Python Automation Testing With Examples
Himanshu Sheth
Posted On: December 11, 2024
128941 Views
15 Min Read
When it comes to automating front-end tests, choosing the ideal programming language becomes extremely crucial. Python is one such language that tops the list, owing to ease of use and extensive community support. Moreover, Python automation testing lets you harness the capabilities offered by popular libraries & frameworks like Selenium, pytest, and Robot, amongst others.
Using Selenium with Python helps in making the most of Selenium’s powerful browser automation capabilities and Python’s simplicity and extensibility. On the whole, Python automation testing is widely used by QAs all over, especially with Selenium WebDriver.
In this blog, we will dive deep into the nuances of Python from a front-end perspective. The learnings of this blog will be useful in leveraging Python capabilities for automating simple as well as complex front-end scenarios.
TABLE OF CONTENTS
What Is Python Automation Testing?
As the name suggests, Python automation testing is the process of using Python scripts for automating tests. It is one of the most sought-after programming languages for the automation of manual and repetitive tasks.
A simple example of Python automation testing involves leveraging the pytest framework with Selenium to automate front-end tests on an eCommerce platform.
You can verify the registration functionality of an eCommerce website and use the same session to navigate through the site and add required items to the shopping cart. By the end of this test, you will have verified the registration, login, and cart functionalities of the eCommerce website.
Lastly, you can also harness Python for automating system administration, automating emails, and data processing tasks.
Since Python is a prerequisite for automated tests, please refer to the below video which deep dives into the installation of Python.
Why Python for Automated Testing?
Now that we know why testers prefer Selenium with Python, let’s look at some of the essential reasons to choose Python for automation testing:
- Wide Range of Libraries and Frameworks: PyUnit (or unittest) is the default test framework for performing unit testing with Python. Though PyUnit is available out of the box, Python supports other popular frameworks like pytest, Behave, Robot, Lettuce, and Nose2.
- Super Easy Parallel Test Execution: Parallel testing in Selenium and Python can be used extensively for performing web browser automation tests across different combinations of browsers and platforms. Though all Selenium-supported languages support parallel test execution, it is darn easy to use with Python.
- Multi-Paradigm Programming Language: Python is a multi-paradigm programming language. Hence, there is full-fledged support for object-oriented programming and structured programming. A majority of the features in Python support functional programming and aspect-oriented programming. Python, along with Selenium can also be used for functional testing of websites and web applications.
- Dynamic Typing: The Python language uses dynamic typing and late binding (or dynamic name resolution) that binds the methods and variable names during execution. This feature comes in super-handy for Python test automation.
- Web Scraping: Web scraping with Python is the process of extracting meaningful and useful information/data from websites. It is primarily used for academic research, competitor analysis, content aggregation, and more.
- Powerful and Hassle-Free Reporting: Reporting in test automation provides greater visibility into the nuances of test execution (i.e., percentage of tests passed/failed, test environment, screenshots, etc.). Powerful reports that give the right information in a concise and understandable form can be sent to necessary stakeholders (in the team) so that they are aware of the progress on the testing front.
All of them can be used exhaustively with Selenium and Playwright frameworks for automating web browser tests.
Python also offers options like Pyre (a performant type checker for Python 3) and Mypy, which are popular static type checkers. With these checkers, Python lets you combine the power of dynamic and static typing.
Python offers a number of libraries and frameworks, namely - BeautifulSoup (bs4), Selenium, Puppeteer, and Pyppeteer that ease the task of scraping content from websites.
How to Perform Python Automation Testing?
Now that we have looked into the importance of Python automation testing, let’s get our hands dirty by running some tests. In this blog, our discussion will be mainly centered around front-testing automated testing with Python.
Subscribe to the LambdaTest YouTube Channel and stay up-to-date with more such tutorials.
Before we get started with the tests, let’s set up the virtual environment (venv) which helps in better management of the environment and dependencies.
venv plays an instrumental role in providing isolation from the packages installed in the base environment. Run the commands virtualenv venv and source venv/bin/activate on the terminal to create the virtual environment.
All the dependencies (or Python packages), like pytest, selenium, etc., required for project execution are available in the requirements.txt file.
1 2 3 4 5 6 |
pytest-selenium pytest-xdist selenium>=4.6.0 urllib3==1.26.12 requests py |
The dependencies can be installed by triggering pip install -r requirements.txt on the terminal. Selenium v4.6.0 (or above) is installed as a part of the installation procedure.
For demonstration, we would execute simple Selenium Python tests with pytest and PyUnit (or unittest) frameworks. In case you are well-versed with either of these frameworks, it is recommended to use fixtures in Python and Page Object Model in Selenium Python for improved maintenance of the tests.
