Best Mockito-kotlin code snippet using test.MockMaker
UnitTestImplTest.kt
Source:UnitTestImplTest.kt
...138 DescriptorProto {139 options = DescriptorProtos.MessageOptions.newBuilder().setMapEntry(false).build()140 }141 )142 val mockMaker = UnitTestImpl.DefaultMockMaker143 assertThat(mockMaker.getMockValue(types, null)).isEqualTo("mock()")144 val fieldInfo: ProtoFieldInfo = mock {145 on { field } doReturn FieldDescriptorProto {146 type = DescriptorProtos.FieldDescriptorProto.Type.TYPE_MESSAGE147 typeName = ".my.test.type"148 }149 }150 assertThat(mockMaker.getMockValue(types, fieldInfo)).isEqualTo("mock()")151 }152 @Test153 fun `can generate sensible enum values`() {154 val enumDescriptor: DescriptorProtos.EnumDescriptorProto = mock {155 on { valueList } doReturn listOf(156 DescriptorProtos.EnumValueDescriptorProto.newBuilder()157 .setName("THE_ONE")158 .build(),159 DescriptorProtos.EnumValueDescriptorProto.newBuilder()160 .setName("TWO")161 .build()162 )163 }164 whenever(types.hasProtoEnumDescriptor(eq(".my.test.type"))).doReturn(true)165 whenever(types.getProtoEnumDescriptor(eq(".my.test.type"))).doReturn(enumDescriptor)166 whenever(types.getKotlinType(eq(".my.test.type"))).doReturn(ClassName("my.test", "Type"))167 val mockMaker = UnitTestImpl.DefaultMockMaker168 val fieldInfo: ProtoFieldInfo = mock {169 on { field } doReturn FieldDescriptorProto { typeName = ".my.test.type" }170 }171 assertThat(mockMaker.getMockValue(types, fieldInfo)).isEqualTo("Type.THE_ONE")172 }173 @Test174 fun `can generate sensible primitive values`() {175 whenever(types.hasProtoEnumDescriptor(any())).doReturn(false)176 val mockMaker = UnitTestImpl.DefaultMockMaker177 assertMockValueForPrimitive(178 mockMaker,179 "\"hi there!\"",180 { type = DescriptorProtos.FieldDescriptorProto.Type.TYPE_STRING }181 )182 assertMockValueForPrimitive(183 mockMaker,184 "true",185 { type = DescriptorProtos.FieldDescriptorProto.Type.TYPE_BOOL }186 )187 assertMockValueForPrimitive(188 mockMaker,189 "2.0",190 { type = DescriptorProtos.FieldDescriptorProto.Type.TYPE_DOUBLE }191 )192 assertMockValueForPrimitive(193 mockMaker,194 "4.0",195 { type = DescriptorProtos.FieldDescriptorProto.Type.TYPE_FLOAT }196 )197 assertMockValueForPrimitive(198 mockMaker,199 "2",200 { type = DescriptorProtos.FieldDescriptorProto.Type.TYPE_INT32 },201 { type = DescriptorProtos.FieldDescriptorProto.Type.TYPE_UINT32 },202 { type = DescriptorProtos.FieldDescriptorProto.Type.TYPE_FIXED32 },203 { type = DescriptorProtos.FieldDescriptorProto.Type.TYPE_SFIXED32 },204 { type = DescriptorProtos.FieldDescriptorProto.Type.TYPE_SINT32 }205 )206 assertMockValueForPrimitive(207 mockMaker,208 "400L",209 { type = DescriptorProtos.FieldDescriptorProto.Type.TYPE_INT64 },210 { type = DescriptorProtos.FieldDescriptorProto.Type.TYPE_UINT64 },211 { type = DescriptorProtos.FieldDescriptorProto.Type.TYPE_FIXED64 },212 { type = DescriptorProtos.FieldDescriptorProto.Type.TYPE_SFIXED64 },213 { type = DescriptorProtos.FieldDescriptorProto.Type.TYPE_SINT64 }214 )215 }216 private fun assertMockValueForPrimitive(217 mockMaker: MockMaker,218 expectedValue: String,219 vararg builders: DescriptorProtos.FieldDescriptorProto.Builder.() -> Unit220 ) {221 for (builder in builders) {222 val descriptor = DescriptorProtos.FieldDescriptorProto.newBuilder()223 descriptor.apply(builder)224 assertThat(mockMaker.getMockValue(types, mock {225 on { field } doReturn descriptor.build()226 })).isEqualTo(expectedValue)227 }228 }229}...
