- Test that the component renders without errors: This test case ensures that the React component renders without any errors. It is the most basic and important React test case to ensure that the component is properly set up and there are no issues with rendering. The test will pass if the component is rendered without any errors or exceptions, and it will fail if any errors occur during the rendering process.
- Test that the component renders with props: This React test case ensures that the React component is rendered correctly with the appropriate props passed to it. The test involves passing the required props to the component and checking that the component is rendered with the expected output.
- Test that the component renders with state: Testing that the component renders with state is important because state is a fundamental feature of React. By testing that the component correctly renders with state, we can ensure that the component behaves as expected and that any changes to state are reflected in the rendered output.
Additionally, testing that the component renders with state can help ensure that the state is properly initialized and that any updates to the state are being correctly handled.
- Test that the component updates state when an event is triggered: This test ensures that the component is correctly updating its state when a specific event is triggered, such as a button click. It checks that the component is responding correctly to user input and updating the state as expected.
- Test that the component renders with conditional logic: This particular React test ensures that the component is rendering correctly based on specific conditions or logic. It checks that the component is correctly rendering different content based on different input or state conditions.
- Test that the component calls a function when a button is clicked: This test ensures that the component is correctly calling a specific function when a button is clicked. It checks that the component is correctly responding to user input and executing the expected behavior.
- Test that the component renders a list of items: This React test case ensures that the component is correctly rendering a list of items, such as a list of products or users. It checks that the component is correctly displaying the data in a list format.
- Test that the component updates the list when a new item is added: This test ensures that the component is correctly updating the list of items when a new item is added. It checks that the component is adding the new item to the list and that the list is correctly re-rendered to display the new item.
- Test that the component removes an item from the list when a delete button is clicked: This React test case ensures that the component is correctly removing an item from the list when a delete button is clicked. It checks that the component is correctly updating the list to remove the deleted item and that the list is correctly re-rendered to display the updated list of items.
- Test that the component sorts a list of items in ascending order: This test ensures that the component is correctly sorting a list of items in ascending order. It checks that the component is correctly re-ordering the list based on a specific sorting algorithm and that the list is correctly re-rendered to display the updated order. This test is particularly important for components that display large amounts of data and need to be able to sort and display that data efficiently.
- Test that the component sorts a list of items in descending order: This React test case ensures that the component is correctly sorting a list of items in descending order. It checks that the component is correctly re-ordering the list based on a specific sorting algorithm and that the list is correctly re-rendered to display the updated order in descending order.
- Test that the component filters a list of items based on a search term: This test ensures that the component is correctly filtering a list of items based on a search term. It checks that the component is correctly updating the list to display only the items that match the search term and that the list is correctly re-rendered to display the filtered list of items.
- Test that the component renders with nested components: This test ensures that the component is correctly rendering with nested components. It checks that the nested components are correctly rendered and displayed within the parent component and that the component hierarchy is correctly maintained.
- Test that the component renders with children components: This test ensures that the component is correctly rendering with children components. It checks that the children components are correctly rendered and displayed within the parent component and that the component hierarchy is correctly maintained.
- Test that the component passes props down to its children components: This test ensures that the component is correctly passing props down to its children components. It checks that the props are correctly passed and received by the children components and that the children components are correctly displaying the passed data.
- Test that the component passes functions down to its children components: This React test case ensures that the component is correctly passing functions down to its children components. It checks that the functions are correctly passed and received by the children components and that the children components are correctly invoking the passed functions.
- Test that the component renders with styles: This test ensures that the component is correctly rendering with styles. It checks that the styles are correctly applied to the component and that the component is correctly displaying the styled content.
- Test that the component renders with classes: This test ensures that the component is correctly rendering with classes. It checks that the classes are correctly applied to the component and that the component is correctly displaying the classed content.
- Test that the component renders with dynamic classes: This test ensures that the component is correctly rendering with dynamic classes. It checks that the classes are correctly applied to the component based on specific conditions and that the component is correctly displaying the dynamically classed content.
- Test that the component renders with dynamic styles: Testing that the component renders with dynamic styles ensures that the styles of the component can be updated dynamically based on user interaction or other events. This can help improve the interactivity and visual appeal of the component.