The Selenium package itself doesn’t provide a testing tool/framework. Hence, we would be using Selenium with pytest and PyUnit for automating interactions with the elements on the web page.
Test Scenario:
|
Implementation (pytest framework):
Below is the test script for the above test scenario:
Code Walkthrough:
To get started, we first import the necessary modules required for the implementation of tests. Since we are using the pytest, the pytest module is also imported into the code.
The WebDriverWait class from the selenium.webdriver.support.ui module is imported so that we can use explicit waits for scenarios where WebElements are located dynamically. The expected_conditions module provides a set of pre-defined ExpectedConditions in Selenium that can be used with explicit waits.Wait
As the elements need to be located before we perform any action, we first define the element locators of the required elements. ID, Name, XPath, Link Text, Partial Link Text, etc., are some of the widely used web locators that help you find elements within the Document Object Model (DOM).
You can either use the inspect tools available natively with the web browser or a plugin (or add-on) like POM Builder that makes it easy to find the XPath/CSS Selector of WebElements.
Once the web locator is identified, the respective WebElement(s) is/are located by combining the locator with the find_element()/find_elements() method of Selenium Python. The find_element() method returns a single WebElement, whereas find_elements() returns a list of WebElements matching the locator criteria.
As stated earlier, the setup_method() is a pytest fixture that is a part of the initialization. The method is called before every test function is implemented under the said test class.
The tests being implemented can be run on Selenium installed on the local machine as well as on the online Selenium grid offered by cloud testing platforms like LambdaTest.
LambdaTest is an AI-powered test execution platform that lets you run Python automated tests at scale across different browsers and operating systems. It comes with a number of benefits, the major ones being reduced maintenance, lower costs, and accelerated test execution.
Looking to get started? Check out this guide on Selenium testing with Python on LambdaTest.
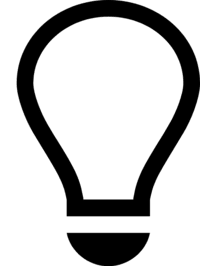
Run Python automation tests across 3000+ real environments. Try LambdaTest Today!
As far as the implementation is concerned, the only change is related to Selenium WebDriver, where Remote WebDriver in Selenium is instantiated when running tests on a cloud grid like LambdaTest. The Automation Capabilities Generator helps generate capabilities for the test combination intended to be used in the tests.
In Selenium 4, browser options are used in place of Desired Capabilities. You can check out Selenium 3 vs Selenium 4 differences blog to know more about what’s deprecated and what’s deprecated in Selenium 4.
The environment variables LT_USERNAME and LT_ACCESS_KEY can be obtained from your LambdaTest Account Settings > Password & Security. The combination, when passed along with the LambdaTest grid URL, helps in executing tests on the LambdaTest cloud grid.
In the test_enter_form_details() method, we first navigate to the test URL by invoking the driver.get() method. Next the instantiated browser window is maximized since it is considered as one of the best practices of Selenium.
Next, the Input Form Submit element is located using the find_element() method along with the XPath locator in Selenium. Once located, the button click in Selenium is invoked to simulate click action on the button element located in the earlier step.
Since all the test steps involve locating elements and performing actions, we focus on only a few methods. Shown below is the approach used for locating the company element via the CSS Selector in Selenium. The send_keys() in Selenium WebDriver helps in sending text input in the located element.
Dropdowns are widely used in websites, hence automating interactions with dropdowns using Selenium becomes an absolute must for your test cases. The Select of the selenium.webdriver.support.ui module provides methods that let you handle dropdowns with Selenium.
Here, an object (i.e., country_dropdown) of the Select class is created with the input set to the dropdown WebElement located with XPath. The select_by_visible_text() method of the Select class helps select items with visible text matching the given string (i.e., United States).
Once all the information in the form is entered and the Submit button is clicked, we wait till the success message string (default: hidden) is visible on the page. An explicit wait with the ExpectedCondition (i.e., presence of located element) is performed till the success message is not present on the page.
An assert is raised if the resultant string is not present on the page. For execution on the LambdaTest cloud, the lambda-status variable is marked as passed/failed depending on the status of execution.
The teardown_method() fixture contains implementation for cleaning up resources or state after execution of the tests in the class. The if __name__ == "__main__": construct ensures that code execution is performed only when the script is run directly. The pytest.main() calls the pytest framework that further discovers and executes all the enabled (i.e., not marked as skipped) tests in the script.
