How To Take Full Page Screenshots In Selenium WebDriver
Faisal Khatri
Posted On: July 9, 2024
382092 Views
19 Min Read
Taking screenshots is one of the most common actions for any web page tester. Screenshots or videos are essential when reporting bugs, as they provide visual evidence of issues. This holds for all types of testing, including Selenium automation testing.
Automated screenshots in Selenium WebDriver are crucial for web application testing, especially with numerous commands and test cases. This is why learning how to take full page screenshots in Selenium WebDriver is essential. These screenshots help developers and testers verify that each test case executes correctly and aid in debugging to identify what went wrong. In Selenium, these screenshots clarify whether failures are due to application errors or script issues.
TABLE OF CONTENTS
Why Take Screenshots in Selenium?
When the automation tests are executed, there is a chance that they may fail for different reasons. Some tests may fail due to exceptions such as NoSuchElementException, TimeoutException, StaleElementReferenceException, and other common expectations in Selenium.
However, we may have error logs printed in the console. It is always good to have a screenshot of the actual web page where the test execution failed. The screenshots may help the tester to analyze the failure quickly. It helps to know whether the code execution reached the desired web page or if any unknown error occurred which led to the failure.
The following are some critical cases where taking screenshots could prove beneficial.
- Test failure is due to exceptions related to the WebElement not being found or an invalid selector or Timeout exception.
- Assertions failure.
- Capturing screenshots during running end-to-end automated test journeys.
Screenshots help the testers understand the exact location of the bug and the reasons behind it without going through the raw logs. It also helps to know the application’s behavior at the time of failure. This eventually helps in fixing the issues quickly.
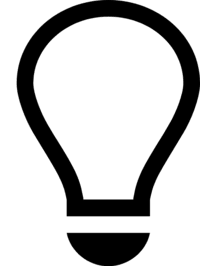
Ensure every visual inconsistency is captured correctly with screenshot testing. Try LambdaTest Today!
As we have learned why it is important to take screenshots, we will further understand how to take screenshots in Selenium WebDriver in detail below.
How To Take Screenshots in Selenium 4?
In Selenium 3, the TakeScreenShot interface was used to take a screenshot of the web page. However, it did not assist in capturing the screenshot of the WebElements.
The Selenium 4 release, which contains the major breaking changes in the Selenium WebDriver framework, provides new ways to capture screenshots.
However, Selenium 4 introduces new features for capturing screenshots and enabling BiDirectional (BiDi) connections. With the addition of the BrowsingContext class, you can now capture screenshots of both the entire web page and specific WebElements. The existing TakeScreenshot interface is still supported. To enable BiDi connections, set the “webSocketUrl” capability to true using the browser options class when instantiating the WebDriver.
If you are unfamiliar with WebSocket and WebSocket URLs, watch the video below for a basic understanding of their usage.
Example Code :
The following code showcases setting the “webSocketUrl” capability to true using the ChromeOptions class.
1 2 3 |
ChromeOptions options = new ChromeOptions(); options.setCapability("webSocketUrl", true); WebDriver driver = new ChromeDriver(options); |
In case the above capability is not provided, the following exception will be thrown by Selenium:
1 |
org.openqa.selenium.bidi.BiDiException: Unable to create a BiDi connection |
Capturing Screenshots in Selenium 4:
The following code example will capture the screenshot of the home page of the LambdaTest eCommerce Playground website. This test will be run on the local machine on the Chrome browser.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
public class ScreenshotWithSeleniumTest { private WebDriver driver; @BeforeTest public void setup() { ChromeOptions options = new ChromeOptions(); options.setCapability("webSocketUrl", true); driver = new ChromeDriver(options); } @Test public void testTakeScreenshot() throws IOException { BrowsingContext browsingContext = new BrowsingContext(driver, driver.getWindowHandle()); driver.get("https://ecommerce-playground.lambdatest.io/"); String screenshot = browsingContext.captureScreenshot(); byte[] imgByteArray = Base64.getDecoder().decode(screenshot); FileOutputStream imgOutFile = new FileOutputStream("screenshot_homepage.png"); imgOutFile.write(imgByteArray); imgOutFile.close(); } @AfterTest public void tearDown() { driver.quit(); } } |
The testTakeScreenshot() method will use the captureScreenshot() method from the BrowsingContext class in Selenium 4. The captureScreenshot() method executes the BiDi command browsingContext.captureScreenshot
and returns the screenshot data in String format. This String can then be converted into an image using plain Java code.
