How To Use Arrays.asList() In Java [With Examples]
Arun Gupta
Posted On: June 16, 2023
148684 Views
11 Min Read
Test automation can be quite challenging sometimes when dealing with complex test cases. Although there are multiple ways available in any programming language to solve a particular problem, you need to be vigilant that your solution follows the best and the most efficient coding practices.
A fundamental yet important approach while designing an automation testing framework is the parameterization of data-driven test cases. A data-driven test case can be invoked multiple times with a different set of data values, which are passed as arguments to the parameters defined in the test method to achieve parameterization.
Talking about Java as the programming language for automation testing, there are many ways available in which you can parameterize your tests with tools like Selenium WebDriver, Appium, etc. But there is one method that can primarily be used for this purpose: Arrays.asList() method.
Arrays.asList() in Java is an important method that acts as a bridge between the array and collection interface in Java and provides many ways to implement parameterization.
In this blog on Arrays.asList() in Java, we will explore how the Arrays.asList() in Java works and provide examples to illustrate its usage. Whether you’re a beginner or a seasoned Java developer, understanding Arrays.asList() in Java can make your coding more efficient and productive.
So, let’s dive in!
TABLE OF CONTENTS
What is Arrays.asList() in Java?
A Java array is a fixed-size data structure that stores elements of the same data type. As an object, it’s assignable to variables, passable as method parameters, and returnable from methods, providing a versatile means of handling sequences in Java programming.
The Arrays.asList() in Java can be used in multiple ways where you can pass an array, elements, and objects as arguments to the method. Let’s first understand its syntax and usage with the help of simple code examples. Post that, we’ll discuss the real-time examples related to test automation using Selenium with Java.
Syntax:
- public is an access modifier.
- static is a non-access modifier.
- List is the method object return type.
- asList is the method name.
- a is a method argument.
Arrays.asList() method examples
There are three ways in which you can use the Arrays.asList() in Java to implement parameterization by passing different argument types and then converting them into a list.
Array as an argument
The Arrays.asList() in Java can accept an array as an argument and convert it to a list. The array passed as an argument to the method must be of Wrapper Class type (Integer, Float, etc.) instead of primitive data type (int, float, etc.).
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
import java.util.Arrays; import java.util.List; public class ArraysAsListWithArrayExample { public static void main (String args[]) { //Initializing a string Array String arr[] = {"Java", "Python", "C", "Ruby"}; //Getting the list view of Array List <String> list = Arrays.asList(arr); //Printing the elements inside the list System.out.println(list); } } |
Output:
In this example, a String type array has been passed as an argument to the Arrays.asList() in Java for converting the array to a list of string elements.
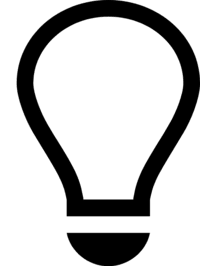
Test your Java automated scripts across 3000+ browser environments. Try LambdaTest Now!
Elements as an argument
Instead of passing the array, you can pass multiple comma-separated elements as arguments to the Arrays.asList() method.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
import java.util.Arrays; import java.util.List; public class ArraysAsListWithElementsExample { public static void main (String args[]) { //Getting the list view of String elements List <String> list = Arrays.asList("Java", "Python", "C", "Ruby"); //Printing the elements inside the list System.out.println(list); } } |
Output:
In the example shown above, multiple String type elements are directly passed as arguments to the method for converting them as a list of string elements.
Objects as an argument
There could be certain scenarios where you must create a list of objects of a self-defined class and then perform some action on that list.
The Arrays.asList() in Java allows you to pass class objects as arguments. It then creates a list of the objects you can use to perform specific actions.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
public class ProgrammingLanguage{ //Declaring the instance variables public String name; public double version; //Constructor to initialize the instance variables public ProgrammingLanguage(String name, double version) { this.name = name; this.version = version; } // Overriding toString method to return the String representation of the object public String toString() { return "(" + this.name + "," + this.version + ")"; } } |
In the ProgrammingLanguage class shown above, we are initializing the instance variables with the help of the constructor and overriding the toString() method to represent the objects in string form.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
import java.util.Arrays; import java.util.List; public class ArraysAsListWithObjectsExample { public static void main (String args[]) { //Getting the list view of ProgrammingLanguage class objects List <ProgrammingLanguage> list = Arrays.asList( new ProgrammingLanguage("Java", 17.0), new ProgrammingLanguage("Python", 3.10), new ProgrammingLanguage("C", 17.0), new ProgrammingLanguage("Ruby", 3.2) ); //Printing the objects inside the list System.out.println(list); } } |
Output:
In the class above, we are passing the objects of the ProgrammingLanguage class to the asList() method as arguments to get a list of these objects.
