scenarios that might trigger transitions, as well as the expected behaviour and appearance of the component.
- Test that the component renders with dynamic transitions: In this Angular test case, we will discuss how dynamic transitions are used to apply transitions based on dynamic changes in the state of a component, such as changes to the data being displayed. By using this you can ensure that your tests accurately reflect the behaviour of the component and catch any issues or regressions that may arise.
- Test that the component renders with conditional transitions: In this Angular test case, we will discuss conditional transitions that are used to apply transitions based on certain conditions, such as when a component is added or removed from the DOM, or when a property changes its value. you can follow these steps to test :
- Create a test component: To test the component with conditional transitions, you will need to create a test component that contains the component being tested as a child. This allows you to manipulate the test component's state and properties to trigger the conditions that will trigger the transitions.
- Set up the test bed: Use Angular TestBed to create an instance of the test component and compile its template.
- Trigger the conditions: Once the test component is set up, you can manipulate its state and properties to trigger the conditions that will trigger the transitions. For example, you could add or remove the component from the DOM, or change the value of a property that controls the transitions.
- Assert the expected results: Finally, you should assert that the expected transitions were triggered and that the component is rendering correctly based on the conditions. You can use the Angular Testing Utilities, such as expect() and toMatchInlineSnapshot(), to make assertions about the component's behaviour and appearance.
These methods will help you make sure that your tests appropriately reflect the behaviour of the component and catch any issue or problem that arises.
- Test that the component renders with images: Images are a critical component of many UIs, and if they are not displayed correctly, it can negatively impact the user experience, So we will create a Angular test case where the component renders with images in Angular which ensures that the images are being displayed correctly.
For example, an e-commerce site that doesn't display product images correctly will not be able to effectively sell products.
Some images are loaded from external systems, such as image hosting services or content delivery networks. By testing that the component can correctly display images from these sources, we can ensure that our application integrates correctly with these systems.
- Test that the component renders with dynamic images: To test whether an Angular component renders with dynamic images, one must ensure that the component correctly renders a set of photos that are supplied dynamically as input. This test checks that the component is correctly rendering and displaying the images as well as handling any changes to the input data.
- Test that the component renders with conditional images: In Angular, when we want to conditionally render images based on certain conditions, we can use a *ngIf directive to achieve this. Here's an example of how this can be done:
Let's say we have a component that displays an image based on a boolean value. If the value is true, we want to display one image, and if it's false, we want to display a different image.
- Test that the component renders with video: In this section of Angular test case, we will see that a component renders with a video which helps to ensure that components are functioning correctly and meets the requirements of the application. A video is a complex media element that requires correct configuration and rendering to function as expected, a component may be responsible for rendering a video element, handling user interactions, and providing other functionality.
By testing that the component correctly renders a video, we can catch potential issues such as incorrect video URLs, missing or incorrect source elements, or other issues that may prevent the video from being displayed or functioning correctly. It also ensures that any changes made to the component, such as adding new functionality or modifying existing functionality, do not break existing functionality related to a video.
- Test that the component renders with dynamic video: Testing that a component renders with dynamic video in Angular ensures that the component is functioning as expected and that any video-related features are working correctly. This is important for maintaining the quality and reliability of the application, as well as ensuring a positive user experience. This Angular test case can be achieved by creating a test file, importing necessary modules, creating a component instance, and then testing that the video element is correctly rendered with the dynamic source.
This can be done by using the Angular testing utilities to access the component's DOM and check the properties of the video element. The test should ensure that the correct video source URL is set, that the video is playing, and that the correct video controls are displayed.
- Test that the component renders with conditional video: In this section, we will discuss the component render with a conditional video which makes sure that the video element with a dynamic source is properly rendered. To develop this test, we would generally utilize the Angular testing facilities to generate a test suite and a test case. We would import the required modules and components into the test case, create an instance of the test component, and then use the Angular testing utilities to access the component's DOM elements.
Coming to dynamic video rendering, we would ensure that the video element is correctly rendered with the dynamic source by checking its properties. This might involve testing that the correct source URL is set on the video element, that the video is playing, and that the appropriate video controls are displayed.
- Test that the component renders with audio.: The purpose of this Angular test case is to ensure that a component that contains an audio element is rendered properly in the Angular application. The test case verifies that the audio element exists in the component and that it has the correct source file or URL. Optionally, the test case can also check that the audio is playing or paused, depending on the expected behavior.
Here's a step-by-step explanation of how this Angular test case works:
- First, the necessary modules and components for testing are imported into the test file.
- Then, a test bed is created for the component using the TestBed API from Angular.
- The necessary services or components for the test are injected using the TestBed API.
- A fixture is created for the component using the ComponentFixture API from Angular.
- The ngOnInit() method of the component is triggered if it exists.
- The view is updated using the fixture's detectChanges() method.
- The audio element in the component's template is found using a CSS selector or other method.
By performing these steps, the test case ensures that the component is rendered properly with the audio element and that it behaves as expected.
- Test that the component renders with dynamic audio: This Angular test case ensures that a component that renders dynamic audio, i.e., audio that is loaded from a source that can change at runtime, is working as expected in the Angular application. The test case verifies that the audio element in the component's template is updated with the correct source file or URL when the source changes. To perform this test you can follow the same steps that mentioned in the above test case (Test that the component renders with audio).
- Test that the component renders with conditional audio: The purpose of this Angular test case is to verify that a component that conditionally renders an audio element based on certain conditions works as expected in an Angular app. Test cases conditionally check the presence of an audio element and the existence of a valid source file or URL (if any).
