How to Handle Hidden Elements in Selenium WebDriver
Sri Priya
Posted On: June 12, 2024
183829 Views
10 Min Read
When running automated tests with Selenium WebDriver, testers might encounter ElementNotInteractableException or ElementNotVisibleException if the web page elements are hidden or can’t be interacted with.
This happens because the elements exist in the Document Object Model (DOM) but aren’t visible on the web page. In such cases, testers can use JavaScriptExecuter to handle hidden elements in Selenium WebDriver.
TABLE OF CONTENTS
What Are Hidden Elements?
Hidden elements are the ones that are present in the DOM but not visible on the web page. Usually, hidden elements are defined by the CSS property style=display:none.
In case an element is a part of the < form > tag, it can be hidden by setting the attribute type to the value hidden.
Hiding the elements can be done using the following ways:
- Using the style attribute and display:none value.
- Using a block value for the display property within the style attribute.
- Using a hidden value for visibility property.
- Giving a hidden value to the type attribute in the HTML code.
What is DOM Implementation of Hidden Elements?
If an element is only hidden until a specific action, such as clicking a link or button, it can be displayed on the web page after the action is performed.
I have created the below web page for the demo of hidden elements:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 |
<!DOCTYPE html> <html> <head> <meta name="viewport" content="width=device-width, initial-scale=1"> <link rel="stylesheet" href= "https://cdnjs.cloudflare.com/ajax/libs/font-awesome/4.7.0/css/font-awesome.min.css"> <script> function showme(id) { var lmd = document.getElementById(id); var LMT = document.getElementById("lmt"); if (lmd.style.display == 'block') { lmd.style.display = 'none'; LMT.innerHTML = 'LMT <i class="I am Hidden "></i>'; } else { lmd.style.display = 'block'; LambdaTest.innerHTML = 'Tag-line <i class="I am Hidden "></i>'; } } </script> </head> <body> <center> <div onclick="showme('widget');" id="geeks"> <h1 style="color:blue"> <button type="button">Click Me!</button> </h1> <i class="I am Hidden "></i> </div> <div id="widget" style="display:none;"> <h3> <div onclick="showme('widget');" id="geeks"> <h1 style="color:blue"> <button type="button">Hide Me!</button> </h1> <i class="I am Hidden "></i> </div> I am Hidden, Click on Hide Me! </h3> </div> </center> </body> </html> |
Here, I have used style.display = ‘block’; and style=”display:none;” for hiding the Hide Me button.
Output (before clicking on the Click Me! button):
Output (after clicking on the Click Me! button):
Since these elements are hidden, they are not visible to Selenium WebDriver.
From the above output, there are two buttons: Click Me! and Hide Me! Here, we do the following checks:
- Check if the Click Me! button is displayed.
- Click on the Click Me! button and the Hide Me! button will be displayed.
- Click on the Hide Me! button and the text and Hide Me! button will be hidden.
While handling hidden elements, you can enhance your Selenium testing process with AI-driven test agents like KaneAI.
KaneAI by LambdaTest is an innovative AI test assistant built for fast-paced quality engineering teams, offering unique AI-powered features for test authoring, management, and debugging. It allows users to easily create and update complex test cases using natural language, making it much easier and faster to get started with test automation without needing deep expertise.
Can Selenium WebDriver Interact With Hidden Elements?
Now you have a gist of the DOM implementation of hidden elements, let’s look at how to handle hidden elements in Selenium WebDriver.
To check if Selenium WebDriver can interact with hidden elements, I will use my portfolio website.
For this, we will use JavaScriptExecutor to handle the hidden elements in Selenium WebDriver. The attribute of the hidden element is changed, making the element visible using visibility:visible.
Subscribe to the LambdaTest YouTube Channel for quick updates on the tutorials on Selenium testing, Selenium Java, and more.
Let’s take a scenario – there are two buttons, Hide and Show. The Hide and Show button and the text box appear when the page is loaded. Once we click on the Hide button, the text box will be hidden. Again, the text box will appear when we click the Show button.
Implementation:
Selenium does not allow us to perform any action on the hidden elements on the web page. In the above case, I clicked on the Hide button, and then I tried entering the text LambdaTest in the text field, so it threw ElementNotInteractableException.
So, how do we interact with the hidden element so that we do not encounter any exceptions? Let’s look into how to handle hidden elements in Selenium WebDriver.
Demonstration: Handle Hidden Elements in Selenium WebDriver
We will use JavaScriptExecutor, tweaking the display:none; for the style attribute to visibility:visible; so that we can make the hidden element visible to the Selenium script.
We can change the display:none value of the style attribute to visibility:visible at run time while running the tests.