The code provided converts the String returned by the captureScreenshot() method into an image. First, the String is decoded into a byte array using the Base64.getDecoder().decode() method. Then, the byte array is converted into an image using the FileOutputStream class in Java. The screenshot will be stored in the project’s root folder.
Result:
The result is displayed using IntelliJ IDE.
The following screenshot is stored in the project’s root folder when the test is executed successfully.
When we say screenshot, we could mean capturing an image of any part of the screen, including a specific element or the entire
There are four major ways to capture screenshot images using Selenium WebDriver:
- Screenshot of the viewable area.
- Screenshot of a particular web element.
- Screenshot of the entire screen, i.e., capturing the full webpage.
- Using a cloud-based platform to capture screenshots of the Application Under Test (AUT).
You can also refer to the video tutorial below on how to take screenshots while performing Selenium testing.
Subscribe to the LambdaTest YouTube Channel for more video tutorials on various automation testing techniques, including Selenium with Python, Selenium with Java, and more.
In order to further enhance your Selenium automation testing, you can consider exploring AI testing agents like KaneAI.
KaneAI is a smart GenAI native test assistant for high-speed quality engineering teams. With its unique AI-driven features for test authoring, management, and debugging, KaneAI allows teams to create and evolve complex test cases using natural language.
Taking Screenshots of Viewable Areas
This is the most used approach for taking screenshots of applications under automation and the easiest one. Selenium provides an out-of-the-box capability called TakeScreenShot interface that can take screenshots of the viewable area.
This interface provides a method called getScreenshotAs(), which helps capture and store the screenshot in the desired location.
Here’s the syntax to capture the screenshot:
1 |
File screenshotFile = ((TakesScreenshot)driver).getScreenshotAs(OutputType.FILE); |
To store the taken screenshot into a file, the below method is used:
1 |
FileUtils.copyFile(screenshotFile, new File("path of the file you want to save the screenshot to")); |
This is it! Just two methods, and you will be able to take the screenshot.
Let’s implement the above syntax into code using the following test scenario.
Test Scenario:
|
Code Implementation:
The below code is the implementation for the above test scenario. We won’t be going into details for the setup() and tearDown() methods, as they are responsible for starting and closing the Chrome browser. The actual scenario implementation will be handled using the testTakeViewableScreenshot() method.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 |
public class ScreenshotWithSeleniumTest { WebDriver driver; @BeforeTest public void setup() { driver = new ChromeDriver(); driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(30)); driver.manage().window().maximize(); } @Test public void testTakeViewableScreenshot() { driver.get("https://ecommerce-playground.lambdatest.io/"); WebElement blogMenu = driver.findElement(By.cssSelector("div.entry-section div.entry-widget ul > li:nth-child(3) > a > div > span")); blogMenu.click(); WebElement firstArticleImage = driver.findElement(By.className("article-thumb")); Actions actions = new Actions(driver); actions.scrollToElement(firstArticleImage).build().perform(); WebElement secondArticleImage = driver.findElement(By.cssSelector(".swiper-wrapper div[aria-label='2 / 10']")); actions.scrollToElement(secondArticleImage).build().perform(); File src = ((TakesScreenshot) driver).getScreenshotAs(OutputType.FILE); try { FileUtils.copyFile(src, new File("./screenshots/blogpage.png")); } catch (IOException e) { throw new RuntimeException(e); } } @AfterTest public void tearDown() { driver.quit(); } } |
The testTakeViewableScreenshot() method will navigate to the LambdaTest eCommerce Playground website, locate the blog menu, and click on it.
Once the blog page loads, it will locate the first and second article images and scroll to those elements. This scrolling ensures the images load before taking the screenshot. Finally, a screenshot will be taken and saved to the screenshots folder in the project’s root directory.
