How To Handle Authentication PopUps in Selenium WebDriver
Vipul Gupta
Posted On: June 13, 2024
217363 Views
20 Min Read
Handling authentication popups in Selenium is a crucial aspect of automated testing. Authentication popups often appear when accessing secure areas of a web application, requiring valid credentials to proceed. These popups can disrupt the flow of automated tests if not properly managed, leading to incomplete or failed test executions.
Effective handling of these popups ensures that the test scripts can seamlessly interact with the application, allowing for comprehensive testing of protected resources and functionalities. Selenium WebDriver provides robust capabilities to interact with web browsers, helping you handle authentication popups by allowing you to input credentials programmatically.
TABLE OF CONTENTS
What Is an Alert/Popup?
Alerts are small modal windows displayed within a web browser. They grab user attention for important messages, often requiring confirmation (e.g., “Are you sure you want to delete?”).
Popups are separate windows or tabs that open on top of the main browser window. They can be triggered by user actions or scripts and are used for various purposes, including displaying information, advertisements, or login forms.
Alerts/popups typically require user confirmation. While popups offer more flexibility for displaying information or collecting user input, alerts are used for critical messages requiring immediate user attention.
Below is the screenshot of the authentication popup prompted on the website.
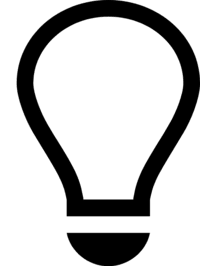
Run tests across 3000+ browsers and OS combinations.Try LambdaTest Today!
Types of Alerts/Popups
There are several different types of alerts/popups, like simple, confirmation, prompt, and authentication alerts, one might encounter on a website or web application. These are used for different purposes, and their handling might vary slightly on the implementation basis.
Before we move forward and learn how to handle authentication popups in Selenium WebDriver, let us quickly look at the different types.
Simple Alerts/Popups
These are used to display simple messages to the user, which can be any information, error, or warning. This type has only the message and a Cancel or an OK button.
Some real-time examples are reminder messages, invalid credentials error messages, device memory/battery low warning notifications, etc.
Confirmation Alerts/Popups
As the name suggests, these are used to take user confirmation on any action. These popups have two buttons, one to accept/provide confirmation and the other to cancel/deny.
Examples of this type include asking for cookie permission on a website, an alert for location/microphone access, a confirmation alert when you try to close it with active audio/video in play, etc.
Prompt Alerts/Popups
These types of alert popups notify users of the input or ask them to enter some data. These tend to have a message, some input fields, and OK/Cancel buttons, depending on the use case.
Some examples include a prompt to enter a password or name, an alert to enter valid/mandatory data, a prompt to enter details of the wireless network, etc.
Authentication Alerts/Popups
This popup asks for user credentials to perform authentication or login to web applications to interact on the same.
Some real-world authentication popup examples would be an alert/popup asking for a username and password to access some protected API, to login to a VPN or your net banking, etc.
Understanding the basic authentication mechanism for handling authentication popups in Selenium WebDriver is crucial for ensuring the security of your web application.
HTTP Basic Authentication
HTTP authentication is a simple security mechanism that restricts access of ineligible users to selected and protected web resources. It involves communication between the server and client using HTTP headers to provide user credentials to establish authenticity and provide access to the user.
One of the most used HTTP authentication methods is the HTTP Basic Authentication. In this, when a user attempts to access a protected web resource, the server prompts with an authentication popup asking for user credentials. These credentials are then used to establish a connection, once the user identity is authenticated at the server, the user can access the resources.
- The client requests to access the server anonymously without providing any credentials.
- The server responds with 401 Unauthorized, asking for user credentials.
- The client responds with its username and password to authenticate itself.
- The server tries to verify the username and password. If valid, access is granted. If invalid, the server responds with 403 Forbidden.
Handling Authentication Popups in Selenium WebDriver
Having understood the basics of HTTP authentication, let us move forward to learn how to handle authentication popups in Selenium WebDriver.
Selenium WebDriver is one of the key components of the Selenium suite, which is used to automate web browsers for web applications’ automated testing. It supports cross-browser testing by providing a programming interface to interact with various real browsers like Chrome, Firefox, etc., using browser drivers like ChromeDriver, GeckoDriver, and more.