- Test that the component renders with iframes: An Angular test case called "Test that the component renders with iframes in Angular" is intended to confirm that a component that contains an iframe element is rendered successfully in the framework. The test case confirms that the iframe element is present in the component's template and has the correct source file or URL. (In Angular, an iframe is an HTML element that is used to embed another HTML document into the current document. It is often used to display content from another website or application within an Angular component).
Here are the basic steps involved in this Angular test case:
- Create a test bed and import any required modules and dependencies for testing the component.
- Instantiate the component using the ComponentFixture API from Angular.
- Trigger any necessary lifecycle hooks, such as ngOnInit(), that will initialize the component and render the iframe element.
- Call the detectChanges() method on the fixture to ensure that the view has been updated.
- Use a CSS selector or other method to locate the iframe element in the component's HTML template.
- Verify that the iframe element is present and that it is rendering the expected content or source file.
- Test any additional functionality related to the iframe element, such as verifying that it is loading or has finished loading.
By performing these steps, the Angular test case can ensure that the component is properly rendering the iframe element and that it can be integrated with other parts of the application that rely on the iframe functionality. This helps to ensure that the component is working as expected and that any issues with the iframe element are identified and addressed.
- Test that the component renders with dynamic iframes: This Angular test case is designed to ensure that an Angular component that generates and renders iframes dynamically is working as expected. It is typically used when the component needs to render with different iframe sources or content based on user input or other dynamic factors. This ensures that the component dynamically creates and renders iframes, integrates correctly with external sources, performs optimally, and is compatible with a variety of dynamic inputs or elements.
This can help you identify and fix the issues with dynamic elements on iframe before they affect the component's full functionality. To perform this Angular test case you need to follow the same steps that mentioned in the above test case (37).
- Test that the component renders with conditional iframes: Checking if a component conditionally renders iframes in an Angular test case is designed to verify that Angular components that conditionally create and render iframes work as expected. Conditional rendering of iframes may occur when we want to render an iframe only when certain conditions are met, such as when the user clicks a button or when certain data is available. Testing the component with conditional iframes can help to ensure that the iframe elements are generated and rendered properly, and only when necessary.
- Test that the component renders with forms: An Angular component that uses forms will need to pass the "Test that the component renders with forms in Angular" test case in order to work as expected. When a component has input fields or other form components that the user may interact with, this test case is often performed. It is important to ensure that the forms in the component are working correctly and providing the desired functionality to the end user. By testing that the component renders with forms, we can identify any issues or bugs in the component's form functionality before it is deployed to production.
- Test that the component renders with dynamic forms: The objective of the test is mainly to find out whether the Angular component can render dynamic forms, which are forms that may be built or modified dynamically depending on user input or other factors. The test may involve checking that the component correctly renders the form fields and handles user input, as well as verifying that the component can handle changes to the form's structure or data. The overall goal of the test is probably to make sure that the Angular component is working correctly and providing the expected user experience when it comes to dynamic forms.
- Test that the component renders with conditional forms: In Angular, conditional form testing is used to check that a component renders successfully and that users can interact with it as expected. By testing the rendering of the angular component, we can verify the necessary input fields, buttons, and other elements are displayed or hidden based on the conditions set in the form. Here, you can also check to see that the component responds to user input successfully and that any validation or error messages are presented correctly.
- Test that the component renders with validation: The goal of testing an Angular component renders with validation is to ensure that the form input data is properly validated and that the relevant error messages are given to the user when incorrect data is supplied. When form data is validated, we make sure it satisfies specified requirements like data type, length, or format. We can also specify unique validation rules. By validating input data, we can improve user experience, maintain consistency and correctness of data, and prevent user errors.
By validating the component's rendering, we can ensure that the validation rules are correctly applied and that the appropriate error messages are displayed when the user enters invalid data. We can also test that the form only submits when the input data is correct. It also helps us ensure that user input data is correctly validated and that the user experience is smooth and error-free.
- Test that the component renders with dynamic validation: The goal of testing that an Angular component renders with dynamic validation is to ensure that the validation rules are correctly applied to dynamic form fields. Dynamic validation means that the validation rules are determined based on user input or other dynamic factors such as data type, user role, or system configuration. We can customise the validation rules based on specific requirements, making the user experience more flexible and intuitive. We can also verify that the validation rules are applied correctly to dynamic form fields, and the correct error messages are displayed when the user enters invalid data.
It also assists us in making sure that user input data is validated correctly based on the specific requirements and that the user experience is customized to their requirements.
- Test that the component renders with conditional validation: This Angular test case ensures that the validation rules are applied correctly based on the conditions specified in the form. The validation rules are determined by specific conditions or criteria, such as the user's input or the state of other form fields, in conditional validation. Conditional validation enables us to apply different validation rules based on the user's input, resulting in a more flexible and intuitive user experience. We can test that the validation rules are updated dynamically when the user interacts with the form and the conditions change.
- Test that the component renders with server-side rendering: The purpose of testing component rendering with Server Side Rendering (SSR) in Angular is to ensure that the component can be rendered correctly both server-side and client-side, and the user experience is consistent across different devices and environments. (Server-side rendering means that the Angular app is rendered on the server and the server sends fully rendered HTML to the client browser instead of sending the app's JavaScript bundle. SSR improves application performance, SEO, and accessibility, ensuring content is visible to users with slow or unstable network connections).
By testing the component's rendering with SSR, we can ensure that the component's template and styles are rendered correctly on the server and that the component can be rendered correctly on the client side. We can also ensure that the user experience is consistent across devices and environments and that the application performs well under different network conditions.