The following screenshot of the viewable screen will be taken after the test execution is successful:
Result:
After learning how to capture a screenshot of the viewable area, we will further learn the second approach: capture screenshots of desired WebElements before we learn how to take full page screenshots in Selenium WebDriver.
Taking Screenshots of Desired WebElements
Taking a screenshot of a desired element is also pretty easy. Selenium 4 provides the captureElementScreenshot() method, part of the BrowsingContext class.
It takes the WebElement ID as the parameter and returns the image data in String format. This data can further be processed using the Base64 decoder and finally written to the image file in either PNG or JPG format using the FileOutputStream class of Java.
Let’s consider the following test scenario to demonstrate the WebElement screenshot capture.
Test Scenario:
|
Code Implementation:
Here’s a code snippet demonstrating how to take a screenshot of just the First Name text field on the registration page of the LambdaTest eCommerce Playground website using Selenium WebDriver.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
public class ScreenshotWithSeleniumTest { private WebDriver driver; @BeforeTest public void setup() { ChromeOptions options = new ChromeOptions(); options.setCapability("webSocketUrl", true); driver = new ChromeDriver(options); } @Test public void testTakeElementScreenshot() throws IOException { BrowsingContext browsingContext = new BrowsingContext(driver,driver.getWindowHandle()); driver.get("https://ecommerce-playground.lambdatest.io/index.php?route=account/register"); WebElement firstName = driver.findElement(By.id("input-firstname")); String screenshot = browsingContext.captureElementScreenshot(((RemoteWebElement) firstName).getId()); byte[] imgByteArray = Base64.getDecoder().decode(screenshot); FileOutputStream imgOutFile = new FileOutputStream("./screenshots/screenshot_webelement.png"); imgOutFile.write(imgByteArray); imgOutFile.close(); } @AfterTest public void tearDown() { driver.quit(); } } |
The setup() method will be executed first before the test. It sets the capability webSocketUrl to true using the ChromeOptions class and starts the Chrome browser. This capability is required to enable the BiDi connection in Selenium. Similar capabilities can be set for other browsers like Firefox and Edge.
The testTakeElementScreenshot() method implements the test scenario. It navigates to the registration page of the LambdaTest eCommerce Playground website and locates the First Name text field using the ID locator input-first name. The captureElementScreenshot() method of the BrowsingContext class in Selenium is then called to get the screenshot of the First Name text field.
Finally, the screenshot is saved as screenshot_webelement.png in the project screenshots folder.
Result:
The following image of the First Name WebElement was taken after successfully executing the above test scenario.
Result:
As we have seen, the captureElementScreenshot() method in Selenium 4 helps take screenshots of WebElements. There are many other features offered by Selenium 4, and some functions or features are also deprecated. If you are still using Selenium 3, follow this blog post on what’s new in Selenium 4 and what’s deprecated to learn more about Selenium 4 features.
So far, we have learned how to capture screenshots of desired WebElements using the Selenium 4 captureElementScreenshot() method. This method represents an advanced approach to capturing screenshots. It is important to learn before we learn how to capture full page screenshots in Selenium WebDriver.
Taking Full Page Screenshots
There may be a need to take screenshots of the entire screen rather than just the browser’s viewport. Some browsers only take screenshots of the viewable port, whereas others take screenshots of the entire web page.
Firefox browser supports capturing entire page screenshots using Selenium, whereas Chrome, Safari, and Edge do not support capturing full page screenshots. So, the AShot library can capture screenshots of the entire screen using Selenium WebDriver.
AShot library is a WebDriver screenshot utility that captures an entire page’s screenshot and is natively supported from Selenium 3 onwards. It provides the following features:
- Helps capture the whole screen and web element.
- Beautify screenshot.
- Provides screenshot comparison.
For more details on the utility, you can refer to the WebDriver Screenshot utilities.
To take a screenshot of the entire screen, the following dependency should be added to the pom.xml file.
1 2 3 4 5 |
<dependency> <groupId>ru.yandex.qatools.ashot</groupId> <artifactId>ashot</artifactId> <version>1.5.4</version> </dependency> |
After adding the dependency, the following lines of code need to be added to the test scripts where it is intended to take a full page screenshot.