To handle authentication popups in Selenium WebDriver, we will use this demo test website: Herokuapp Basic Auth. Navigating this gives us an alert/popup like the one below.
We will learn how to handle authentication popups in Selenium WebDriver using the following approaches:
- Passing the Username and Password in the URL
- Using AutoIT
- Using Selenium 4 and Chrome DevTools
To learn more about handling authentication popups in Selenium WebDriver, watch the following video tutorial and get practical insights.
Subscribe to the LambdaTest YouTube Channel and stay updated on other automation frameworks like Playwright, Cypress, Appium, and more.
Handling Authentication Popups by Passing the Username and Password in the URL
The basic authentication popup is similar to the alert that pops up when the browser is navigated to a specific web page. To handle the basic authentication popup, you can pass the username and password along with the web page’s URL.
The syntax for handling this authentication popup is:
1 |
https://username:password@URL |
You enter the username as “admin” and the password as “admin”, followed by the URL, and then log in. Thus, the user would be successfully logged into the website without providing any login credentials for authentication.
Once the credentials are passed correctly, the user will be authenticated successfully, and a proper message will be displayed on the website.
Let us set up the project before moving to the code for this method of handling authentication popups in Selenium WebDriver.
Before setting up the project, we will first define the prerequisites. For demonstration purposes, we will use Eclipse IDE, Selenium with Java, and TestNG as the testing framework for running the tests.
Project Setup:
First, create a Maven project named HandlingLoginPopup using Eclipse IDE and then update the pom.xml file with the latest version of Selenium and TestNG.
Once you update the pom.xml file, it will look as shown below.
Next, create a Java class file and name it BaseTest.java. This file will have the common code to launch and close the WebDriver instance before/after the test execution. All the following test case files will extend this base file and its entities.
The Java class file will look as shown below.
Before writing test scripts, we will understand the code in the BaseTest.java file below.
Code Walkthrough:
- Create an instance of WebDriver, which will be used to perform browser interactions.
- Define the username and password of the user to authenticate the user on the test website.
- Add the first method as setDriver(). This will be used to instantiate the driver session. Annotate this with @BeforeClass annotation to execute it before each test case.
- Add tearDown() as another method and annotate it with @AfterClass to close the browser and end the WebDriver session after each execution.
To learn about other annotations offered by TestNG, follow this guide on TestNG annotations. It will help you understand how to prioritize and run your tests from most to least important.
Now that we have set up the project with all the required prerequisites, we will begin to write code for the first method of passing the username and password in the web page URL.
To begin, create a Java class file named TestHandlingLoginPopUpUsingCredentials. This file will contain the code that enters the username and password into the web page URL and retrieves the text once successfully logged in.
Let us understand the code given in the TestHandlingLoginPopUpUsingCredentials file in detail below.
Code Walkthrough:
- Start by extending BaseTest in this test case file. This will help to inherit the driver and user credentials, which will be used further.
- Add the test case as testHandlingLoginPopUpUsingCredentials() and annotate it with @Test, as we are using the TestNG for test execution.
- Create the web page URL using username and password in the given format and navigate to the same using the get() function of Selenium WebDriver.
- Fetch and print the page title and successful authentication message on the console.
- Download AutoIT from the official website or use the AutoIT download link.
- Navigate to the installed folder and open the AutoIT editor.
- Enter the below code in the editor to send the username and password to handle the authentication popup in Selenium WebDriver on the web page.
- Save the file with the extension as Login.au3.
- Convert the script to an executable file by right-clicking on the saved file and clicking Compile Script. You will see an executable file created with the name Login.exe.
- Move to your IDE and add the test case file to write the test to handle the authentication popup in Selenium WebDriver using the AutoIT executable file you just created.
- Add a test class as TestHandlingLoginPopUpUsingAutoIT and extend BaseTest in it.
- Inside the test case, we start by defining the test URL and navigating to it.
- Define a timeout of 10 seconds to allow the page to load and the authentication popup to be visible.
- Use the exec() function of the Runtime class to execute the Login.exe you created using AutoIt in previous steps. Remember to define the path of the .exe file properly for execution.
- Add a Thread.sleep() for the script execution to complete and the authentication popup in Selenium WebDriver to disappear.
- Fetch and print the page title and successful authentication message on the console to confirm that the authentication popup in Selenium WebDriver was handled successfully.