CustomMockMaker.kt
Source:CustomMockMaker.kt
...14 * limitations under the License.15 */16package androidx.core.util.mockito17import android.os.Build18import com.android.dx.mockito.DexmakerMockMaker19import com.android.dx.mockito.inline.InlineDexmakerMockMaker20import org.mockito.invocation.MockHandler21import org.mockito.mock.MockCreationSettings22import org.mockito.plugins.InlineMockMaker23import org.mockito.plugins.MockMaker24/**25 * There is no official supported method for mixing dexmaker-mockito with dexmaker-mockito-inline,26 * so this has to be done manually.27 *28 * Inside the build.gradle, dexmaker-mockito is taken first and preferred, and this custom29 * implementation is responsible for delegating to the inline variant should the regular variant30 * fall to instantiate a mock.31 *32 * This allows Mockito to mock final classes on test run on API 28+ devices, while still33 * functioning for normal non-final mocks API <28.34 *35 * This class is placed in the core sources since the use case is rather unique to36 * [androidx.core.content.pm.PackageInfoCompatHasSignaturesTest], and other testing solutions should37 * be considered before using this in other modules.38 */39class CustomMockMaker : InlineMockMaker {40 companion object {41 private val MOCK_MAKERS = mutableListOf<MockMaker>(DexmakerMockMaker()).apply {42 // Inline only works on API 28+43 if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.P) {44 this += InlineDexmakerMockMaker()45 }46 }47 }48 override fun <T> createMock(settings: MockCreationSettings<T>, handler: MockHandler<*>): T? {49 var lastException: Exception? = null50 MOCK_MAKERS51 .filter { it.isTypeMockable(settings.typeToMock).mockable() }52 .forEach {53 val mock = try {54 it.createMock(settings, handler)55 } catch (e: Exception) {56 lastException = e57 null58 }59 if (mock != null) {60 return mock61 }62 }63 lastException?.let { throw it }64 return null65 }66 override fun getHandler(mock: Any?): MockHandler<*>? {67 MOCK_MAKERS.forEach {68 val handler = it.getHandler(mock)69 if (handler != null) {70 return handler71 }72 }73 return null74 }75 override fun resetMock(76 mock: Any?,77 newHandler: MockHandler<*>?,78 settings: MockCreationSettings<*>?79 ) {80 MOCK_MAKERS.forEach {81 it.resetMock(mock, newHandler, settings)82 }83 }84 override fun isTypeMockable(type: Class<*>?): MockMaker.TypeMockability? {85 MOCK_MAKERS.forEachIndexed { index, mockMaker ->86 val mockability = mockMaker.isTypeMockable(type)87 // Prefer the first mockable instance, or the last one available88 if (mockability.mockable() || index == MOCK_MAKERS.size - 1) {89 return mockability90 }91 }92 return null93 }94 override fun clearMock(mock: Any?) {95 MOCK_MAKERS.forEach {96 (it as? InlineMockMaker)?.clearMock(mock)97 }98 }99 override fun clearAllMocks() {100 MOCK_MAKERS.forEach {101 (it as? InlineMockMaker)?.clearAllMocks()102 }103 }104}...
2016-9f30dd7283f90d50dc9a3d1e.awesome.kts
Source:2016-9f30dd7283f90d50dc9a3d1e.awesome.kts
...36 assertEquals(300.0, amount)37}38```39but on running it, Mockito would complain indicating that it cannot mock a class/method that is final.40## MockMaker41[Mockito 2](http://mockito.org/) solves this. With the recent [release of version 2](https://github.com/mockito/mockito/wiki/What's-new-in-Mockito-2), one of the big changes is the ability to mock the un-mockable. In other words, you can mock final classes in Java and consequently all classes and member functions in Kotlin.42In order to take advantage of this feature, you write your code in exactly the same way you would for mocking an open class/function. However, you need to perform an additional step which basically consists in creating a file named `org.mockito.plugins.MockMaker` and placing this under the `resources/mockito-extensions` directory in your test folder[1].43The file contains a single line:44```45mock-maker-inline46```47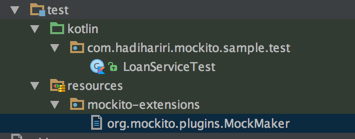 48If youâre using Gradle, you can also have it [generate the file for you.](https://github.com/hhariri/mockito-sample/blob/master/build.gradle#L16). You can download the [sample project Iâve created from GitHub](https://github.com/hhariri/mockito-sample/)49Finally, a big kudos to [Rafael](https://twitter.com/rafaelcodes) for his work in making this possible!50[1] This feature is still new and plans are to make it easier to configure as they receive feedback on its usage.51"""52Article(53 title = "Mocking Kotlin with Mockito",54 url = "http://hadihariri.com/2016/10/04/Mocking-Kotlin-With-Mockito/",55 categories = listOf(56 "Kotlin",...
DependencyPatterns.kt
Source:DependencyPatterns.kt
...53val mockitoPatterns = listOf(MOCKITO_JAR_PATTERN, MOCKITO_MVN_PATTERN)54val MOCKITO_BASIC_MODULE_PATTERN = Regex("mockito-core")55val mockitoModulePatterns = listOf(MOCKITO_BASIC_MODULE_PATTERN)56const val MOCKITO_EXTENSIONS_STORAGE = "mockito-extensions"57const val MOCKITO_MOCKMAKER_FILE_NAME = "org.mockito.plugins.MockMaker"58val MOCKITO_EXTENSIONS_FILE_CONTENT = listOf("mock-maker-inline")...
