Learn To Close Browser Tabs In Selenium Python And Java
Tanay Kumar Deo
Posted On: March 21, 2024
268173 Views
21 Min Read
If you have landed here, you must be familiar with the basics of the automation testing framework, Selenium. Selenium automation offers various methods to mimic user interaction with testing scripts. From unit testing to functional testing, there is no limit. Testers and Developers often involve opening and closing multiple tabs while performing testing and mining of websites.
In this article, we will learn all the techniques for Selenium Close Tab in both Python and Java. With that, we will also explore some methods used to close current or multiple tabs in Selenium and how to handle alerts and pop ups while closing the browser tab. If you are preparing for an interview you can learn more through Selenium interview questions.
TABLE OF CONTENTS
- When Do We Need To Close Tab In Selenium Automation?
- The close() and quit() methods in Selenium WebDriver
- Close Current Tab in Selenium
- Close All Tabs Except the Current One
- Selenium Close Tab using Browser Shortcuts
- Selenium Close Tab using JavascriptExecutor
- Handling Alerts during Selenium Close Tab
- Selenium Close Tab on a Cloud-Based Testing Platform
- Frequently Asked Questions
When Do We Need To Close Tab In Selenium Automation?
Opening and closing a new tab is one of the most basic functionalities needed for automation testing. Closing unnecessary tabs helps improve testing efficiency and speed by freeing up resources. To learn more about it, let’s look at some scenarios when closing tabs is handy during Selenium automation.
- Testing Ecommerce App Feature: When testing or gathering information from a large and complex web application like e-commerce, it becomes crucial to be mindful of our resources. For example, if we are trying to scrape information on multiple products, which opens up in a new tab by default, we may use these separate tabs to extract information. Once we have pulled the needed information, it’s a good idea to close the tabs to free up memory and processing power for other tests.
- Multimedia Uploading Functionality Testing: Another typical example is when we test the scalability of platforms like YouTube for handling multiple coincidental/parallel uploads made through various tabs, browsers, and systems. This is an excellent case for cross platform and cross browser testing on multiple tabs.
- Troubleshoot Errors While Performing Parallel Testing: In some scenarios where we perform parallel testing to test multiple features simultaneously on different tabs, browsers, and devices, it becomes tough to troubleshoot the errors if we have many tabs open. To prevent this scenario, we must regularly close tabs using Selenium.
In short, automation testing involves closing tabs to free up resources and speed up the testing process. This article will cover all the methods and techniques needed to close the browser tab in Selenium Using Python and Java with practical examples.
To further enhance your test automation, KaneAI, offered by LambdaTest, can assist in optimizing these processes. KaneAI analyzes your Selenium tests, helping you manage browser tabs more efficiently by identifying resource-heavy tabs and suggesting when to close them.
This AI-powered assistant reduces memory usage and improves the overall performance of your tests across different browsers and tabs, ensuring smoother and faster test executions.
The close() and quit() methods in Selenium WebDriver
In Selenium WebDriver, there are two methods to handle closing a browser window (tab) or completing a session, close() and quit(). These functions are usually used interchangeably, but both have different functions.
Note: In Selenium automation, we usually refer to a browser tab as a browser window, and a browser session means the complete browser.
close()
The close() method is an in-built Selenium WebDriver method that closes the browser window in focus. It is always recommended to use the close() method when multiple browser windows or tabs are open. If only one tab is open in the entire browser session, the close() method will also quit the complete browser session.
quit()
The quit() method simply quits the browser as the whole session with all its tabs or windows. This method is mainly used when the developer or tester wants to end the program. It is always recommended to call the quit() function at the end of the program; otherwise, the Selenium WebDriver session will not close correctly and may lead to memory leaks, as assets will not be wiped off memory.
You can also subscribe to the LambdaTest YouTube Channel for the latest updates on tutorials around Selenium testing, and more.
Close Current Tab in Selenium
In this section, we will explore a real example of how to close a current tab in Selenium using the close() method. We will be covering this example in both Python and Java separately.