The React test case checks that the component can receive and apply new styles based on changes to the props or state of the component. It is important to verify that the styles are applied correctly to ensure that the component is functioning as intended.
- Test that the component renders with CSS modules: Testing that the component renders with CSS modules ensures that the CSS styles applied to the component are scoped to that component only, avoiding potential conflicts with styles in other parts of the application.
This test can involve importing and using a CSS module in the component, and verifying that the styles are applied correctly. It can also involve testing that the CSS class names generated by the CSS modules are unique and scoped to the component.
- Test that the component renders with conditional styles: Testing the component with conditional styles involves testing whether the component renders correctly when certain styles are applied or removed based on certain conditions. This ensures that the styles are correctly applied and removed based on the component's state or props.
The React test case can involve setting up the component with different props or state values that trigger different styles, and then asserting that the expected styles are applied or removed. This test can be done using a testing library like React Testing Library or Enzyme, along with a tool like Jest to run the tests.
- Test that the component renders with dynamic CSS modules: Testing that the component renders with dynamic CSS modules involves checking if the styles applied to the component are dynamic and modular in nature. This means that the styles are scoped to the component and are not global. The tests need to ensure that the styles are being applied correctly and that changes to the component's state or props affect the styles as expected.
- Test that the component renders with CSS-in-JS: Testing that a component renders with CSS-in-JS involves verifying that the styles defined using a CSS-in-JS library, such as styled-components or Emotion, are properly applied to the component. This includes testing both static and dynamic styles.
In the case of styled components, for example, a test would involve rendering a component that uses the styled React components library to define its styles, and then checking that the styles are correctly applied to the rendered output.
- Test that the component renders with media queries: When testing a React component, it's important to ensure that it renders correctly at different screen sizes. To accomplish this, you can write React test cases to check that the component renders with media queries. These tests should include assertions that the component's styles change based on the width of the viewport.
- Test that the component renders with responsive styles: Testing that a component renders with responsive styles is important to ensure that the component is appropriately displayed across different screen sizes and devices. This test helps to ensure that the component is responsive and can adapt to the viewport size.
To perform this test, the component can be rendered within a variety of viewport sizes, such as using the ‘@media’ rule to simulate different screen sizes. The rendered component can then be checked to ensure that the styles have been applied correctly and that the component is appropriately displayed.
- Test that the component renders with conditional responsive styles: In this React test case, we want to verify that our component renders with the correct styles when certain conditions are met, based on the screen size or device type. This can be achieved by using media queries or CSS frameworks such as Bootstrap or Material UI.
To test this, we can write a test that checks if the component's styles change when the screen size changes. We can use a library like Enzyme to simulate a window resize and check if the styles of our components are updated accordingly.
- Test that the component renders with dynamic responsive styles: In React applications, it is common to have components that render differently based on screen size or other factors. Testing that these responsive styles are correctly applied can be important to ensure a good user experience.
To test that a component renders with dynamic responsive styles, we can use a testing library like Enzyme or React Testing Library to simulate different screen sizes or other factors that affect the styles. We can then assert that the component renders the expected styles for each condition.
- Test that the component renders with dynamic conditional responsive styles: You perform this React test case, we are checking if the component can render with dynamic conditional responsive styles. This means that the component should be able to adjust its styles based on the screen size and also based on certain conditions.
To test this, we can pass props to the component that specifies the responsive styles to be applied based on certain conditions. We can then check that the component is rendering with the correct styles based on the props passed.
- Test that the component renders with animations: This React test case ensures that the component renders with animations that add visual interest and improve user experience. It tests that the animations are smooth and don't cause performance issues.
- Test that the component renders with dynamic animations: Test that the component renders with dynamic animations refers to testing whether a React component can render with dynamic animations. This type of testing is important because animations can be an integral part of a user interface and can enhance the user experience.
Dynamic animations can be created using various libraries like React Spring, Framer Motion, and CSS animations. The React test case involves passing dynamic animation styles or parameters to the component and verifying that the component renders with the expected animation behavior.