It also assists us in ensuring that the user experience remains consistent regardless of the client's environment and that the application is optimized for performance and accessibility.
- Test that the component renders with dynamic server-side rendering: To verify that dynamic content is rendered successfully on the server and client side and that the user experience is consistent across various devices and contexts, it is important to test that a component renders with dynamic server-side rendering (SSR) in Angular. Dynamic server-side rendering means that the Angular application is rendered on the server, and the server sends the fully rendered HTML, including any dynamic content, to the client's browser. Data retrieved from an external API or changes to the UI based on user interactions are examples of dynamic content.
- Test that the component renders with accessibility features: The goal of testing how an Angular component renders with accessibility features is to ensure that the component is accessible to users with disabilities or impairments and meets accessibility guidelines and standards. Accessibility features include keyboard navigation, screen reader compatibility, colour contrast, and ARIA attributes, among others. We can verify that accessibility features are implemented correctly and that the component is usable and understandable for users with disabilities or impairments by testing it with accessibility features.
Testing the component with accessibility features can assist us in identifying any issues or barriers that may prevent disabled users from accessing or using the component. It also assists us in ensuring that the component complies with accessibility standards and guidelines, such as the Web Content Accessibility Guidelines (WCAG), and provides a friendly user experience for all users. Improving the accessibility of the application not only helps users with disabilities but also benefits all users by making the application more user-friendly, easier to navigate, and improving overall user experience.
- Test that the component renders with localization: Testing that the component renders with localization in Angular is the process of ensuring that the Angular component shows localized content in the user's desired language (the term "localization" describes the process of modifying an application to accommodate various languages, cultures, and geographical areas). This testing procedure involves making sure that the component shows the correct localized text for each supported language, as well as any other parts of the component's behaviour that may be affected by the localization, such as date and time formats, number formats, and currency symbols.
- Test that the component renders with dynamic localization: When testing whether a component with dynamic localization is rendered in Angular, we verify that the component displays the correct localized content based on the user's dynamically selected language setting. Dynamic localization means that the user can change the language preference of the application while the application is running, and the component should be updated to display the content in the newly selected language.
- Test that the component renders with conditional localization: In Angular, conditional localization refers to the process of presenting alternative localized content based on the user's language preferences. This is commonly achieved in Angular using the ngx-translate module. It is used to design an app that supports many languages and gives a personalized experience for each user. For example, depending on the user's language, you may display different text, graphics, or even entire sections of the program.
To perform conditional localization in Angular, separate translation files are typically created for each supported language. Then, based on the user's language option, you can show the relevant content using Angular's built-in directives or ngx-translate methods.
- Test that the component renders with conditional dynamic localization: When testing a component with conditional dynamic localization, you should verify that the component's output changes appropriately based on the user's language preference or location. You should also ensure that the component's localization logic works correctly for all possible input values.
To achieve this, you can use Angular's built-in testing framework, which provides various testing utilities and methods to simulate user interactions and verify the behaviour of your components. You can write unit tests that use mock data to test the component's localization logic or integration tests that verify the behaviour of the component in a real-world scenario.
- Test that the component renders with error handling: The goal of testing an error-handling component in Angular is to guarantee that the component can handle problems that may occur during its execution. Error management is a critical component of every application since it helps the application recover from errors gracefully and deliver a better user experience.
We can confirm that the application acts as expected when things go wrong by testing the component's error-handling capabilities. This involves ensuring that the correct error message is given to the user, that the application does not crash or behave unexpectedly when an error occurs, and that the appropriate recovery procedures are taken.
Testing error handling also assists us in identifying and resolving issues before they reach production.
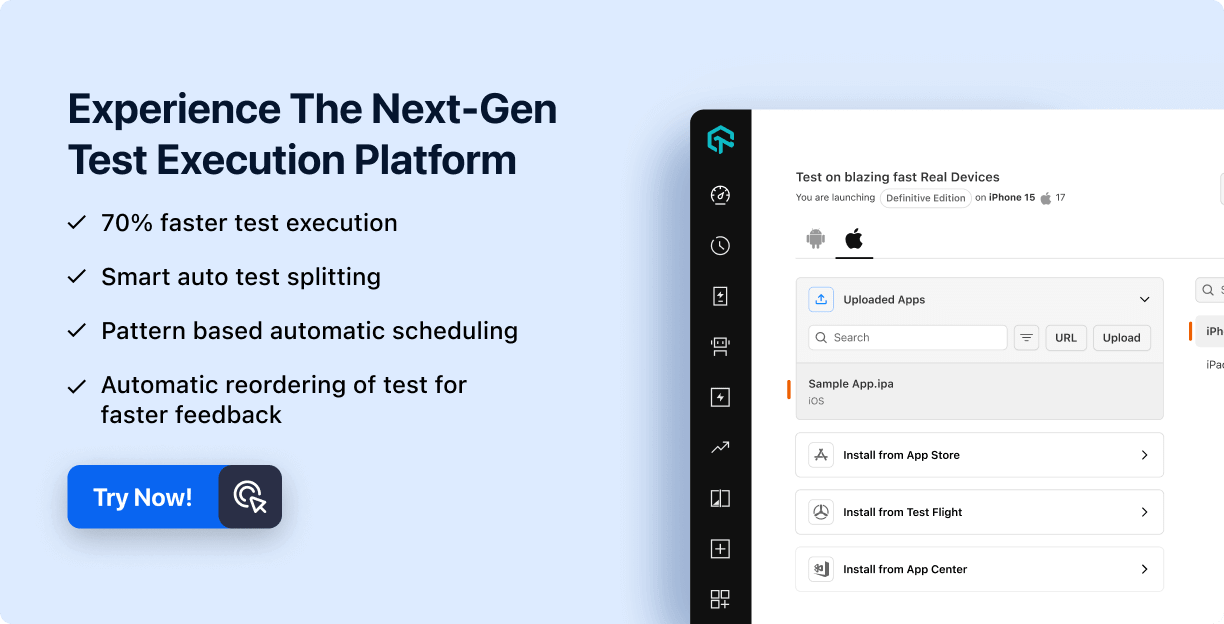
- Test that the component renders with dynamic error handling: The goal of testing an Angular component with dynamic error handling is to guarantee that it can handle a variety of problems that may occur during its operation dynamically and flexibly. Dynamic Error handling refers to the component's capacity to respond to various types of errors and give relevant error messages and actions based on the error observed.