Close Current Tab in Selenium using Python
To get started with Python Selenium close tab automation, our system needs to be equipped with all the required tools. Listed below are the necessary prerequisites:
- Python version 3.7 or above
- Latest version of Selenium (version 4.6 or above recommended)
- IDE or Code Editor like VS Code
Although in this article we will be using the Google Chrome browser, you can choose any other browser and just need to call the respective WebDriver (like: webdriver.Edge(), edgedriver.Firefox()); the rest of the code will remain the same. Once the setup is complete, we can proceed with the example of how to close a current tab in Selenium using Python.
In this example, we will first open a URL in a new tab, print the title of the current tab and then close the current tab using the close() method. So, let’s start with this example by creating a Python file.
In the above code, we used the close() method to close a current tab in Selenium using Python. Here, the close() method first closes the current tab in focus and then exits the browser session as no other tab is available on the browser.
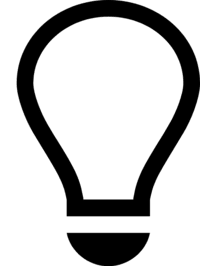
Automate your tests on a Selenium cloud grid of 3000+ real browsers. Try LambdaTest Today!
Close Current Tab in Selenium using Java
Prerequisites:
- Java SE 8 or above
- Latest version of Selenium for Java (version 4.6 or above recommended)
- Code Editor or IDE like Eclipse IDE
You may check out our blog on Selenium Automation Testing with Java to learn in depth.
Once the setup is complete, we can proceed with the example of how to close a current tab in Selenium using Java.
Similar to the above example in Python, we will first open a URL in a new tab, print the title of the current tab and then close the current tab using the close() method in Java. So, let’s get started with this example by creating a Java class.
The above code will first open a URL in a new tab, and print the title of the current tab. Then the close() method is called to close a current tab in Selenium using Java. Here, the close() method first closes the current tab in focus and then exits the browser session as no other tab is available on the browser.
Close All Tabs Except the Current One
Now that we have seen a simple example of how to close a current tab in Selenium, let’s explore a little complex example and learn to close all tabs except the current tab in focus.
In this intermediate example on Selenium close tab, we will first open multiple tabs, print their page title, and then try to close all the tabs except the current one. Here also, we will be using the close() method to close the tab and then the quit() method to end the session. This is a common practice while working with multiple tabs. Let’s look at the code for this example in Python and Java.
Close All Tabs Except the Current One using Python
Closing multiple tabs in Selenium Python is not bulky. Selenium has methods like window_handle() and current_window_handles() that add the cherry on top to automate multiple tabs closing with Python. We can use these methods to switch between tabs and then close them using the close() method. The switch_to.window() method makes the switching between tabs a cakewalk.
We can start with the example by creating a Python file and following the steps mentioned below:
- Open multiple URLs on separate new tabs using the javascript with execute_script() method of Selenium.
Print the title of each tab opened to track them. - Get the current tab using the current_window_handles method.
- Get all opened tabs listed using the window_handle method of Selenium and iterate over them.
- While iteration, if the selected tab is not equal to the current tab, switch to it and close that tab.
- At the end, switch to the current tab.
Let us break down the code better to understand Selenium Close All Tabs Except the Current One.
We first import all the needed dependent libraries. webdriver module from the Selenium Python library to automate browser actions, and the Python time module explicitly or implicitly pauses the execution or wait.
In the open_multiple_tabs_from_urls method, we use the JavascriptExecutor to open all the URLs in the urls list. It uses the execute_script(f”window.open(‘{url}’, ‘_blank’);”) method provided by Selenium to open a new tab with ease. It also prints the title of each page opened. Lastly, we added a time delay to ensure the page loads before moving to the new tab.
The close_all_except_current_tab() method is mainly responsible for closing all tabs but not the current one. To do so, it uses window_handle and current_window_handle methods offered by Selenium. The window_handles method returns the list of all open tabs, and current_window_handle returns the current tab in focus.
We then iterated over the all_tab_list to switch tabs using the switch_to.window() method and call the close() method for smooth closing of the tabs. A condition (if window != current_tab:) is checked to ensure the current tab is not closed. This function also prints the title of the closed tab in the console.
The console output produced by running this automation script is shown in the image below.