- Test that the component renders with conditional animations: Testing that the component renders with conditional animations ensures that the component behaves as expected based on the state of the application. This can be achieved by testing the component's animation properties when certain conditions are met or when certain events are triggered.
For example, if a button click triggers an animation, the test should verify that the animation is triggered and that the component updates as expected. By testing conditional animations, developers can ensure that the component provides a smooth and intuitive user experience, even when the application's state changes.
- Test that the component renders with dynamic conditional animations: In this React test case, we want to verify that the component can render with dynamic conditional animations. This means that the component can change its animations based on the props or state passed to it.
To test this, we can create a component that has different animation options based on the props passed to it. We can then assert that the correct animation is applied when the component is rendered with different props.
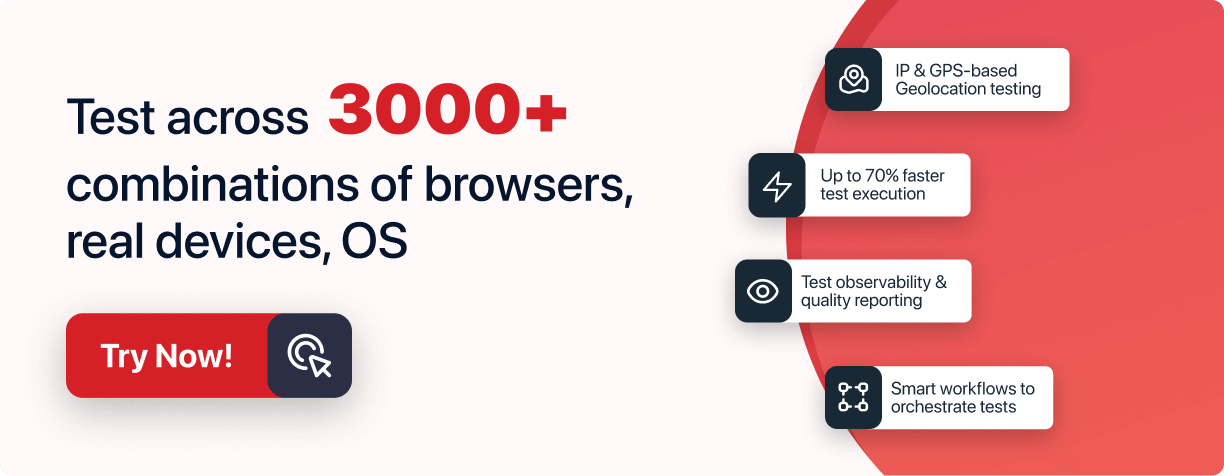
- Test that the component renders with transitions: Testing that a component renders with transitions involves checking that the specified transition animations are applied to the component when certain actions or events occur, such as when the component is mounted or updated.
This can be done using a testing library like React Testing Library or Enzyme and involves checking that the CSS classes or properties associated with the specified transitions are being correctly applied to the component's DOM elements.
- Test that the component renders with dynamic transitions: Testing that a component renders with dynamic transitions typically involves rendering a component with dynamic data and checking that the transitions are applied correctly. This could involve testing that the animation starts and stops at the correct times, that the timing and easing functions are correct, and that the animation behaves as expected under different conditions.
- Test that the component renders with conditional transitions: Testing that the component renders with conditional transitions involves verifying that the component renders properly when certain conditions are met. This can be achieved by setting the required conditions and checking if the component responds accordingly.
For example, if the transition should only occur when a certain state is active, the test should ensure that the transition occurs only when the state is active and not when it is inactive. This ensures that the component is rendering transitions properly when conditions are met and not when they are not met. The test can be performed using a testing library such as Jest or Enzyme.
- Test that the component renders with dynamic conditional transitions: Test that the component renders with dynamic conditional transitions refers to the testing of a React component's ability to render with transitions that are both dynamic and conditional.
To test this, you would need to create a component with dynamic conditional transitions and then use testing tools such as Jest and Enzyme to ensure that the transitions are working as expected. You can simulate different scenarios and conditions to ensure that the transitions are happening correctly.
- Test that the component renders with images: Test that the component renders with images refers to the testing of the ability of the component to correctly display images. This can be done by passing a source URL to the image tag and verifying that the image is rendered on the screen.