Arrays.asList() method usage in test automation
In this section of the Selenium Java tutorial, we will discuss the real-time examples in which we can use the Arrays.asList() in Java to parameterize our test cases.
Handling different driver instances
The basic capability of a UI testing framework is to execute the test cases on different browsers or platforms. This can be easily achieved using the concept of parameterization in the TestNG framework.
Feel free to choose any framework you are comfortable working with to implement the logic of handling multiple browsers. TestNG is one option, but if you are not familiar with it or prefer another framework, you can explore other alternatives.
The Arrays.asList() in Java can be used predominantly for this purpose. Assuming Selenium WebDriver as the automation testing tool in this example, we will see how we can write a logic for handling multiple driver instances for different browsers using the Arrays.asList() method.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 |
import java.util.Arrays; import java.util.List; import java.net.MalformedURLException; import java.net.URL; import org.openqa.selenium.remote.DesiredCapabilities; import org.openqa.selenium.remote.RemoteWebDriver; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.edge.EdgeDriver; import org.openqa.selenium.firefox.FirefoxDriver; public class DriverManager { private RemoteWebDriver driver; public static void init_driver(String browser) throws MalformedURLException { String username = System.getenv("LT_USERNAME") == null ? "Your LT Username" : System.getenv("LT_USERNAME"); String authkey = System.getenv("LT_ACCESS_KEY") == null ? "Your LT AccessKey" : System.getenv("LT_ACCESS_KEY"); // Create a list of browsers List<String> browsers = Arrays.asList("chrome", "firefox", "edge"); // Loop through the list of browsers and create a driver instance for each one for (String browserName : browsers) { if (browser.equalsIgnoreCase("chrome")) { //To launch the chrome browser on local /*driver = new ChromeDriver();*/ //Setting chrome capabilities DesiredCapabilities ChromeCaps = new DesiredCapabilities(); ChromeCaps.setCapability("platform", "Windows 10"); ChromeCaps.setCapability("browserName", "chrome"); ChromeCaps.setCapability("version", "latest"); ChromeCaps.setCapability("build", "TestNG With Java"); ChromeCaps.setCapability("name", m.getName() + this.getClass().getName()); ChromeCaps.setCapability("plugin", "git-testng"); //To launch the chrome browser on LambdaTest cloud grid String[] Tags = new String[]{"Feature", "Magicleap", "Severe"}; ChromeCaps.setCapability("tags", Tags); driver = new RemoteWebDriver(new URL("https://" + username + ":" + authkey + hub), ChromeCaps); } else if (browser.equalsIgnoreCase("firefox")) { //To launch the firefox browser on local /*driver = new FirefoxDriver();*/ //Setting firefox capabilities DesiredCapabilities FirefoxCaps = new DesiredCapabilities(); FirefoxCaps.setCapability("platform", "Windows 10"); FirefoxCaps.setCapability("browserName", "firefox"); FirefoxCaps.setCapability("version", "114.0"); FirefoxCaps.setCapability("build", "TestNG With Java"); FirefoxCaps.setCapability("name", m.getName() + this.getClass().getName()); FirefoxCaps.setCapability("plugin", "git-testng"); //To launch the firefox browser on LambdaTest cloud grid String[] Tags = new String[]{"Feature", "Magicleap", "Severe"}; FirefoxCaps.setCapability("tags", Tags); driver = new RemoteWebDriver(new URL("https://" + username + ":" + authkey + hub), FirefoxCaps); } else if (browser.equalsIgnoreCase("edge")) { //To launch the edge browser on local /*driver = new EdgeDriver();*/ //Setting edge capabilities DesiredCapabilities EdgeCaps = new DesiredCapabilities(); EdgeCaps.setCapability("platform", "Windows 10"); EdgeCaps.setCapability("browserName", "edge"); EdgeCaps.setCapability("version", "112.0"); EdgeCaps.setCapability("build", "TestNG With Java"); EdgeCaps.setCapability("name", m.getName() + this.getClass().getName()); EdgeCaps.setCapability("plugin", "git-testng"); //To launch the edge browser on LambdaTest cloud grid String[] Tags = new String[]{"Feature", "Magicleap", "Severe"}; EdgeCaps.setCapability("tags", Tags); driver = new RemoteWebDriver(new URL("https://" + username + ":" + authkey + hub), EdgeCaps); } else { throw new IllegalArgumentException("Invalid browser name: " + browser); } // Use the driver instance to navigate to a webpage and perform some actions driver.get("https://www.lambdatest.com"); System.out.println(driver.getTitle()); // Close the driver instance driver.quit(); } } } |
You will see that a list of browser names has been initialized with the help of the Arrays.asList() in Java. This list is then iterated through a for-each loop to instantiate the driver class of the respective browser.