Close All Tabs Except the Current One in Java
As we already discussed, closing multiple tabs in Selenium Java is not bulky. We can proceed with the algorithm that we will be following for this example of the Selenium close tab in Java. The steps to close all tabs but not current one are mentioned below:
- Open multiple URLs on separate new tabs using the javascript with the help of JavascriptExecutor class in Selenium Java.
- Print the title of each tab opened to track them.
- Get the current tab using the getWindowHandle() method.
- Get all opened tabs list using the getWindowHandles() method that returns a set of all tabs and then iterates over the set of tabs.
- While iteration, if the selected tab is not equal to the current tab, then switch to it and close that tab.
- At the end switch back to the current tab.
Let’s start writing a Java program for our example by creating a Java class.
Let us break down the code for a better understanding of Selenium Close All Tabs Except the Current One. Here it goes:
Here, we first import all the necessary dependent Java libraries. WebDriver class from the Selenium Java library to automate browser actions. The Set and Iterator classes from Java util package to store all the browser tabs and iterate over them. Lastly, the JavascriptExecutor class to execute JavaScript through Selenium.
The openMultipleTabs(string[] urls) is our supporting method which opens multiple tabs using the urls array. It opens multiple tabs based on the URL list passed. It uses the JavascriptExecutor class to execute the “window.open(‘” + urls[i] + “‘, ‘_blank’)” JavaScript command to open a new tab with ease. This function also prints the title of the opened page. Lastly, after opening every tab, it uses Thread.sleep() to add a delay for loading the page before proceeding with the next tab.
The closeMultipleTabs() function is mainly responsible for closing all tabs but not the current one. For this purpose, it uses the getWindowHandles() and getWindowHandle() methods provided by Selenium. The getWindowHandles() method returns the Set
The allTabs set is then iterated over to switch to that particular tab and call the close() method for smooth closing of that tab. A condition (if(!selectedTab.equals(currentTab))) is checked to make sure that the current tab is not closed. This function also prints the title of the tab to be closed before calling the close() method.
The console output produced by running this automation script is shown in the image below.
Selenium Close Tab using Browser Shortcuts
Another common approach to closing tabs in Selenium is to invoke browser shortcuts. Although this method is not 100% reliable, it works on most popular and modern browsers. The default shortcut that is supported by Google Chrome, Mozilla Firefox, Microsoft Edge, and Safari browsers is:
- open tab: ctrl + T or cmd + T
- close tab: ctrl + W or cmd + W
In this example of the Selenium close tab, we will be using this shortcut to close the tab in the browser. We will be seeing the code in both Python and Java.
Selenium Close Tab using Browser Shortcuts in Python
In Python, it becomes effortless to execute keyboard shortcuts by importing the Keys class and using the send_keys() method. Let’s start with this simple example, opening and closing a tab using the browser shortcuts by creating a Python file.
Let us break down the code for a better understanding of the Selenium close tab using the browser shortcuts.
In this example, we imported the By class to locate elements on the web page and the Keys class to imitate keyboard button inputs.
The code below will open the specified URL (“https://ecommerce-playground.lambdatest.io/”) on the browser tab. It then prints the title of the opened page on the console using the print(chromedriver.title) method. After opening the page a time delay is added to pause the further execution for some time.
After printing the title of the page Selenium first finds the body of the web page opened using the find_element(By.TAG_NAME, “body”). Selenium then sends the browser command to press CTRL/COMMAND + W keys using the send_keys() method. Once this shortcut is pressed the browser will close the current tab. Here it also quits the browser session as no other tab is opened on the browser.
Selenium Close Tab using Browser Shortcuts in Java
In Selenium Java there is no direct method to trigger keyboard shortcuts like send_keys() in Python but instead, we can use the Action class to interact with the browser and simulate the keyboard shortcuts. Let’s get started with this example on Selenium close in Java using browser shortcuts by creating a Java class.
Let us break down the code for a better understanding of the Selenium close tab using the browser shortcuts. Here it goes:
Here, we will import the Action class from Selenium WebDriver – for automating low-level interactions using keyboard and mouse interrupts.
The code will open the specified URL (“https://ecommerce-playground.lambdatest.io/”) on the browser tab using the driver.get() method. It then prints the title of the opened page on the console using the driver.getTitle() method. Lastly, this code pauses the execution of further code by the Thread.sleep() method for proper loading and visibility. Any exception during Thread.sleep() was handled using the try-catch block.