By validating the component's dynamic error-handling capabilities, we can ensure that the application provides a consistent and user-friendly error-handling experience. This includes ensuring that the component can handle various error types, displays the proper error message for each issue type, and offers the user suitable actions or ideas for resolving the error.
- Test that the component renders with conditional error handling: When working with angular it's usual to face errors, such as failed HTTP requests or incorrect user input. To handle these errors, components often display error messages to the user or take other actions to recover from the error. By testing that a component renders with conditional error handling, you can verify that the component behaves correctly in the event of an error.
It plays an important role in building high-quality Angular applications that are reliable and user-friendly.
- Test that the component handles input binding: The goal of Angular input binding testing is to ensure that the component successfully receives input data from its parent component and uses that data to produce its output. This mechanism allows a parent component to provide data as an input property to a child component. This part of the component is tested to ensure that it is handling the input data correctly and responding appropriately to changes in the input data.
Input binding testing often entails building a mock parent component, passing in test input data, and then validating that the component renders the output correctly based on the input data. This helps to detect any problems with the component's handling of incoming data and ensures that the component is functioning properly.
Overall, testing input binding is a crucial part of assuring the validity and robustness of an Angular component, and it can aid in the prevention of mistakes and difficulties that may develop as a result of incorrect input data handling.
- Test that the component handles output binding: This Angular test case confirms that the component is successfully generating output events to its parent component and that the parent component can handle these events appropriately. It allows a child component to use an output property to transmit events to its parent component. This feature of the component is tested to ensure that the component emits events effectively and that the parent component can handle these events and respond properly.
To test output binding, one typically builds a fake parent component, subscribes to the output event sent by the child component, and then uses test data to trigger the event in the child component. This makes it easier to identify any problems with the component's event emission and guarantees that the parent component can appropriately react to the events that the child component emits.
- Test that the component renders with dependency injection: In this section, we will learn that the testing of the component that renders with dependency injection ensures that the component correctly uses the dependencies injected into it via its constructor. (Dependency injection is an Angular design pattern in which the Angular injector provides a component's dependencies. This enables better modularization and separation of concerns, making components easier to write and test).
To test that a component renders with dependency injection, we can use Angular's testing utilities to create a test bed and configure it with the necessary dependencies for the component. To accomplish this, mock dependencies must first be created using the jasmine.createSpyObj method, and then supplied to the test environment using the provider's property.
Once the dependencies are supplied, we can create an instance of the component and verify that it uses the injected dependencies correctly. This can include ensuring that the component's methods and properties behave as expected, based on the functionality provided by the injected dependencies.
- Test that the component handles multiple dependencies: In Angular, testing how a component handles multiple dependencies includes verifying that the component uses all of the dependencies that are injected into it via its constructor. To test a component with multiple dependencies, we can use Angular's testing utilities to create a test bed and configure it with the component's dependencies. This entails using jasmine to create mock dependencies. Each dependency is created using the createSpyObj method and then provided to the test bed via the provider's property.
After the dependencies are given, we can build an instance of the component and check to see if it uses all of the injected dependencies correctly. This can include ensuring that the component's methods and properties behave as expected, based on the functionality provided by each of the injected dependencies.
It is critical to test that the component correctly handles all dependencies, including any edge cases or error scenarios that may arise due to the interaction of the various dependencies. We can accomplish this by including relevant test data and scenarios in the Angular test case.
- Test that the component handles service dependency: Service dependencies in Angular refer to the use of services within a component or another service. Services are a key component of Angular applications, and they are used to provide functionality that can be shared across multiple components and modules.
The goal of testing is to ensure that the component interacts correctly with its dependencies and that the dependencies are properly injected into the component.
- Test that the component handles router dependencies: The goal of testing how the component handles router dependencies in Angular is to ensure that the component is properly integrated with the Angular Router and that it handles routing events and URL changes correctly.
We can identify any issues or errors that may arise as a result of incorrect handling of routing events or changes in the URL by testing the component's interaction with the router. This contributes to the application's overall quality and ensures that it meets the needs and expectations of its users.
- Test that the component handles HTTP dependencies: The goal of testing that the Angular component handles HTTP dependencies is to ensure that the component is properly integrated with Angular's HTTP module and that it handles HTTP requests and responses correctly.
We can identify any issues or errors that may arise as a result of incorrect HTTP requests or response handling by testing the component's interaction with the HTTP module. This contributes to the application's overall quality and ensures that it meets the needs and expectations of its users. It also helps to reduce the risk of bugs and other problems that can occur when components are not properly integrated with the HTTP module.

Free Angular Test Case Template
Note : We have compiled all Angular test cases for you in a template format. Feel free to comment on it. Check it out now!!
- Test that the component handles observable data: The goal of testing how an Angular component handles observable data is to ensure that the component correctly handles asynchronous data streams returned by observables.