You can run the tests on LambdaTest, a unified digital experience testing platform that allows you to perform Java automation testing on an online Selenium Grid across 3000+ browsers and real devices. You can generate automation capabilities for your tests via the LambdaTest capabilities generator. The code snippets of the capabilities, which are automatically generated using the capabilities generator, have been included in the test code above for reference.
Subscribe to our LambdaTest YouTube Channel for live updates with the latest tutorials around Selenium testing, Cypress testing, and more.
Validating multiple URL redirects
A very interesting scenario that I came across in one of my previous projects was to validate a security checklist that contained a list of URLs and their expected redirected URLs.
This list was huge and contained many URLs. The best way to test this scenario was to parameterize a test method with these URLs as arguments and open all of them one by one in the browser to check the redirected URL.
Although I used an Excel sheet as a data source for this test scenario, here I will try to provide a simplified code example using the Arrays.asList() method, which will take the URLs as arguments and print the redirected URLs on the console.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
import java.io.IOException; import java.util.Arrays; import java.util.List; import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; public class ValidateURLRedirect { public static void main(String args[]) throws IOException { //Creating ChromeDriver instance WebDriver driver = new ChromeDriver(); //Initializing a String array with URLs String URLs[] = {"https://app.lambdatest.com/", "https://accounts.lambdatest.com/detail/profile"}; //Passing the String array to the asList method as an argument List <String> URLlist = Arrays.asList(URLs); //Iterating through the list and printing the redirected URLs for (String URL: URLlist) { driver.get(URL); System.out.println(driver.getCurrentUrl()); } } } |
Output:
To gain access to these URLs, register for a free account and log in to your LambdaTest account.
Post logging in, you can see that this code is simply converting the array of URLs into a list using the Arrays.asList() method and then iterating the list using a for-each loop to open all the URLs one by one in the browser. Once the URLs are loaded, the current page URL is extracted and printed on the console.
Handling multiple web elements
There are certain scenarios in which you need to handle web elements at once to validate some functionality. A good example of such a scenario is to validate that there are no broken links or images on a webpage.
To build the logic for this scenario, you first need to collect all the links and then hit them one by one to check if the server is returning a 404 response code. The Arrays.asList() in Java again comes in handy here and does the job easily.