Changing the style attribute will change the style of the page, but it will make it visible to the Selenium scripts. Here, we again use the execute_script() method to execute the script.
1 |
driver.execute_script("arguments[0].setAttribute('style','visibility:visible;');",element) |
We will be using the getElementById() method of JavaScript and pass the ID as in the below syntax:
1 2 |
JavascriptExecutor jse=(JavascriptExecutor) driver; jse.executeScript("document.getElementById('displayed-text').value='LambdaTest';"); |
We often will not get the elements with unique IDs or names for them. In that case, we can use XPath and use it in the JavaScriptExecutor methods as below:
We use arguments[0].click() as it holds the web element in the first line of code, which is hidden on the web page.
Apart from the direct use of JavaScriptExecutor, we also have another way to make the element unhidden for the Selenium tests. As we discussed, we use CSS to hide the element. We can tweak the identical CSS to unhide the element as well.
We have used the display:none property for the style attribute to hide this element.
We can change the display:none value of the style attribute to visibility:visible while running the Selenium test.
Changing the style attribute will change the CSS of the text field, and the element will be visible to the Selenium tests at run time.
Here, we will also use JavaScriptExecutor and the setAttribute() method to make the element unhidden or not hidden. We use the below code to make it.
1 2 |
WebElement element=driver.findElement(By.xpath("//input[@id='displayed-text']")); ((JavascriptExecutor)driver).executeScript("arguments[0].setAttribute('style','visibility:visible;');",element); |
Next, we will run tests to handle hidden elements in Selenium WebDriver. Testing on a cloud grid is feasible to achieve better scalability and reliability. For this, we leverage cloud-based testing platforms such as LambdaTest.
It is an AI-powered test orchestration and execution platform that allows you to perform automation testing with Selenium WebDriver on a remote test lab of different browsers and operating systems.
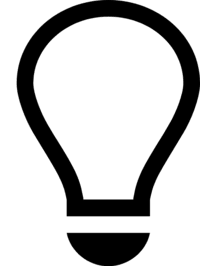
Automate Selenium Java Tests Across 3000+ Real Browsers and OS. Try LambdaTest Today!
Implementation:
Code Walkthrough:
The below snap shows the structure of our web project.
Import packages using JavaScriptExecutor and other methods.
Create the RemoteWebDriver class that implements the WebDriver interface to execute the tests on the Remote WebDriver server on a remote machine. It is implemented under the package below:
@BeforeMethod annotation in TestNG sets the browser’s capabilities. A RemoteWebDriver instance is created with the desired browser capabilities, with the Selenium Grid URL ([@hub.lambdatest.com/wd/hub]) set to the cloud-based Selenium Grid on LambdaTest.
All the test navigation steps are implemented under the @Test annotation. Here is how we navigate to the desired URL:
Maximize the window and wait for the page to load using the explicit wait in Selenium. Selenium WebDriver must wait until the specified condition occurs before executing the code.
Use the maximize() method to maximize the window in Selenium:
To use explicit wait, use ExpectedConditions in Selenium.
Initialize the wait object using the Selenium WebDriverWait class.
Create an object of JavaScriptExecutor and pass the element to the script to interact with the element to send text.
The executeScript() method executes the test script in the context of the currently selected window or frame.
Send the text through JavascriptExecutor. The document.getElementById() method returns the element of the specified id. The document refers to a web page that is the Document Object Model of the web page. If we want to access any element in an HTML page, we need to access the document object.
Close the driver instance using the quit() method.
Test Execution:
Once you run the test, navigate to the LambdaTest Web Automation Dashboard to view your test results.
Final Thoughts!
In this blog, we learned how to handle hidden elements in Selenium WebDriver.
To summarize, hidden elements are HTML elements not visible to the user when a web page is displayed in a web browser. They can be created using the hidden attribute in HTML or CSS to set the element’s display property to none.
When performing Java automation testing on hidden elements, it is important to ensure that the elements are accessible to the test script and that interacting with them does not cause any unexpected behavior in the web application.
Frequently Asked Questions (FAQs)
What are the hidden elements in Selenium?
Hidden elements in Selenium typically have CSS properties like display: none or visibility: hidden, making them not visible on the web page.
How to show hidden elements in inspect?
To show hidden elements in the inspect tool, you can manipulate the CSS properties of the element to change its display or visibility settings.
What to do if the element is not visible in Selenium?
If an element is not visible in Selenium, you can try using actions like scrolling, resizing the window, or switching to an iframe context to interact with it.
How to validate invisible elements in Selenium?
To validate an invisible element in Selenium, you can check its attributes or properties using methods like is_displayed(), get_attribute(‘style’), or execute_script() to inspect its visibility status.
Got Questions? Drop them on LambdaTest Community. Visit now