- Add a new test case as testHandlingLoginPopUpUsingDevTools() and annotate it with @Test annotation.
- Create a driver instance of the ChromeDriver class. This is because ChromeDevTools works only for Chromium-based browsers like Google Chrome. Another browser driver is EdgeDriver, also available if you want to use the Edge browser.
- Get the DevTools and create a new session to be used.
- Enable the network domain of DevTools. We don’t want to specify any network, so we have used the empty() method.
- Assign the username and password concatenated by a colon (:) to a String variable. Encode this value to get an encoded Base64 credentials string.
- Finally, pass the encoded credentials as basic authorization network header to the DevTools by creating headers in a HashMap.
- Now that the entire code for handling the authentication popup in Selenium WebDriver and navigating to the website.
- You can see there is no authentication popup now, as credentials have already been passed in the headers. Verify the same by fetching and printing the page title and successful authentication message from the webpage body.
- Create an instance of Selenium RemoteWebDriver and initialize it to null.
- Add a variable status and initialize with the value failed. This will be used to update the test status on the LambdaTest dashboard to pass/fail.
- Add the LambdaTest Username and Access Key for your account to be used to run the script. You can find these details in the LambdaTest Profile section by navigating to Account Settings > Password & Security.
- Add the first method as setup() and annotate it with @BeforeTest annotation to execute it before each test for driver setup and connecting to the LambdaTest cloud grid.
- Within this function, create an object of the ChromeOptions class, which defines browser properties like browser version, OS version, etc.
- Next, define some additional variables for test execution on LambdaTest. These variables are used to set values like build, name, or other browser property/behavior. This is done with the help of a HashMap variable, which is then passed to chromeOptions.
- Finally, create an instance of RemoteWebDriver to connect to the LambdaTest cloud grid using all these properties.
- Add another method as closeDriver() and annotate it with @AfterTest to execute after each test execution. This method updates the test status on the LambdaTest dashboard and terminates the browser and driver session.
- Create a test class file TestHandlingLoginPopUpUsingCloudGrid, and inherit the BaseTest.
- Add the test case and annotate it with @Test annotation to execute it as the TestNG test case.
- Set the credentials to authenticate the user and navigate to the test web page URL as you did earlier.
- Fetch the page title and successful authentication message and print on the console.
- Set the status as passed to update the LambdaTest dashboard results.
- Video Recordings: Watch replays of your test execution to visualize each step.
- Automation Script Steps: Review the automation script steps taken during the test.
- Network and Console Logs: Analyze network traffic and console messages for deeper troubleshooting
- Navigate to Analytics: In the LambdaTest platform, locate the Insights section and select Analytics.
- View Test Results: The dashboard will display key metrics like the number of passed and failed tests. Additionally, you’ll see data trends to help you analyze test runs and compare results over time.
Output:
When executing the test case with TestNG, the test will handle the authentication popup and prevent it from displaying, resulting in an output similar to the one below.
Result:
Having learned the first method of handling authentication popups in Selenium WebDriver by passing the username and password in the URL, we will now learn the second approach using AutoIT with a code demonstration.
Handling Authentication Popups by Using AutoIT
AutoIT is a common scripting language for automating GUI programs and tasks involving keyboard and mouse interactions. It is frequently used to automate processes such as handling authentication popups and downloading and uploading files.
Let’s start by setting up AutoIT and see how it can handle the authentication popup on the web page.
To understand how the code works in the TestHandlingLoginPopUpUsingAutoIT file, we will go through a code walkthrough below.
Code Walkthrough:
It is advisable to use Selenium Waits, such as implicit, explicit, and fluent methods offered by Selenium rather than Thread.sleep(), as it has drawbacks.
Output:
Executing the above script will give an output like the one below.
Both the approaches to handling authentication popups in Selenium WebDriver you have learned so far are older and do not have much Selenium usage. To get to an advanced level, let us learn how to handle authentication popups in Selenium 4 and Chrome DevTools.
Handling Authentication Popups in Selenium 4 and Chrome Dev Tools
Selenium 4 introduced the ChromiumDriver class, which has two methods, namely getDevTools() and executeCdpCommand(), to access the Chrome DevTools.
The getDevTools() method returns the new DevTools object, which allows the user to send the built-in Selenium commands.