1 2 |
Screenshot screenshot=new AShot().shootingStrategy(ShootingStrategies.viewportPasting(1000)).takeScreenshot(driver); ImageIO.write(screenshot.getImage(),"PNG",new File("path of the file")); |
The Screenshot class of the AShot library is instantiated first. The viewPortPasting() method will move into the viewport by scrolling the page at 1000 milliseconds and taking the screenshot. Finally, the screenshot is converted to a PNG image.
The following test scenario will be demonstrated to capture a full page screenshot of the LambdaTest eCommerce Playground website.
Test Scenario:
|
Code Implementation:
Below is the code implementation for taking a full screenshot of the web page under test using the AShot library. This example focuses on the usage and implementation of the AShot library, assuming that the test setup and browser initialization are already handled.
A new Java class, FullPageScreenshotTest, has been created and contains the following code:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
public class FullPageScreenshotTest { WebDriver driver; @Test public void testTakeScreenshotUsingAShot() throws IOException { driver = new ChromeDriver(); driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(30)); driver.get("https://ecommerce-playground.lambdatest.io/"); Object devicePixelRatio = ((JavascriptExecutor)driver).executeScript("return window.devicePixelRatio"); String dprValue = String.valueOf(devicePixelRatio); float windowDPR = Float.parseFloat(dprValue); Screenshot screenshot = new AShot() .shootingStrategy(ShootingStrategies.viewportPasting(ShootingStrategies.scaling(windowDPR),1000)) .takeScreenshot(driver); ImageIO.write(screenshot.getImage(), "png", new File("./screenshots/AshotFullPageScreen.png")); } @AfterTest public void tearDown() { driver.quit(); } } |
The testTakeScreenshotUsingAShot() test method will help us take a full page screenshot of the home page of the LambdaTest eCommerce website. The code will open the Chrome browser and help you navigate the LambdaTest eCommerce Playground website.
To take the complete screenshot of the page from left to right and top to bottom, we must provide the scaling value using the ShootingStrategies class of the AShot library. This scaling value is the device pixel ratio of the window, which can be captured using the JavaScript executor’s command return window.devicePixelRatio
.
The value of the ratio is returned in Object format. However, we need this value in float format; hence, we will be parsing the object value to float.
The value of the device pixel ratio will be supplied to the scaling method of the ShootingStrategies class, as shown in the screenshot below.
The viewportPasting() method of the AShot library will help take a screenshot. The scaling value is added to ensure we get a complete screenshot of the web page from left to right.
The screenshot image will be saved in a PNG format in the screenshots folder in the project’s root folder.
Result:
The code will automatically scroll down when executing the test, and the entire web page will be screenshotted.
The following screenshot image was captured successfully by the test.
We saw how the AShot library could help us take screenshots of the entire page. However, we can take the full page screenshot using Selenium and Firefox browsers. Currently, browsers other than Firefox are not supported.
Test Scenario:
|
Code Implementation:
Below is the code implementation for the given test scenario. In this scenario, we will use the Firefox browser and Selenium’s getFullPageScreenshotAs() method to capture a screenshot of the entire page.
The following test method, testTakeFullPageScreenshotFirefox(), demonstrates how to capture the entire page screenshot.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 |
@Test public void testTakeFullPageScreenshotFirefox() { WebDriver driver = new FirefoxDriver(); driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(30)); driver.manage().window().maximize(); driver.get("https://ecommerce-playground.lambdatest.io/"); JavascriptExecutor js = (JavascriptExecutor) driver; Actions actions = new Actions(driver); WebElement topTrendingItemList = driver.findElement(By.className("swiper-wrapper")); js.executeScript("arguments[0].scrollIntoView(true);", topTrendingItemList); actions.pause(2000).build().perform(); WebElement exchangeOffer = driver.findElement(By.cssSelector("#entry_213263 > div > h4")); js.executeScript("arguments[0].scrollIntoView(true);",exchangeOffer); actions.pause(2000).build().perform(); WebElement bottom = driver.findElement(By.className("article-thumb")); js.executeScript("arguments[0].scrollIntoView(true);", bottom); actions.pause(2000).build().perform(); File src = ((FirefoxDriver) driver).getFullPageScreenshotAs(OutputType.FILE); try { FileUtils.copyFile(src, new File("./screenshots/fulpagescreenshot.png")); } catch (IOException e) { throw new RuntimeException(e); } driver.quit(); } |
The getFullPageScreenshotAs() method will capture the screenshot of the entire page in one go. Unlike AShot, it will not scroll the page while taking the screenshot. Hence, some lines of code have been added for scrolling the page and pausing the test for 2000 milliseconds so the images on the page load successfully, and we get a clear screenshot with all the images loaded successfully.