After printing the page title, an instance of the Actions class was created using the default constructor. Next, Selenium sends the browser command to press CTRL/COMMAND + W keys using the Action class, keyDown(Keys.CONTROL.sendKeys(“w”) method. Once this shortcut is pressed the browser will close the current tab. Here it also quits the browser session as no other tab is opened on the browser.
Selenium Close Tab using JavascriptExecutor
In simple words, JavascriptExecutor is an interface that helps execute JavaScript with Selenium. To streamline the usage of JavascriptExecutor in Selenium, assume it as a medium that allows the WebDriver to interact with HTML components within the browser.
We can utilize this JavascriptExecuter to close tabs in Selenium. With the help of JavaScript commands like “window.close()” or “window.open()” we can close and open the browser tab. JavascriptExecutor is an interface available for all the languages supporting Selenium.
Note: Before proceeding with the example, please note that window.close() function would not close the tab if it was not opened via the window.open(). In other words, if we open the tab manually, we cannot use the window.close() approach with the help of browser.executeScript().
Selenium Close Tab using JavascriptExecutor in Python
Using JavascriptExecutor in Selenium WebDriver with Python is a cakewalk. Selenium in Python contains the execute_script() method to execute JavaScript code or commands which are passed as the argument to that method. And we previously discussed that the “window.close()” javascript command can close the current tab. To get started with the example let’s create a Python file.
Let us break down the code for a better understanding of the Selenium close tab using the JavaScript Executor. Here it goes:
The below mentioned code will then open the specified URL (“https://ecommerce-playground.lambdatest.io/”) on the browser tab. Then the code opens a different URL (“https://www.lambdatest.com”) in a new tab using the execute_script() method and passing the window.open() javascript command. The recently opened tab is stored in the popup_window variable.
Next, Selenium sends the browser command to execute the popup_window.cose() JavaScript command. Once the browser executes this command, it will close the last opened tab.
Selenium Close Tab using JavascriptExecutor in Java
In Selenium Java, we have the JavascriptExecutor class. A reference to this class can be created by typecasting the browser driver. Later on, we can use this reference to the JavascriptExecutor to call the executeScript() and pass the javascript command as an argument to that function. To get started with the example, let’s create a Java class.
Let us break down the code for a better understanding of the Selenium close tab using the JavascriptExecutor. Here it goes:
The below lines simply open the specified URL (“https://ecommerce-playground.lambdatest.io/”) in a browser tab using the driver.get() method. It also prints the title of the website opened using the driver.getTitle() method in the console.
The code below then creates a JavascriptExecutor class reference using the Chrome browser driver (JavascriptExecutor js = (JavascriptExecutor) driver;). Next, the code opens a new tab using the js JavascriptExtractor reference to execute the executeScript() method and pass the window.open() javascript command. The recently opened tab is stored in the popup_window variable. Lastly, it also prints the title of the newly opened page.
After printing the page title, Selenium sends the browser command to execute the popup_window.cose() JavaScript command. Once the browser executes this command, it will close the last opened tab.
Handling Alerts during Selenium Close Tab
There are times in the software testing life-cycle of some web applications when alerts are displayed on the web app to interrupt while closing it. These may be alerts, warnings, or information. Web browsers have native dialog boxes that can show alerts to users for these important notifications using JavaScript.
A common alert the browser throws while closing the tab is for unsaved changes or “Confirmation Dialogue”. A well-written automation script must ensure the alerts are handled properly before closing the tab. Moreover, sometimes ignoring these alerts may prevent the tab from getting closed.
This section will explore the methods and techniques to handle alerts during the Selenium close tab.
Handling Alerts during Selenium Close Tab in Python
Selenium WebDriver has an Alert class to handle any type of alert on the web page. Using this class, we may accept, dismiss, retrieve details, or send key values to handle the alerts on a web page. In Python, this class can be imported with the following commands.
1 2 |
# import Alert from selenium.webdriver.common.alert import Alert |
Here are the major functions that can be performed on a JavaScript alert in Selenium WebDriver Python during Selenium close tab:
- accept()
- dismiss()
- send_keys()
This is similar to pressing the “OK” button in the alert popup window.