This React test case is important because images are an important component of most websites and applications, and ensuring that they are displayed correctly is crucial for user experience.
Additionally, images can sometimes cause issues such as broken links or incorrect sizing, so testing their display is essential to catch any issues early on.
- Test that the component renders with dynamic images: Test that the component renders with dynamic images is a type of React test case that ensures that the component renders with dynamic images. In this test case, we test if the component correctly displays images that are dynamically loaded at runtime. This test is important because it ensures that the component can handle images that are loaded from an external source.
- Test that the component renders with conditional images: In this test case, we want to ensure that the component correctly renders images conditionally. This can be important when we have a component that may or may not display images based on certain conditions.
To test this, we can create a mock component that conditionally renders an image based on a prop value. We can then create two test cases - one where the prop value is true and one where it is false - and ensure that the component correctly renders the image in the first case and does not render it in the second case.
Accelerate the testing speed of your React test cases. Try LambdaTest Now!
- Test that the component renders with dynamic conditional images: This React test case ensures that the component is capable of rendering images dynamically and conditionally based on specific criteria. It validates whether the component can handle changes to image sources and display the correct images based on the specified conditions.
- Test that the component renders with video: This React test case validates whether the component is capable of rendering videos. It ensures that the video player is functional and able to play videos of different formats and sizes without any errors.
When testing a component that renders with video, we want to ensure that the video element is being rendered properly, including its source URL, dimensions, and any other relevant attributes. We can also test the functionality of the video player by simulating user interactions such as playing, pausing, seeking, and changing the volume.
- Test that the component renders with dynamic video: This test case ensures that the component is capable of dynamically rendering videos based on certain criteria. It validates whether the video player can handle changes to video sources and display the correct videos based on the specified conditions.
- Test that the component renders with conditional video: This React test case ensures that the component is capable of rendering videos conditionally based on certain criteria. It validates whether the video player can display different videos based on the specified conditions.
- Test that the component renders with dynamic conditional video: Testing that a component renders with dynamic conditional video means ensuring that the component properly displays video content based on various conditions that can change dynamically.
This involves writing React test cases that cover different scenarios, such as
- Testing that the component correctly displays a video when the video URL is provided as a prop.
- Testing that the component displays an error message when an invalid video URL is provided.
- Testing that the component can handle dynamic changes in the video URL prop and updates the video accordingly.
- Testing that the component can handle changes in other props that affect the video, such as the autoplay or loop props.
- Testing that the component can handle changes in the video's playback state, such as when the video is paused or when the user seeks a different time.
- Testing that the component handles accessibility features for the video, such as providing captions or audio descriptions.
By writing test cases for these scenarios and others, developers can ensure that their component properly handles video content under a variety of dynamic conditions.
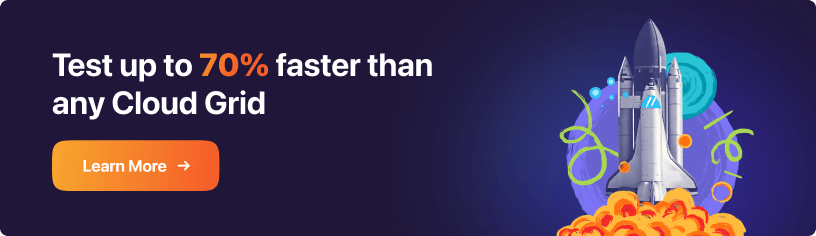
- Test that the component renders with audio: Testing audio in a React component involves checking that the component renders an audio player and that the correct audio file is loaded and played when triggered.
Additionally, we can also test for edge cases such as testing if the audio player handles errors, if it correctly pauses and resumes when expected, and if it can handle different audio formats.
Overall, testing audio in a React component is important to ensure that the component functions as expected and that it provides a good user experience for the end-user.
- Test that the component renders with dynamic audio: This React test case ensures that the component is capable of dynamically rendering audio based on certain criteria.
When testing a component that renders audio, it's important to check that the audio element is being created with the correct source and that it plays when it's supposed to.
To test dynamic audio, we can create a test that mounts the component with different props that contain different audio sources and then asserts that the audio element has the expected source and plays correctly.