OMDBAppTest.kt
Source:OMDBAppTest.kt
...22 fun setup() {23 MockitoAnnotations.initMocks(this);24 //dataStore = Mockito.mock(OMDBContentDataStore::class.java)25 /*26 java.lang.AbstractMethodError: org.powermock.api.mockito.internal.mockmaker.PowerMockMaker.isTypeMockable(Ljava/lang/Class;)Lorg/mockito/plugins/MockMaker$TypeMockability;27 at org.mockito.internal.util.MockUtil.typeMockabilityOf(MockUtil.java:29)28 at org.mockito.internal.util.MockCreationValidator.validateType(MockCreationValidator.java:22)29 at org.mockito.internal.creation.MockSettingsImpl.validatedSettings(MockSettingsImpl.java:186)30 */31 }32 @Mock33 private lateinit var dataStore: OMDBContentDataStore34 @Mock35 private lateinit var repository: OMDBContentRepository36 @Mock37 private lateinit var useCase: GetContentsUseCase<Map<String, String>>38 private fun handleContentsFound(contents: List<Content?>) {39 this.contents = contents.toMutableList()40 }...
NoteListPresenterTest.kt
Source:NoteListPresenterTest.kt
...14 private val note = Note(1, "Title", "Body")15 private val notes: List<Note> = listOf(note)16 private val scope: CoroutineScope = TestCoroutineScope()17 /**18 * ADR # 17. Testing: use Mockito MockMaker for mocking in unit tests19 */20 private val view: NoteListViewContainer = mock()21 private val navigation: NoteListNavigation = mock()22 private val service: NoteService = mock()23 private val sut = NoteListPresenter(view, navigation, service, scope)24 @Test25 fun `after initialization note will be loaded`() = runBlocking {26 whenever(service.getNotes()).thenReturn(notes)27 sut.start()28 verify(view).setLoading(true)29 verify(service).getNotes()30 verify(view).showNotes(notes)31 }32 @Test...
UtilityTest.kt
Source:UtilityTest.kt
...16 fun test_functionUnderTest() {17 /**18 * ì½í린ìì 모ë í´ëì¤ê° 기본ì ì¼ë¡ final19 * ê°ì ë°©ë² : open classë¡ ë§ë¤ê±°ë ìë ë°©ë²20 * > org.mockito.plugins.MockMaker21 */22 val classUnderTest = mock(Utility::class.java)23 classUnderTest.functionUnderTest()24 verify(classUnderTest).functionUnderTest() // ë©ìëê° í¸ì¶ ëìëì§25 }26 @Test27 fun testSharedPreference() {28 val sharedPreference = mock(SharedPreferences::class.java)29 `when`(sharedPreference.getInt("random_int", -1))30 .thenReturn(1)31 .thenReturn(123)32 assertEquals(sharedPreference.getInt("random_int", -1), 1)33 assertEquals(sharedPreference.getInt("random_int", -1), 123)34 }...
ContainerTest_Final.kt
Source:ContainerTest_Final.kt
...8import org.mockito.Mock9import org.mockito.junit.MockitoJUnitRunner10/**11 * In order for this to work, rename file in test/resources/mockito-extensions from12 * 'MockMakerToRename' to 'org.mockito13 * .plugins.MockMaker'. However, this slows down running of unit tests by about 5 seconds14 *15 * Shows how even mocking of a final class 'DependencyImpl' is possible with MockMaker, which was16 * added to in Mockito 217 */18@RunWith(MockitoJUnitRunner::class)19class ContainerTest_Final {20 @Mock lateinit var dependency : DependencyImpl21 @Mock lateinit var dataObject : DataObject22 lateinit var container : Container23 @Before fun setup() {24 container = Container(dependency)25 }26 @Test27 fun mockingSimpleFinalClassWorks() {28 //WHEN29 container.doTheThing(4)...
MockMaker
Using AI Code Generation
1@RunWith(MockitoJUnitRunner.class)2public class MyTest {3 private MyService myService;4 private MyController myController;5 public void test() {6 when(myService.getSomething()).thenReturn("something");7 assertThat(myController.getSomething()).isEqualTo("something");8 }9}
MockMaker
Using AI Code Generation
1 Employee employee;2 public void testCalculateGrossSalary() {3 Mockito.when(employee.getBasicSalary()).thenReturn(10000.0);4 double grossSalary = employeeService.calculateGrossSalary(employee);5 assertEquals(13000.0, grossSalary, 0.02);6 }7 public void testCalculateNetSalary() {8 Mockito.when(employee.getBasicSalary()).thenReturn(10000.0);9 Mockito.when(employee.getMedical()).thenReturn(500.0);10 double netSalary = employeeService.calculateNetSalary(employee);11 assertEquals(12450.0, netSalary, 0.02);12 }13}
Learn to execute automation testing from scratch with LambdaTest Learning Hub. Right from setting up the prerequisites to run your first automation test, to following best practices and diving deeper into advanced test scenarios. LambdaTest Learning Hubs compile a list of step-by-step guides to help you be proficient with different test automation frameworks i.e. Selenium, Cypress, TestNG etc.
You could also refer to video tutorials over LambdaTest YouTube channel to get step by step demonstration from industry experts.
Get 100 minutes of automation test minutes FREE!!