Observables are a key feature of the RxJS library in Angular, and they are frequently used to represent asynchronous data streams like HTTP requests, user events, or other data sources. When working with observables, it is critical to ensure that the component correctly subscribes to and handles the observable's data, as well as any errors or completion events.
We can detect any problems or errors that might develop as a result of improper handling of asynchronous data streams by checking that the component properly handles observable data. This contributes to the application's overall quality and ensures that it meets the needs and expectations of its users.
- Test that the component handles subscriptions: To test the component handles Subscription in Angular involves some easy steps to take:
- Subscribing to ngOnInit()-Subscribing to observables in the ngOnInit() lifecycle hook is the best practice because it ensures that the subscription is set before the component is displayed to the user.
- Unsubscribe in ngOnDestroy()-When subscribing to observables, it is important to unsubscribe them when the component is destroyed to avoid memory leaks. You can do this in the ngOnDestroy() lifecycle hook.
- Using asynchronous channels-Using asynchronous channels in component models helps simplify subscription management because it automatically subscribes and unsubscribes observables for you.
- Using the takeUntil operator -The takeUntil operator can be used to automatically unsubscribe from an observable when a component is destroyed. You can create a Subject in your component and use the takeUntil operator to unsubscribe from the observable when the Subject emits.
- Test that the component handles data streams: This Angular test case aims to ensure that the component receives and processes data streams from various sources, such as services or the Angular framework itself. The testing procedure entails ensuring that the component Subscribes to data streams correctly and correctly handles errors and edge cases. The received data is used to update the component view or other components and prevent memory leaks, and unsubscribes from data streams when the component is destroyed.
Testing these aspects of data streams in components helps to ensure that the application behaves as designed and provides the best user experience. It also aids in identifying potential data handling issues early in the development process, allowing for quick resolution before deployment.
- Test that the component handles lazy loading: The goal of testing how an Angular component responds to lazy loading is to confirm that the component loads and renders correctly when the feature module to which it belongs uses lazy loading. Only loading the necessary code when necessary, makes sure that the application's performance is optimized. This can be especially helpful for larger applications with multiple feature modules. When lazy loading is handled correctly, the component won't be loaded until it is required, which can speed up the application's initial load.
- Test that the component handles dynamic loading: In this section, we will see that the component supports dynamic loading in Angular. This Angular test case ensures that the component is properly loaded and displayed when it is dynamically constructed or loaded at runtime. This is crucial when components need to be built dynamically as a result of user input or other runtime variables. Managing dynamic loading correctly guarantees that the component is generated, and loaded and that any associated data or functionality is also accurately loaded and functional. We can make sure that the application works as planned and offers a good user experience by ensuring that the component handles dynamic loading correctly.
- Test that the component handles asynchronous loading: Asynchronous loading in Angular refers to the procedure of non-blocking loading data and functionality, allowing the application to run while the data is being loaded. There are many ways to implement asynchronous loading, including promises, observables, and the async/await keywords.
Asynchronous loading is frequently used in the context of Angular to enhance the functionality and user experience of web applications by enabling data to be loaded in the background without impeding the user interface. When loading big volumes of data or data from a remote server, where the loading procedure may take some time, asynchronous loading is very crucial.
- Test that the component handles data caching.: The goal of testing how the component handles data caching in Angular is to ensure that the component is properly caching data to improve application performance. It refers to the practice of storing data in memory or on disk so that it can be quickly retrieved and reused later. (Caching is an important technique for improving the performance of web applications, particularly those that rely on external data sources such as APIs or databases).
There are a few different approaches to data caching in Angular, including using Angular's built-in caching mechanisms or implementing custom caching logic. One common approach is to use the HTTP interceptor in Angular to intercept HTTP requests and responses, and to cache the response data in memory or on disk. This can help to reduce the number of requests made to the server, improving the application's performance and reducing server load.
Another approach is to use a dedicated caching library such as ngx-cache, which provides a simple and flexible API for caching data in Angular applications. This library supports both in-memory and persistent caching and can be easily configured to meet the specific needs of an application.
The overall efficiency and user experience of Angular apps, especially those that depend on external data sources, can be improved by validating that the component handles data caching appropriately.
- Test that the component handles form submission: This Angular test case determines whether the component properly handles form data submission.This test usually consists of simulating a form submission event and ensuring that the component handles the event correctly.
To run this test, the developer usually writes a Angular test case that initialises the component and populates the form data with appropriate values.The test case then simulates a form submission event by triggering the submit event on the form element programmatically. The test case then validates that the component handled the form submission correctly, typically by ensuring that the appropriate data was sent to the server or that the component took the appropriate actions based on the form data .
In addition to ensuring that it handles successful form submissions your tests should ensure that it handles validation errors and other potential issues that may arise during form submissions. Modelling invalid form data and ensuring the component responds appropriately, such as displaying an error message or preventing form submission, are examples of this.
Overall, making sure your Angular component handles form submissions is an important part of making sure your component works properly and provides a smooth user experience when submitting forms from your application.
- Test that the component handles form reset: The Test that the component handles form reset in Angular is a test that determines whether the component properly handles form data resetting. This test typically consists of simulating a form reset event and ensuring that the component correctly resets the form data and state.
To run this test, the developer usually writes a Angular test case that initializes the component and populates the form data with appropriate values. The test case then simulates a form reset event by triggering the reset event on the form element programmatically. The test case then verifies that the component correctly reset the form data and state, typically by ensuring that the form controls have been reset to their default values or that any errors have been corrected.