In previous versions of Selenium, we used the technique of passing the credentials in the URL of the web page to handle the basic authentication popup on the web page. Now, in Selenium 4, we can easily set the basic authentication by sending extra HTTP headers; thus, handling the login authentication popups in Selenium becomes easy.
Below is the final test case file with Selenium 4 and DevTools usage to handle authentication popups in Selenium WebDriver.
To understand the code given in the TestHandlingLoginPopUpUsingDevTools.java file, let’s look into the code walkthrough below.
Code Walkthrough:
Output:
When executing the above code to handle authentication popups in Selenium 4 and Chrome DevTools, you will get an output like the one below.
With this, we have seen different ways to handle authentication popups in Selenium WebDriver with Java. All the methods we learned use local execution.
Local execution comes with challenges, such as scaling, using varied browser and OS combinations, speed of execution, and debugging. However, using cloud testing platforms like LambdaTest can overcome these challenges.
LambdaTest is an AI-powered test orchestration and execution platform that allows you to perform manual and automation testing at scale over 3000+ real devices, browsers, and OS combinations. This provides the speed and resources needed to execute more test cases in parallel and in a more organized manner.
Handling Authentication Popups on Cloud Selenium Grid
As we have learned some approaches for handling authentication popups in Selenium WebDriver, implementing them on the cloud will require modifying the existing code.
Let’s see how easy it is to implement the code over the LambdaTest platform to run tests using RemoteWebDriver.
For this first create a base class file, BaseTest.java. This will have the code to initiate the browser session for connecting to the LambdaTest platform, handle the code for browser setup, and close it after test completion.
The test class will inherit this code, thus preventing redundancy and providing an abstraction of driver code from every other test class user.
Below is the code walkthrough for the code in the BaseTest.java file
Code Walkthrough:
LambdaTest provides a way to easily retrieve these properties and values using the LambdaTest Capabilities Generator. You only must select the required operating system, browser combination, and versions. The rest is done for you, and you have the code ready.
We are ready with the required configuration to set up and connect with the LambdaTest cloud grid in the BaseTest.file.
We will now have to create a test file named TestHandlingLoginPopUpUsingCloudGrid.java. This file consists of the same code used for the first method, which is handling authentication popups by passing the username and password in the web page URL.
Below is the detailed code walkthrough to help you understand how each code instruction works.
Code Walkthrough:
Output:
Executing the above test case will give output like the one below on the console.
Result:
Since you run your tests on the LambdaTest platform, you can easily access detailed test logs on the LambdaTest Web Automation Dashboard. These logs include:
Your executed test cases are under Automation > Web Automation on the Web Automation Dashboard, as shown below.
To see the detailed execution for your case, click on the test case, and you can see the details like the following:
In addition to detailed logs, you can gain valuable insights into your test performance using the LambdaTest Analytics Dashboard.
Here’s how to access it:
Wrap Up
To summarize, we have seen different ways of handling authentication popups in Selenium WebDriver using Java on both the local and cloud grid. We gained an understanding of how to do the same in your automation scripts, depending on the use case. It’s time for you now to go ahead and handle these authentication popups. Happy Testing…! 😊
Frequently Asked Questions (FAQs)
How do I stop popups in Selenium?
Firstly, you need to check for the active window in your application (Popup or main window). If the popup is the active window, you can use the driver.close() or a window handler to close the popup if the main window is active.
How to handle login popups that are not alerts?
If the login popup is not an alert but part of the web page, it must be handled differently. Using WebDriver’s findElement() method, the username and password fields and submit buttons can be located and interacted with accordingly.
How to handle login popups that appear in iframes?
If the login popup appears inside an iframe, we must first switch to the iframe to handle the same. For this, switchTo().frame() method of Selenium can be used. Once you are on the required frame, handle the login popup using the findElement() method to locate the WebElements and interact with them.
Can I handle basic authentication popups using WebDriver’s Alert interface?
No, WebDriver’s Alert interface is not applicable for handling basic authentication popups as they are not JavaScript alerts. To handle basic authentication popups, you can pass the credentials in the URL, use an AutoIT script or Chrome DevTools with Selenium 4.
Is it safe to pass credentials in plain text in URL when handling authentication login popups?
Embedding credentials in the URL is the most commonly used approach to handle basic authentication popups. They expose credentials in plain text, so we need to use a secure method to store and manage credentials to prevent any security breach.
Got Questions? Drop them on LambdaTest Community. Visit now