1 2 3 |
# Initialize the alert in the website alert = driver.switch_to.alert alert.accept() |
This is used to cancel the alert, close the window, and pass control to the webpage.
1 2 3 |
# Initialize the alert in the website alert = driver.switch_to.alert alert.dismiss() |
This is for a prompt alert that requires input from the user. With this method, we can send text/keys as input to the alert box.
1 2 3 |
# Initialize the alert in the website alert = driver.switch_to.alert alert.send_keys('560085') |
Now that we have covered the fundamental aspects of Alerts in Selenium WebDriver during the Selenium close tab using Python, let’s get our hands dirty and understand how to handle them.
1 2 3 4 5 6 7 8 9 10 11 12 |
# Try to get alert from the web app alert = driver.switch_to.alert # Check if the alert is popped up or not if alert is not null: # Accept or decline the alert alert.accept() # alert.dismiss() else: print("No alert popped up") |
The above Python code can be used once the close() method or any other method to close a tab in Selenium Python is called. This code basically tries to access the alert from the browser driver by alert = driver.switch_to.alert. If the alert is not null then we can perform the desired operation on the alert to accept or dismiss it using the alert.accept() or alert.dismiss() method. Otherwise, if the alert is null then the tab will be closed uninterrupted.
If you want to learn more about handling alerts in Selenium using Python, please read – How to Handle JavaScript Alert in Selenium WebDriver Using Python?
Handling Alerts during Selenium Close Tab in Java
Selenium WebDriver in Java has an Alert class to handle any type of alert on the web page. Using this class we may accept, dismiss, retrieve details, or send key values with the intention to handle the alerts on a web page. In Java, this class can be imported with the following commands.
1 2 |
// Import Alert class import org.openqa.selenium.Alert; |
Just like Python in Java also we can perform the following major functions on a JavaScript alert in Selenium WebDriver during the Selenium close tab:
- >accept()
- dismiss()
- sendKeys()
- getText()
This is used to cancel the alert, close the window, and control is passed over to the webpage.
1 2 3 |
// Create an instance of Alert class Alert alert = driver.switchTo().alert(); alert.dismiss(); |
This is for a prompt alert that requires input from the user. With this method, we can send text/keys as input to the alert box.
1 2 3 |
// Create an instance of Alert class Alert alert = driver.switchTo().alert(); alert.sendKeys("Text"); |
This is used to get the text message from the confirmation alert box.
1 2 3 |
// Create an instance of Alert class Alert alert = driver.switchTo().alert(); alert.getText(); |
Now that we have covered the fundamental aspects of alerts in Selenium WebDriver during the Selenium close tab using Java, let’s understand the code to handle them.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
// Perform these operations after Selenium close tab // Try to get alert from the tab Alert alert = driver.switchTo().alert(); // Check if the alert is poped up or not if(alert != null){ // Perform operation on the alert alert.accept(); // alert.close() }else{ System.out.println("No alert displayed."); } |
The above Java code can be used once the method to close a tab in Selenium Java is called. It basically tries to access the alert from the browser driver by Alert alert = driver.switchTo().alert();. If the alert is not null then we can perform the desired operation on the alert to accept or dismiss it using the alert.accept() or alert.dismiss() method. Otherwise, if the alert is null, the tab will be closed uninterrupted.
At the end of this section, you can be assured that all the major techniques and methods for Selenium Close Tab are covered. So get your hands dirty by implementing these on your real project.
You can find the GitHub repository for all the above examples at this GitHub repo.
Selenium Close Tab on a Cloud-Based Testing Platform
Using a cloud-based platform is the best way to leverage Selenium automation and get the desired results seamlessly without worrying about installation and setup. LambdaTest is an AI-powered test orchestration and execution platform that lets you run manual and automated tests at scale on over 3000 real devices, browsers, and OS combinations. We can perform Python and Java Selenium close tab automation on LamdaTest’s Selenium based cloud grid.
To do so, you can get the value for the access token, username, and gridUrl upon registering with LambdaTest. The first step is to register on LambdaTest for free. After registration, you can find these credentials from the LambdaTest dashboard, as shown in the image below.