The Angular test case then validates that the component correctly reset the form data and state, typically by ensuring that the form controls have been reset to their default values and that any errors or validation states have been cleared.
In addition to ensuring that the component handles successful form resets, the test should ensure that the component handles potential issues that may arise during form resets. This may entail simulating a reset event after the form data has been submitted or when the form is in a different state than expected, and ensuring that the component responds appropriately.
- Test that the component handles form validation: This Angular test case determines whether the component handles form validation correctly. It usually includes simulating user input on a form control and ensuring that the component is validating the input and updating the form state correctly.
To run this test, the developer typically writes a Angular test case that initializes the component and configures the form with the appropriate validators. The test case then simulates user input on a form control by setting its value programmatically and triggering the appropriate input events. The Angular test case then validates that the component validated the input correctly, usually by checking the validity state of the form control or the form as a whole.
It ensures that the component handles potential issues that may arise during validation. This may entail simulating invalid inputs or inputs that result in multiple validation errors, and ensuring that the component displays error messages correctly or disables the form submission button until the input is valid.
- Test that the component handles form errors: This Angular test case determines whether the component handles form data resetting correctly. It normally involves simulating a form reset event and ensuring that the component correctly resets the form data and state.
To run this test, the developer typically writes a Angular test case that initializes the component and populates the form data with appropriate values before simulating a form reset event by programmatically triggering the reset event on the form element. Following that, it ensures that the component has correctly reset the form data and state, typically by ensuring that the form controls have been reset to their default values and that any errors or validation states have been cleared.
The component handles any issues that may arise during form resets. This may involve simulating a reset event when the form data has already been submitted or when the form is in a different state than expected, and ensuring that the component responds appropriately.
Overall, testing how the component handles form reset in Angular is an important part of ensuring that the component is working properly and that the user experience for resetting form data in the application is smooth.
- Test that the component handles form feedback: In this section, we will see the component handles form feedback in Angular which validates that the component is giving the user accurate feedback on the form's state. This test often consists of simulating various user activities with the form and determining whether the component is responding to the proper feedback.
To perform this test, the developer typically creates a Angular test case that initializes the component and sets up the form with appropriate validators then it simulates various user interactions with the form, such as submitting the form with valid or invalid data, resetting the form, or updating the form controls.
The Angular test case then checks that the component is providing appropriate feedback to the user in response to these interactions. For example, if the user submits the form with invalid data, the test case might check that the component is correctly displaying error messages next to the relevant form controls or highlighting them with a red border. If the user resets the form, the test case might check that the component is clearing any error messages and resetting the form controls to their initial state.
- Test that the component handles form submission with HTTP requests: It's crucial to test an Angular component's ability to submit forms using HTTP requests to guarantee that the form data is transmitted and received properly. To test this functionality, we can use Angular's HttpClientTestingModule to create a mock HTTP service and a test component that includes the actual component being tested as well as the mock HTTP service. Then we can simulate user input, trigger form submission, and use Jasmine's expect method to ensure that the onSubmit method was called and the mock HTTP service received the correct data in its request. Form submission testing with HTTP requests is critical for developing reliable and robust applications and providing a better user experience.
- Test that the component handles form submission with mock data: This Angular test case involves testing the functionality of a form designed to submit data to a server using a mock service rather than sending actual HTTP requests. This type of testing is typically performed during the development process to detect bugs and ensure that the form submission functionality works as expected. To create a mock test that returns a mock response instead of sending an HTTP request to a server achieved by using Spy object and the jasmine.createSpyObj() method.
Here are some steps to create a Angular test case:
- Make a test component that contains both the actual component being tested and the mock form service. This test component will allow us to isolate the form submission handling and simulate user input.
- Create an instance of the test component in the test and use the ComponentFixture object to access the component's properties and methods.
- Change the form data and start the change detection cycle to simulate user input and get a reference to the form element and fire a submit event, which calls the component's onSubmit() method.
- Use the Jasmine expect () function to ensure that the mock service has been called with the correct data.
- Test that the component handles form submission with real data: To ensure that an Angular component handles form submission with real data, you need to create a unit test that simulates a user filling out the form and submitting it with real data. The test should perform the following functions:
- Create a test environment for the component and its dependencies
- Make a fixture to hold the component
- Fill out the form with real data by using the setValue() method to set the values of the form controls.
- Call the component's onSubmit() method to initiate the form submission.
- Expect the form submission to be successful and the component to behave correctly based on the data submitted.
- Test that the component handles form submission with error handling: The goal of testing how an Angular component handles form submission with error handling is to ensure that the component can handle and respond to invalid user input. You can make sure the component correctly displays error messages and prevents the submission of invalid data by testing the component's behaviour when invalid data is submitted. This type of testing is necessary to ensure that your application is robust and user-friendly, as well as that it can handle unexpected user input. You can prevent application crashes and improve the overall user experience by detecting errors early and handling them gracefully.
- Test that the component handles form submission with success handling: The goal of testing how an Angular component handles form submission with success handling is to ensure that the component can handle and respond to validate user input. You can ensure that the component correctly processes the data and provides appropriate feedback to the user by testing its behaviour when valid data is submitted. This type of testing is essential to ensuring that your application is working properly and providing a positive user experience. By testing the successful handling of form submissions, you can ensure that your application can meet the needs of your users and achieve your application's goals.
- Test that the component handles reactive forms: The goal of testing a component's ability to handle reactive forms in Angular is to confirm that the component is properly handling form inputs and states using the Reactive API Forms (Reactive forms enable you to respond to form inputs and state changes more reactively and functionally. RxJS Observables can be used to listen for changes to form inputs and state, and operators like map, filter, and debounceTime can be used to transform and manipulate those values.) that Angular provides. To maintain a solid and maintainable codebase, it's crucial to make sure your components are using reactive forms correctly. Reactive forms enable a more declarative approach to building forms and managing form states.