Here’s a code example for this.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 |
import java.io.IOException; import java.net.HttpURLConnection; import java.net.URL; import java.util.ArrayList; import java.util.Arrays; import java.util.List; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; public class ValidateLinksAndImagesExample { public static void main(String args[]) throws IOException { //Creating ChromeDriver instance WebDriver driver = new ChromeDriver(); //Opening the test URL driver.get("https://the-internet.herokuapp.com/broken_images"); //Initializing a list of img elements List<WebElement> allImages = driver.findElements(By.tagName("img")); //Creating a list to store the broken image URLs List<String> brokenImages = new ArrayList<String>(); //Iterating through the list of elements and hitting the img src URLs for (WebElement link : allImages) { String src = link.getAttribute("src"); System.out.println("Testing image source "+src); HttpURLConnection connection = (HttpURLConnection) new URL(src).openConnection(); connection.setRequestMethod("HEAD"); int responseCode = connection.getResponseCode(); if (responseCode >= 400) { //Adding all the broken image URLs to the list brokenImages.add(src); } } System.out.println("Broken images:"); //Printing the broken img URLs list System.out.println(Arrays.asList(brokenImages)); } } |
Output:
As you can see in this code example, all img elements present on the test URL are first collected into a list, and then their src URLs are extracted and hit one by one to assert the response code. All the URLs which returned 404 as a response code are added to another list, and then this list is printed on the console. There are currently two broken images available on this test URL, and the src URLs of both are printed in the console.
Bonus: Arrays.asList vs. new ArrayList(Arrays.asList())
Arrays.asList() and new ArrayList<>(Arrays.asList()) are two different ways of creating a List from an array in Java. Let’s discuss them in detail.
Arrays.asList()
Arrays.asList() in Java creates a list backed by the original array. This means that any changes made to the list will be reflected in the original array and vice versa. The resulting list is fixed-size and cannot be resized. If you need a mutable list, you need to create a new list based on the original list.
Mutable in Java refers to those objects whose state can be changed after they are created. In this case, a mutable list is a list that can be resized by adding or removing the elements. On the other hand, an immutable list is a list in which no structural changes can be made.
Below is the code example to demonstrate that any changes made to the list will also be reflected in the original array.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
import java.util.Arrays; import java.util.List; public class ModifyElementsExample { public static void main (String args[]) { //Initializing a string Array String [] arr = {"Java", "Python", "C", "Ruby"}; //Getting the list view of Array List <String> list = Arrays.asList(arr); //Modifying the value of thatist list.set(3, "C++"); //Printing the original Array System.out.println(Arrays.toString(arr)); } } |
Output:
Here you can see that the value of the third index of the list has been changed to “C++” from “Ruby” and when the original array was printed on the console, it also reflected the change.
The second example shown below is to prove that the list created using the Arrays.asList() in Java is immutable.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
import java.util.Arrays; import java.util.List; public class ModifyListExample { public static void main (String args[]) { //Initializing a string Array String [] arr = {"Java", "Python", "C", "Ruby"}; //Getting the list view of Array List <String> list = Arrays.asList(arr); //Modifying the structure of the list list.add("C++"); //Printing the List System.out.println(list); } } |
Output:
Here you can see that the program had thrown an UnsupportedOperationException when an attempt at the structural modification of the list was made using the add() method.
new ArrayList(Arrays.asList())
On the other hand, new ArrayList<>(Arrays.asList()) creates a new ArrayList based on the elements of the array. This means any changes to the new list will not affect the original array and vice versa. The resulting list is mutable and can be resized.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
import java.util.ArrayList; import java.util.Arrays; import java.util.List; public class ModifyNewListExample { public static void main (String args[]) { //Initializing a string Array String [] arr = {"Java", "Python", "C", "Ruby"}; //Getting the list view of Array List <String> list = new ArrayList<String>(Arrays.asList(arr)); //Modifying the list list.add("C++"); //Printing the original Array System.out.println(list); } } |
In the example above, the list has been modified successfully.
Output:
Conclusion
The Arrays.asList() in Java works as a bridge between the array and the collection interface. It converts an array into a fixed-size list, and any changes made to the values of the list elements will be reflected in the original array and vice versa.
This is a convenient method to implement parameterization in your tests, especially when you are not using other supporting libraries like TestNG in your framework.
Throughout this blog, we explored the syntax and usage of the Arrays.asList() in Java and provided examples to demonstrate its functionality.
Frequently Asked Questions (FAQs)
What is the asList() method of ArrayList in Java?
The asList method returns an ArrayList of a different type from Java’s util. ArrayList. The primary distinction is that the resulting ArrayList acts as a wrapper for an existing array; the add and remove functions are not included.
What type of list is Arrays.asList()?
asList returns a fixed-size list backed by the specified array; the returned list is serializable and allows random access.
Got Questions? Drop them on LambdaTest Community. Visit now