- Test that the component renders with conditional audio: Testing that a component renders with conditional audio typically involves checking if the audio element is included in the component only when a certain condition is met.
For example, if the audio should only be included if the user has enabled sound, you can write a test that verifies the presence of the audio element when the sound is enabled and its absence when the sound is disabled.
- Test that the component renders with dynamic conditional audio: Testing for dynamic conditional audio involves checking if the audio component is rendered based on a certain condition and whether it plays the correct audio file dynamically based on some user input or event.
For example, if there is a button that triggers the audio to play, you would want to test that the audio file played is the correct one based on the button that was clicked.
Some things to consider when testing dynamic conditional audio components include
- Testing that the audio component is rendered based on the correct conditions
- Testing that the audio file played is the correct one based on user input or events
- Testing that the audio component behaves correctly under different conditions, such as when the audio file fails to load or when the user pauses or stops the audio playback.
Overall, testing dynamic conditional audio components helps ensure that the user experience is seamless and that the audio component behaves as expected under different scenarios.
- Test that the component renders with iframes: This React test case ensures that the component is capable of rendering iframes. It validates whether the iframe is functional and able to display the specified content without any errors. It also checks whether the component can handle changes to iframe sources and display the correct content based on the specified conditions.
- Test that the component renders with dynamic iframes: Testing iframes in React components involves verifying that the iframe element is properly rendered and that the expected content is loaded inside the iframe. This can include testing for dynamic iframes, which may have to change content or sources based on user input or other factors.
- Test that the component renders with conditional iframes: Testing components that render with conditional iframes is important to ensure that the correct iframe is rendered based on the given conditions. The tests should check that the component renders with the correct source URL, width, height, and any other relevant attributes based on the given conditions.
Some common scenarios for testing dynamic iframes include
- Testing that the correct source URL is set based on a prop or state value.
- Testing that the correct dimensions are set based on a prop or state value.
- Testing that the correct attributes are set based on a prop or state value, such as the frameborder, allowfullscreen, or allowpaymentrequest attributes.
- Testing that the iframe is not rendered at all when certain conditions are not met.
By testing these scenarios, you can ensure that your component behaves correctly in different situations and that your users can view the intended content in the iframe.
- Test that the component renders with dynamic conditional iframes: Testing the rendering of dynamic conditional iframes in a component is important to ensure that the iframe content is loaded correctly based on certain conditions. This can be achieved by setting up a test scenario where the iframe is rendered based on a conditional statement and the content of the iframe changes based on dynamic data.
Overall, testing the rendering of dynamic conditional iframes involves creating test scenarios where the visibility and content of the iframe change based on dynamic data and testing for edge cases where the iframe fails to load.
- Test that the component renders with forms: Testing forms in React components is an important part of ensuring that user input is captured accurately and efficiently. Here are some tests you can run to ensure that your form components are working as expected.
- Test that the form renders without errors. This test checks that the form component can render without any issues.
- Test that form fields render correctly. Check that each field in the form is rendered correctly and has the expected properties such as name, type, and label.
- Test that form submission works. Check that the form can be submitted successfully and that the data is sent to the correct endpoint.
- Test that validation works correctly. If your form has validation rules, test that they are correctly applied and that invalid data cannot be submitted.
- Test that form inputs can be updated. Ensure that each input field can be updated with new values and that the state is updated correctly.
- Test that form reset works. Ensure that the form can be reset and that all input fields are cleared.
- Test that form errors are displayed correctly. If the form has validation errors, test that they are displayed correctly to the user.
- Test that form success messages are displayed correctly. If the form submission is successful, test that the success message is displayed correctly to the user.
By running these tests, you can ensure that your form components are working correctly and capturing user input accurately.

Free React Test Case Template
Note : We have compiled all React test cases for you in a template format. Feel free to comment on it. Check it out now!!
- Test that the component renders with dynamic forms: This React test case checks if the component renders with dynamic forms. It verifies that the component renders the expected form fields dynamically.
Testing dynamic forms involves testing that the form fields change based on user input or other conditions, such as the presence or absence of certain data.
For example, if a form has a dropdown menu that dynamically populates with data from an API, we would test that the menu displays the correct options and updates when new data is received.