The proper handling of form inputs and state changes, such as validation, error handling, and submission, can be verified by this Angular test case. This type of testing is necessary to ensure that your application is working properly and that the user experience is positive. You can prevent application crashes and improve the overall user experience by detecting errors early and handling them gracefully. Furthermore, testing the component's reactive forms usage can help ensure that your codebase is maintainable and extensible as you add new form inputs and functionality to your application over time.
- Test that the component handles template-driven forms: In this section, we will see the test that handles template-driven forms cases which makes sure that the component is properly handling form inputs and states using the Template-driven Forms API that Angular provides. In comparison to reactive forms, template-driven forms are built on top of Angular's directive system and provide an easy-to-use approach to building forms. You can ensure that a component is correctly handling form inputs and state changes, such as validation, error handling, and submission, by testing that it handles template-driven forms correctly.
This type of testing is essential to ensuring that your application is working properly and providing a positive user experience. You can prevent application crashes and improve the overall user experience by detecting errors early and handling them gracefully.
- Test that the component handles form arrays: The goal of testing how an Angular component handles form arrays is to ensure that the component can manipulate and manage form arrays properly. This involves altering the form controls' values in the array, adding or removing controls from the array, and validating the inputs within the form array.
By testing that the component handles form arrays correctly, We can ensure that the component is functioning as expected and that any user input is properly captured and processed by testing that it handles form arrays correctly. Furthermore, this type of testing can help identify any potential issues or bugs within the component's code, allowing developers to address and resolve any issues as soon as possible.
To test that the component handles form arrays in Angular, we would create a set of Angular test cases that cover various scenarios, such as adding or removing form controls, updating values, and validating input. These Angular test cases should be designed to simulate real-world use cases and user behavior.
- Test that the component handles form groups: The goal of testing how an Angular component handles form groups is to make sure that the component can interact with and suitably manipulate form data. Form groups must be tested because they are an essential component of many web applications and, if not handled properly, can be a source of errors or user frustration.
We can confirm that the component is correctly validating and processing user input as well as displaying any errors or messages to the user by testing the component's capacity to handle form groups. This can help ensure that the form is simple to use and understand, resulting in a better user experience.
Furthermore, testing in groups can assist in identifying any potential bugs or problems in the component's code, ensuring that it is operating as intended and preventing any unexpected behaviour or errors in the application.
- Test that the component handles form controls: In this section, we will see how to test that the component handles form controls which is important because forms are a common feature in web applications, and they generally involve complex interactions between the user, the application, and the back-end server. It ensures that the component is handling user input correctly, validating user input, and sending the correct data to the back-end server.
Testing the form controls in an Angular component can help to identify the following issues:
- Validation errors: Angular provides a built-in validation system for form controls, which ensures that user input is valid before it is sent to the back-end server.
- User interactions: Forms often involve complex interactions between the user and the application, including button clicks, input changes, and form submissions.
- User experience: Forms are an important part of the user experience, and testing the form controls ensures that the form is intuitive, easy to use, and provides useful feedback to the user.
- In summary, it ensures that the component is correctly handling user input, validating user input, sending the correct data to the back-end server, and providing a good user experience.
- Test that the component handles form validators: To make sure that an Angular component is correctly validating user input and giving the user the right feedback, it is important to test how the component handles form validators. Form validators are used to make sure that user-entered data satisfies specific requirements, such as being in a required field, falling within a certain range, or fitting a particular pattern. We can ensure that the component is correctly validating user input and informing the user of any errors or messages as needed by testing the component's capacity to handle form validators.
Incorrect validation rules or unexpected behaviour when certain criteria are met are just two examples of potential problems or bugs in the component's validation logic that can be found by testing form validators. We can help ensure that the component provides accurate and helpful feedback to the user and that any errors or issues are communicated and easy to understand by catching these issues early in the testing process.
In conclusion, testing form validators is crucial for ensuring the form's overall quality and usability. It can also enhance user satisfaction by providing concise and useful feedback during the form submission process.
- Test that the component handles form patterns: The goal of testing how an Angular component handles form patterns is to ensure that the component is validating user input based on specific patterns or formats. Form patterns are used to ensure that user input, such as a phone number or an email address, matches a specific pattern or format. We can confirm that the component is accurately validating user input and communicating any errors or messages to the user as needed by testing its capacity to handle form patterns.
Testing form patterns can assist in identifying any potential issues or bugs in the validation logic of the component, such as incorrect pattern matching or unexpected behaviour when certain patterns are encountered.
- Test that the component handles form error messages: The goal of testing how an Angular component handles form error messages is to ensure that the component displays clear and helpful error messages to the user when issues with the form arise.
It is used to inform the user about specific problems with the form, such as missing required fields, invalid input, or incorrect formatting. We can verify that the component's ability to handle form error messages is correct by testing its ability to display error messages clearly and understandably and that users can easily identify and address any issues with the form. can be tested to identify any potential issues or bugs in the error message logic of the component, such as incorrect error message formatting or unexpected behaviour when certain errors occur. We can help ensure that the component provides accurate and helpful feedback to the user and that any errors or issues are communicated and easy to understand by catching these issues early in the testing process.
Overall, it is an important part of ensuring the form's overall quality and usability and can help improve the user experience by providing clear and helpful feedback throughout the form submission process, and ensuring that users can successfully submit the form without any issues.