The steps for scrolling the page and pausing are added for demo purposes; in reality, they have nothing to do with capturing the screenshot.
Result:
Upon executing the test, the screenshot will be captured with the filename fullpagescreenshot.png and saved in the screenshots folder in the project.
The following screenshot is captured on executing the test.
Taking full page screenshots is useful, but there are cases where we need to capture screenshots of specific WebElements. For instance, we might want to screenshot a brand logo or any specific UI WebElement to verify its pixels or check for any UI-related issues. Selenium 4 supports taking screenshots of individual WebElements. Let’s learn more about how to implement it.
Running tests locally is crucial, but it has its limitations. For example, you can’t install multiple operating systems or browsers on a single machine. As projects evolve, it becomes challenging for developers and testers to ensure that websites work across various OS and browsers, including those not locally accessible.
To overcome these challenges and limitations, using cloud-based platforms is beneficial. One such platform is LambdaTest. These platforms provide seamless support for screenshots and video recordings of test automation executions. With LambdaTest, there’s no need to write code for taking screenshots; you simply pass the required capabilities, and the platform handles the rest. We will learn how to implement full page screenshots on a cloud platform.
How To Take Full Page Automated Screenshots On the Cloud?
LambdaTest is an AI-powered test execution platform that lets you run manual and automated screenshot tests at scale with over 3000+ real devices, browsers, and OS combinations.
The best part about the LambdaTest Cloud Grid is that it automatically takes screenshots of your webpage after executing each Selenium command. Additionally, this platform records videos of the entire test execution.
Using the test scenario below, let us learn how to take screenshots in Selenium WebDriver on the LambdaTest cloud platform.
Test Scenario:
|
Code Implementation:
Before we move on to implementing the test scenarios, let’s gather the necessary capabilities that need to be provided in the configuration. These capabilities will enable automatic screenshot capture and video recording of the test execution.
Follow the steps below to get started with the platform.
- Create an account on the LambdaTest platform.
- Get the LambdaTest Username and Access key from Profile > Account Settings > Password & Security tab.
- Updated the required capabilities using the LambdaTest Capabilities Generator, which provides the code after selecting the required capabilities from the UI.
The following capabilities must be passed in the code to capture screenshots and video recordings of the test execution.
Capability Name | Value | Description |
visual | true | Captures screenshots on every command |
video | true | Record a video of the test execution |
Once the necessary capabilities are gathered, you must create a new test class called LambdaTestScreenshotTest and add the details to implement the test scenario.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 |
public class LambdaTestScreenshotTest { private RemoteWebDriver driver; @BeforeTest public void setup() { final String userName = System.getenv("LT_USERNAME") == null ? "LT_USERNAME" : System.getenv("LT_USERNAME"); final String accessKey = System.getenv("LT_ACCESS_KEY") == null ? "LT_ACCESS_KEY" : System.getenv("LT_ACCESS_KEY"); final String gridUrl = "@hub.lambdatest.com/wd/hub"; try { this.driver = new RemoteWebDriver(new URL("http://" + userName + ":" + accessKey + gridUrl), getChromeOptions()); } catch (final MalformedURLException e) { System.out.println("Could not start the remote session on LambdaTest cloud grid"); } this.driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(10)); } @Test public void testScreenshotCaptureOnLambdaTest() { driver.get("https://ecommerce-playground.lambdatest.io/"); WebElement myAccountLink = driver.findElement(By.linkText("My account")); Actions actions = new Actions(driver); actions.moveToElement(myAccountLink).build().perform(); WebElement registerLink = driver.findElement(By.linkText("Register")); actions.moveToElement(registerLink).click().build().perform(); String registerPageHeader = driver.findElement(By.tagName("h1")).getText(); assertEquals(registerPageHeader, "Register