- Test that the component renders with conditional forms: This test case checks if the component renders with conditional forms. It verifies that the component renders the expected form fields conditionally based on some criteria.
Testing conditional rendering of forms is important to ensure that the form only appears when it is necessary and relevant. For example, a form to subscribe to a newsletter should only appear if the user has not subscribed yet.
- Test that the component renders with dynamic conditional forms: Testing dynamic conditional forms is an important part of ensuring that a component behaves correctly under different conditions.
Some examples of when dynamic conditional forms may be necessary include
- When certain fields are only visible or required based on the value of other fields
- When certain options are only available based on the user's permissions or other dynamic factors
- When certain fields need to be pre-filled or disabled based on data fetched from an API
- Test that the component renders with validation: This React test case checks if the component renders with validation.Testing validation in components can be important to ensure that user input is being correctly processed and validated.
- Here are some things you can test for when validating a component
- Test that required fields are being validated correctly.
- Test that validation messages are being displayed correctly when a field fails validation.
- Test that the component correctly handles different types of input (such as email, numbers, dates, etc.).
- Test that the component correctly handles input that is too long or too short.
- Test that the component correctly handles input that contains special characters or other non-standard input.
To test validation, we can create React test cases that simulate user input and check that the component behaves as expected. We can also use tools like Jest or React Testing Library to help you test your components.
- Test that the component renders with dynamic validation: Testing dynamic validation in a component ensures that the validation rules are applied correctly to the input fields and that the error messages are displayed when the input is not valid.
Testing dynamic validation can also include testing server-side validation, where the component communicates with a server to validate user input. In this case, we can mock the server response and ensure that the component correctly displays the error message or handles the successful response.
Overall, testing dynamic validation ensures that our component is user-friendly, error-free, and can handle various input scenarios.
- Test that the component renders with conditional validation: Testing conditional validation for a component involves verifying that the component displays the appropriate validation messages based on the user's input or other conditions.
For example, a form field may require a minimum number of characters, and the validation message should appear only if the user's input is below that threshold.
To test conditional validation, we can simulate different user inputs and verify that the correct validation messages are displayed or hidden. We can also test that the validation messages are displayed in the correct location and format.
- Test that the component renders with dynamic conditional validation: Dynamic conditional validation is the process of validating user input data based on certain conditions or rules that may change dynamically based on other user inputs or external factors.
To test this in a component, we can simulate user input data and verify that the validation rules are being applied correctly based on the current state of the component.
For example, you can test whether a form field that is required only when a certain checkbox is checked is being validated correctly when the checkbox is toggled on or off.
- Test that the component renders with server-side rendering: This React test case ensures that the component renders correctly on the server side. It verifies that the component's HTML and CSS markup is rendered correctly before the page is loaded.
Overall, the goal is to ensure that the component can be rendered on the server and that the resulting HTML matches the expected output. This can help catch issues related to server-side rendering, such as incorrect markup or missing data before they reach production.
- Test that the component renders with dynamic server-side rendering: Testing dynamic server-side rendering can be a bit tricky as it involves testing the behavior of the server itself, which is not easily simulated in a testing environment. However, it is important to test server-side rendering to ensure that the component is correctly rendered on the server and that the markup generated on the server matches the markup generated on the client.
Keep in mind that server-side rendering is typically done for the initial page load, and subsequent updates to the page are typically handled through client-side JavaScript. Therefore, it may not be necessary to test dynamic server-side rendering, but rather the dynamic updates that happen on the client-side.
- Test that the component renders with accessibility features: Testing for accessibility is crucial in ensuring that web components are usable by everyone, including those with disabilities. Accessibility testing includes checking for things like proper labeling of elements, correct use of ARIA attributes, and ensuring that the component can be navigated using keyboard-only input.
To test for accessibility features, you can use tools like screen readers, keyboard-only navigation, and automated accessibility testing tools like Lighthouse or Axe. Manually testing with a screen reader is especially important as it can help identify any issues that automated tools may miss.
It's important to note that accessibility is not just a testing consideration, but should also be a design and development consideration from the start of the project. By designing and developing with accessibility in mind, you can reduce the number of accessibility issues that need to be fixed during testing.