- Test that the component handles form feedback messages: In this test, we will ensure that the component displays clear and helpful feedback messages to the user after the form has been successfully submitted. Form feedback messages inform the user that the form has been successfully submitted and may also include additional information or instructions about the submission process. We can verify that the component's ability to handle form feedback messages is correct by testing its ability to display feedback messages in a clear and understandable manner so that users can easily understand the status of their submissions.
It can assist in identifying any potential issues or bugs in the feedback message logic of the component, such as incorrect formatting or unexpected behaviour when certain conditions are met. We can help ensure that the component provides accurate and helpful feedback to the user and that any feedback or messages are clearly communicated and easy to understand by catching these issues early in the testing process.

Free Angular Test Case Template
Note : We have compiled all Angular test cases for you in a template format. Feel free to comment on it. Check it out now!!
- Test that the component handles from disabled state: In this Angular test case, it ensures that the component handles the form disabled state to ensure that the component disables or enables the form and its controls correctly based on specific conditions. The form disabled state is used to disable or enable form controls based on specific conditions, such as whether specific fields are completed or whether the user has the necessary permissions to submit the form. We can verify that the component is correctly disabling or enabling the form and its controls based on the appropriate conditions by testing its ability to handle the form disabled state.
- Test that the component handles form read-only state: The purpose of testing how an Angular component handles a form read-only state is to ensure that the component correctly displays the form in a read-only state when necessary. It is generally used to display the form in read-only mode, preventing the user from changing the form data. This is frequently used to show the user information that cannot be edited, such as a confirmation page or a summary of previously entered data. We can verify that the component is correctly displaying the form in read-only mode when appropriate by testing its ability to handle form read-only state.
This ensures that the component is providing a consistent and clear user experience and that the user can effectively interact with the form and its data, even in read-only mode. These Angular test cases could be implemented using Angular's testing framework, and they could include creating mock data and simulating user interactions to ensure that the component behaves correctly in different scenarios.
- Test that the component handles form required state: The goal of testing how an Angular component handles form required state is to ensure that the component correctly sets the form controls based on specific conditions.
The form required state indicates that a form control must have a value entered for the form to be submitted successfully. We can verify that the component is correctly setting the form controls as required based on the appropriate conditions by testing its ability to handle the form required state. The form required state indicates that a form control must have a value entered for the form to be submitted successfully. We can verify that the component is correctly setting the form controls as required based on the appropriate conditions by testing its ability to handle form required state.
- Test that the component handles form optional state: In this section, we will look at the test of an Angular component that handles form an optional state which ensures that the component is handling optional fields appropriately and that the user may interact with them as expected. The term "form optional state" describes a condition in which a user may choose whether or not to fill out specific fields on a form.
By testing that a component handles form optional state, It ensures that users can interact with the form as intended, filling out only the fields they wish to fill out without being prevented from submitting the form. This can lead to a more positive user experience and fewer user errors, as users are not forced to fill out unnecessary fields and can focus on the information they wish to provide.
- Test that the component handles form values: Testing that a component handles form values in Angular is important to ensure that user input is handled correctly and that the component behaves as expected when the user interacts with it.
Angular applications often rely heavily on forms for user input, and testing that a component handles form values is an important part of ensuring the correctness and reliability of your application.
- Test that the component handles form reset with default values: The purpose of testing that a component handles form reset with default values in Angular is to ensure that the component correctly resets the form and its controls to their default values after the user has entered data and submitted the form.
Form reset with default values clears out any data entered by the user and returns the form to its original state, allowing the user to start over with a new form. We can verify that the component is correctly resetting the form and its controls to their default values after the user submits the form by testing its ability to handle form reset with default values. It ensures that the component is providing a clear and consistent user experience and that the user can easily reset the form and start over as needed.
- Test that the component handles form reset with custom values: The goal of testing how an Angular component handles form reset with custom values is to ensure that the component properly resets the form and its controls to the custom values supplied by the user or by the component logic, rather than the default values.
Form reset with custom values is used to clean out any data provided by the user and reset the form to a specified state that may be more relevant to the user's needs or the logic of the component. We can validate that the component is appropriately resetting the form and its controls by evaluating its ability to handle form reset with custom values. It assists in identifying any potential issues or bugs in the component's logic for resetting the form, such as incorrect values being set or unexpected behaviour when certain conditions are met. We can help ensure that the component provides a clear and consistent user experience and that the user can easily reset the form to the desired state by catching these issues early in the testing process.
- Test that the component handles form reset with empty values: The goal of Angular form reset with empty values testing is to ensure that the component correctly resets the form and its controls to an empty state with no values set. It removes any data entered by the user and returns the form to a blank slate. We can verify that the component is correctly resetting the form and its controls to an empty state by testing its ability to handle form reset with empty values. It helps identify any potential issues or bugs such as incorrect values being set or unexpected behaviour when certain conditions are met.
The steps to test form reset with empty values are similar to testing form reset with default values, but this time the initial values will be empty and there will be no custom values defined.
- Test that the component handles form reset with null values: Handling form reset with null values in Angular refers to a component's ability to reset a form to its initial state, with all form fields cleared and their values set to null.
When a user interacts with a form, they may wish to clear all input fields and restart. This is accomplished in Angular by calling the reset() method on the form element, which returns the form to its initial state. However, if the form contains any pre-populated values, the reset() method may not clear these values. The component must handle the reset functionality with null values to ensure that the form is completely cleared and all values are set to null. In Angular, the component can define a resetForm() method that resets the form with null values for all fields to handle form reset with null values. This can be accomplished by calling the setValue() method on each form control and specifying null as the new value.