Account"); WebElement loginLink = driver.findElement(By.linkText("Login")); loginLink.click(); String loginPageHeader = driver.findElement(By.cssSelector(".col-lg-6 h2")).getText(); assertEquals(loginPageHeader, "New Customer"); } public ChromeOptions getChromeOptions() { final var browserOptions = new ChromeOptions(); browserOptions.setPlatformName("Windows 10"); browserOptions.setBrowserVersion("125"); final HashMap<String, Object> ltOptions = new HashMap<String, Object>(); ltOptions.put("project", "LambdaTest Screenshot Testing demo"); ltOptions.put("name", "Capture Screenshot test"); ltOptions.put("w3c", true); ltOptions.put("visual", true); ltOptions.put("video", true); ltOptions.put("plugin", "java-testNG"); browserOptions.setCapability("LT:Options", ltOptions); return browserOptions; } @AfterTest public void tearDown() { this.driver.quit(); } } |
The setup() method will configure and set up the environment on the cloud platform using the getChromeOptions(), which supplies all the necessary capabilities. The tearDown() method will gracefully close the WebDriver session.
The testScreenshotCaptureOnLambdaTest() method will implement the test scenario as discussed earlier. It will navigate to the website’s homepage, open the registration page, verify its page header, and finally navigate to the login page, checking the page header. Assertions are included in the test to confirm that we land on the correct pages.
There is no need to include methods in the test script for capturing screenshots or recording videos. The capabilities specified in the configuration will automatically capture screenshots of each step and record the video of the entire test execution.
Result:
Upon successful execution of the tests on the LambdaTest cloud platform, the details of the test execution, screenshots, and video recordings can be found on the LambdaTest dashboard screen, as shown below.
Apart from this, LambdaTest also provides a standalone full page automated screenshot feature called Screenshot. This feature allows you to take screenshots of your application across multiple browsers and compare them. You can access these screenshots anytime, share them with stakeholders, and email them as needed.
This is particularly useful for cross-browser testing, as you can quickly identify and compare UI issues across different browsers and versions. Imagine the amount of time you save with this feature!
Conclusion
We covered all the possible combinations on how to take full page screenshots in Selenium WebDriver. We wrote some necessary code to convert the image’s raw data to a proper image file, such as PNG or JPG.
To enhance the functionality of capturing screenshots, we have used cloud platforms that offer much more robust features to capture screenshots and video recordings of the tests without writing a single line of code. In many previous projects, we have leveraged the cloud platform screenshot capturing utility and video recording to analyze the test failures.
Happy Testing!!
Frequently Asked Questions (FAQs)
How to take a screenshot in Selenium WebDriver?
There are two ways to take screenshots in Selenium WebDriver
- Using the getScreenshotAs() method from the TakesScreenshotAs interface.
- Using the BrowsingContext class in Selenium 4.
How to take a full page screenshot in the Firefox browser?
The getFullPageScreenshotAs() method can take a screenshot of an entire page in the Firefox browser. The following code snippet should help:
1 2 3 4 5 |
WebDriver driver = new FirefoxDriver(); driver.get("https://example.com"); File screenshot = ((TakesScreenshot)driver).getScreenshotAs(OutputType.FILE); FileUtils.copyFile(screenshot, new File("full_page_screenshot.png")); driver.quit(); |
Is it possible to take a full page screenshot in Chrome browser?
It is possible to screenshot an entire web page in Chrome using the AShot library.
Does Selenium allow the capture of a screenshot of WebElement?
Yes, Selenium 4 allows for capturing a screenshot of WebElement using the captureElementScreenshot() method of the BrowsingContext class.
Do we need to write code to capture screenshots on cloud platforms?
No. Cloud platforms provide the capabilities that can be used to configure capturing the screenshots of web pages and also get the video recording. No code is needed to capture screenshots if the tests are executed on cloud platforms.
Got Questions? Drop them on LambdaTest Community. Visit now