Test Execution (pytest framework):
After setting EXEC_PLATFORM to cloud, invoke the following command on the terminal to run pytest tests on the LambdaTest cloud grid:
1 |
pytest --verbose --capture=no tests/pytest/pytest_selenium_demo.py |
Shown below is the LambdaTest Web Automation dashboard screenshot that indicates that the test execution was successful:
Implementation (PyUnit framework):
The test script for the above-mentioned test scenario using the PyUnit framework is located in the tests/pyunit/pyunit_selenium_demo.py
The core logic of the test remains unchanged when migrating from pytest to PyUnit (or unittest) framework. Instead of the pytest module, the unittest module is imported into the code. The test case class is inherited from the unittest.TestCase informs the unittest module that this is a test case.
pytest fixtures setup_method()/teardown() are similar to the setUp()/tearDown() methods of the PyUnit framework. The setUp() and tearDown() methods consist of implementation whose responsibility is initialization and deinitialization, respectively.
Shown below is the boilerplate code to execute the test suite:
1 2 |
if __name__ == "__main__": unittest.main() |
Test Execution (PyUnit framework):
After setting EXEC_PLATFORM to cloud, invoke the following command on the terminal to run PyUnit tests on the LambdaTest cloud grid:
1 |
python tests/pyunit/pyunit_selenium_demo.py |
Shown below is the LambdaTest Web Automation dashboard screenshot that indicates that the test execution was successful:
In case you want to run the above tests on Selenium installed on the local machine, simply set EXEC_PLATFORM to local and you are all set with the local execution.
Top Python Testing Frameworks
Since Python supports a number of test automation frameworks, choosing the right framework becomes extremely crucial for your project. The choice actually lays the foundation for efficient testing.
Apart from the framework’s capabilities, you also need to have a look at the internal expertise with the said framework.
Shown below are some of the best Python testing frameworks:
- PyUnit (unittest): It is the default framework available in Python. As the name suggests, it is primarily used for unit testing. PyUnit is inspired by the JUnit framework for Java and shares a similar structure and functionality.
- pytest: It is one of the most popular test automation frameworks for Python. It uses a less verbose and user-friendly syntax for implementing tests. pytest can be leveraged for implementing unit tests as well as complex functional tests for testing websites and web applications.
- Robot: It is a keyword driven open-source Python framework that is majorly used for Robot Process Automation (RPA) and test automation. Akin to the pytest framework, Robot is also extensible. The usage of human-readable syntax/keywords minimizes the learning curve involved in learning Robot.
- Nose2: It is the successor to Nose and is a Python testing framework that extends the capabilities of the PyUnit (or unittest) framework. It is relatively easy to get started with Nose2 if you have prior working experience with unittest.
- Behave: It is a Python framework that is used for Behavior-Driven Development (BDD). The tests are based on the Gherkin syntax that is based on the Given-When-Then format.
The unittest framework also supports test automation sharing of setup and shutdown code for tests, independence of the tests from the reporting framework, and more. It also supports test suites and test cases that too in an object-oriented way. It also has a test runner which is a component that is responsible for orchestrating the execution of tests.
Tests written using pytest are a lot more compact, as the framework does not require boilerplate code. pytest has built-in features that help with the auto-discovery of test modules and functions.
Tests written in Robot are saved with a .robot extension. In case you plan to use Robot for front-end tests, you can do so with the SeleniumLibrary - a web testing library for Robot Framework. The library supports an exhaustive range of keywords - Click Button, Click Image, Open Browser, Drag and Drop, among others.
The major upside of Nose2 over PyUnit is the availability of a large number of built-in Nose plugins that make testing easier and faster. Plugins in Nose2 help in test parameterization, better organization of tests, fixtures support, test discovery, and more.
Since the tests are implemented in scenario and feature files, even non-technical personnel can be a part of the QA process. SpecFlow (C#) and Cucumber (Java, JS, Ruby) are some of the other popular BDD frameworks.
Conclusion
As seen so far, Python is by far the best scripting language for test automation. It is relatively easy to get started with Python automation testing. Its wide range of test frameworks can be used for unit testing, cross-browser testing, and more.
Let us know below your preferred programming language for Selenium testing and how you rate it against Python, the undisputed king for automation testing.
Happy testing!
Frequently Asked Questions (FAQs)
Is Python good for automation testing?
Yes! Python is good for automation testing due to its simplicity, vast library support, and frameworks like Selenium, pytest, and Robot Framework make it a favorite among testers.
What is automated testing in Python?
Automated testing in Python involves using testing tools to automate repetitive test cases efficiently, ensuring faster and more accurate validation of software functionalities.
Which tool is used for Python automation testing?
Selenium is a go-to for web automation, while pytest excels in functional testing; plus, tools like Robot Framework offer keyword-driven testing flexibility.
Got Questions? Drop them on LambdaTest Community. Visit now