- Test that the component renders with dynamic accessibility features: Testing dynamic accessibility features involves checking that the component's accessibility properties and features are updated correctly in response to user interactions or changes to the component's state.
For example, if a button's label changes dynamically based on user input, we would want to test that the screen reader correctly announces the updated label when the button is focused. Similarly, if a form input's error message changes dynamically based on user input, we would want to test that the screen reader correctly announces the updated error message when the input is focused.
Overall, testing dynamic accessibility features is an important part of ensuring that the component is accessible to all users, regardless of their abilities or disabilities.
- Test that the component renders with conditional accessibility features: Testing conditional accessibility features of a component involves verifying that the component renders and behaves correctly for users with different abilities or accessibility requirements, based on the state of the component.
For example, the component should have appropriate ARIA labels, keyboard accessibility, and focus management.
To test the component with conditional accessibility features, we can create different React test cases that cover the different states or props of the component that affect its accessibility. We can simulate keyboard navigation and ensure that the focus is properly managed, test screen reader compatibility, and verify that the appropriate ARIA attributes are present and accurate.
We can also use automated tools like Lighthouse or axe-core to detect accessibility issues and provide recommendations for improving the component's accessibility. Additionally, we can manually test the component with assistive technologies like screen readers, magnifiers, or voice recognition software.
It is important to ensure that the component meets the accessibility requirements specified by the relevant accessibility standards, such as WCAG |.| or Section ||| of the Rehabilitation Act, to ensure that the component can be used by all users, regardless of their abilities or disabilities.
- Test that the component renders with localization: This React test case ensures that the component renders correctly with different languages and locales. It verifies that the component's text content and formatting are localized according to the user's settings.
- Test that the component renders with dynamic localization: Testing dynamic localization is an important part of ensuring that a component is ready for use in a multilingual application. Dynamic localization refers to the ability of a component to dynamically update its content based on the user's language preferences.
- Test that the component renders with conditional localization: Testing component rendering with conditional localization involves verifying that the component renders correctly based on the selected language or locale. This includes checking that text content, formatting, and date/time display is accurate for the selected language/locale.
- Test that the component renders with dynamic conditional localization: Testing a component with dynamic conditional localization involves checking that the component can display different content based on the user's locale or language preferences. This can be achieved by simulating different locale settings in the test environment and checking that the component renders the correct content.
- Test that the component renders with error handling: Testing error handling is an important aspect of testing a React component. It involves verifying that the component handles errors in the expected way and displays the appropriate error messages or fallback content when an error occurs.
Here are some examples of how to test error handling in React components
- Test that the error boundary component renders when there is an error in a child component.
- Test that the error message is displayed when there is an error in a child component.
- Test that the error boundary component gracefully handles errors and does not crash the entire application.
- Test that the error boundary component works correctly with asynchronous code and does not cause unexpected behavior.
- Test that the error boundary component logs errors to the console or sends them to a remote logging service for debugging purposes.

Free React Test Case Template
Note : We have compiled all React test cases for you in a template format. Feel free to comment on it. Check it out now!!
- Test that the component renders with dynamic error handling: This React test case ensures that the component's error-handling feature is dynamic. It verifies that the error handling changes based on different scenarios and conditions.
Dynamic error handling tests could include testing that error messages are displayed conditionally based on the type of error or the severity of the error. We can also test that error messages are displayed differently for different users or in different parts of the application.
- Test that the component renders with conditional error handling: Testing the component with conditional error handling refers to checking how the component behaves and renders when an error is thrown conditionally, based on some specific conditions.
This type of testing is important as it helps to ensure that the component behaves as expected and provides users with appropriate feedback when an error occurs.
- Test that the component renders with dynamic conditional error handling: This React test case ensures that the component's error-handling feature is both dynamic and conditional. It verifies that the error handling changes based on different scenarios and conditions.
This involves testing how the component behaves when it encounters errors that are specific to certain conditions, such as a network error or an invalid input.
Overall, testing for dynamic conditional error handling is an essential part of ensuring that your React components are robust and reliable, even when faced with unexpected errors and conditions.