Once you have the credentials, you can declare your testing environment’s preferred capabilities. LambdaTest has a Desired Capabilities Generator that will provide you with the capabilities based on your selection of OS, browser version, and resolution. Below is an automation test script to close the current tab in Selenium with Python using LambdaTest.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 |
# Import required modules from selenium import webdriver from selenium.webdriver.chrome.options import Options as ChromeOptions import time # your_username and your_access_key is important # to run your test on LambdaTest your_username = "YOUR_LAMBDATEST_USERNAME" your_access_key = "YOUR_LAMBDATEST_ACCESS_TOKEN" # Capabilities define the Browser, name, version, # platform and other necessary details # Capabilities define the Browser, name, version, # platform and other necessary details options = { "platformName": "Windows 10", "user": your_username, "accessKey": your_access_key, "browserName": "Chrome", "browserVersion": "114.0", "selenium_version": "4.8.0", "name": "LambdaTest selenium close tab automation using python", "build": "LambdaTest selenium close tab", "video": True, "network": True, "console": True, "visual": True, "w3c": True, # inform to use latest Selenium 4 } def selenium_close_tab(): # Setup to run the test on the platform remote_url = "http://"+ your_username + ":" + your_access_key+ "@hub.lambdatest.com/wd/hub" browser_options = ChromeOptions() # adding the capability to the chrome browser_options.set_capability('LT:Options', options) print("Initializing remote driver on platform: " + options["platformName"] + " & browser: " + options["browserName"] + "on version: " + options["browserVersion"]) # initializing remote server driver = webdriver.Remote(command_executor=remote_url, options=browser_options) driver.get("https://ecommerce-playground.lambdatest.io/") time.sleep(5) print(driver.title) time.sleep(5) driver.close() selenium_close_tab() |
This is the only method that needs to be added or changed when executing your automation test script in LamdaTest’s cloud Selenium Grid. Mainly this function defines the desired capabilities of the system for testing. It also takes your_username and your_accesstoken obtained from LambdaTest. Lastly, this function uses these details to create the browser driver using webdriver.Remote() method.
Once this test is executed successfully. We can track our test case on the LambaTest dashboard as shown in the image below.
Conclusion
Selenium has plenty of techniques to close single or multiple tabs using both Java and Python as the programming language. We started the article by understanding the close() method in Selenium for closing the current tab. To advance our automation skills, we learned examples to close multiple tabs.
We also go in-depth to learn other hacky yet effective techniques to close tabs in Selenium, like using browser shortcuts (ctrl +w) and using JavascriptExecutor. Before concluding the article, we learned a very practical use case for handling alerts while Selenium close tab.
Additionally, We came to realize the pain point of running Selenium WebDriver, & Grid, locally. Moving to cloud based Selenium Grid such as LambdaTest will help you scale effortlessly, so you could reduce your build times significantly & ship products faster.
Frequently Asked Questions (FAQs)
How to close the current tab in Selenium Python?
To close the current tab in Selenium we can use the inbuilt close() method. The close() method is a Selenium WebDriver function that currently closes the browser window in focus. It is always recommended to use the close() function when multiple browser windows or tabs are open.
How to close multiple tabs in Selenium?
To close the multiple tabs in Selenium we can use the inbuilt Window Handles method. The window_handles method in Python returns the list of all tabs and the getWindowHandles() method in Java returns a set of all tabs. We can iterate over this list and the set to switch between them and close it.
What are various methods to close tabs in Selenium?
Selenium has the default method close() to close the open tab on the automated browser. Another method is a little hacky where we close the browser tab by invoking the browser shortcuts like ctrl + W or cmd + W. Selenium also provides JavascriptExecutor to execute JavaScript on the web app. We can pass the JavaScript command window.close() to close the open tab.
What is the difference between the close() and quit() methods in Selenium?
The close() method is a Selenium WebDriver function that currently closes the browser window in focus. It is always recommended to use the close() function when multiple browser windows or tabs are open. If only one tab is open in the entire browser, then the close() function will quit the complete browser session. While the quit() function quits the complete browser session directly with all its tabs or windows. The function is used when the developer or tester wants to end the program.
Got Questions? Drop them on LambdaTest Community. Visit now