How to Get Element by Tag Name In Selenium
Sadhvi Singh
Posted On: April 18, 2019
83142 Views
5 Min Read
Selenium locators are your key when dealing with locating elements on a web page. From the list of locators like ID, Name, Class, tag name, XPath, CSS selector etc, one can choose any of these as per needs and locate the web element on a web page. Since ID’s, name, XPath or CSS selectors are more frequently used as compared to tag name or linktext, people majorly have less idea or no working experience of the latter locators. In this article, I will be detailing out the usage and real-time examples of How to Get Element by Tag Name locators In Selenium.
So, what is a tag name locator in Selenium?
A tag name is a part of a DOM structure where every element on a page is been defined via tag like input tag, button tag or anchor tag etc. Each tag has multiple attributes like ID, name, value class etc. As far as other locators in Selenium are concerned, we used these attributes values of the tag to locate elements. In the case of tag name Selenium locator, we will simply use the tag name to identify an element.
Below is the DOM structure of LambdaTest login page where I have highlighted the tag names:
Email Field: < input type="email" name="email" value="" placeholder="Email" required="required" autofocus="autofocus" class="form-control mt-3 form-control-lg">
Password Field: < input type="password" name="password" placeholder="Password" class="form-control mt-3 form-control-lg" >
Login Button: < button type="submit" class="btn btn-primary btn-lg btn-block mt-3">LOGIN< /button >
Forgot Password Link: < button type="submit" class="btn btn-primary btn-lg btn-block mt-3">LOGIN< /button >
Now the question that arises in one’s mind is, when do I use this tag name locator in Selenium? Well, in a scenario where you do not have attribute values like ID, class or name and you tend to locate an element, you may have to rely on using the tag name locator in Selenium. For example, in case you wish to retrieve data from a table, you may use < td >
tag or < tr >
tag to retrieve data.
Similarly, in a scenario where you wish to verify the number of links and validating whether they are working or not, you can choose to locate all such links through the anchor tag.
Please note: In a simple basic scenario where an element is located just via tag, it may lead to a lot of values being identified and may cause issues. In this case, Selenium will select or locate the first tag that matches the one provided from your end. So, refrain yourself from using tag name locator in Selenium if you intend to locate a single element.
The command to identify an element via tag name in Selenium is:
1 |
driver.findElement(By.tagName("input")); |
This certification is for anyone who wants to stay ahead among professionals who are growing their career in Selenium automation testing.
Here’s a short glimpse of the Selenium 101 certification from LambdaTest:
Real Time Scenarios Highlighting How to Get Element by Tag Name In Selenium
Scenario 1
A basic example, where we are locating the image avatar on ‘my profile’ section of LambdaTest:
Reference is the DOM structure of the avatar:
< img src="https://www.gravatar.com/avatar/daf7dc69b0d19124ed3f9bab946051f6.jpg?s=200&d=mm&r=g" alt="sadhvi" class="img-thumbnail" >
Let’s look into the code snippet now:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 |
package Chromedriver; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; public class Locator_By_Tagname { public static void main(String[] args) throws InterruptedException { // TODO Auto-generated method stub //Setting up chrome using chromedriver by setting its property System.setProperty("webdriver.chrome.driver", " Path of chromeDriver "); //Opening browser WebDriver driver= new ChromeDriver() ; //Opening window tab in maximize mode driver.manage().window().maximize(); //Opening application driver.get("https://accounts.lambdatest.com/login"); //Locating the email field element via Name tag and storing it in the webelement WebElement email_field=driver.findElement(By.name("email")); //Entering text into the email field email_field.sendKeys("sadhvisingh24@gmail.com"); //Locating the password field element via Name tag and storing it in the webelement WebElement password_field=driver.findElement(By.name("password")); //Entering text into the password field password_field.sendKeys("New1life"); //Clicking on the login button to login to the application WebElement login_button=driver.findElement(By.xpath("//button[text()='LOGIN']")); //Clicking on the 'login' button login_button.click(); //Clicking on the Settings option driver.findElement(By.xpath("//*[@id='app']/header/aside/ul/li[8]/a")).click(); //Waiting for the profile option to appear Thread.sleep(3500); //*[@id="app"]/header/aside/ul/li[8]/ul/li[1]/a //Clicking on the profile link driver.findElement(By.xpath("//*[@id='app']/header/aside/ul/li[8]/ul/li[1]/a")).click(); //Locating the element via img tag for the profile picture and storing it in the webelement WebElement image= driver.findElement(By.tagName("img")); //Printing text of Image alt attribute which is sadhvi System.out.println(image.getAttribute("alt")); } } |
Scenario 2
In this example, we will be verifying the number of links on the LambdaTest homepage and printing those link-texts:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 |
package Chromedriver; import java.util.List; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; public class Tagname_linktest { public static void main(String[] args) { // TODO Auto-generated method stub //Setting up chrome using chromedriver by setting its property System.setProperty("webdriver.chrome.driver", " Path of chromeDriver "); //Opening browser WebDriver driver= new ChromeDriver() ; //Opening window tab in maximize mode driver.manage().window().maximize(); //Opening application driver.get("https://www.lambdatest.com"); //storing the number of links in list List<WebElement> links= driver.findElements(By.tagName("a")); //storing the size of the links int i= links.size(); //Printing the size of the string System.out.println(i); for(int j=0; j<i; j++) { //Printing the links System.out.println(links.get(j).getText()); } //Closing the browser driver.close(); } } |
Below is a screenshot of the console:
Scenario 3
In this example, I will showcase when one wants to identify the number of rows in a table as during runtime this information can be dynamic and hence, we need to evaluate beforehand on the number of rows and then retrieve or validate the information.
Below is the DOM structure of the table:
< tbody class="yui3-datatable-data" >< tr id="yui_patched_v3_18_1_1_1554473988939_130" data-yui3-record="model_1" class="yui3-datatable-even" >
< tr id="yui_patched_v3_18_1_1_1554473988939_130" data-yui3-record="model_1" class="yui3-datatable-even">< td class="yui3-datatable-col-name yui3-datatable-cell ">John A. Smith< /td >
//……further rows continue//
Now let’s look into its code snippet:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 |
package Chromedriver; import java.util.List; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; public class Tagname_Row_data { public static void main(String[] args) { // TODO Auto-generated method stub //Setting up chrome using chromedriver by setting its property System.setProperty("webdriver.chrome.driver", "Path of chromeDriver"); //Opening browser WebDriver driver= new ChromeDriver() ; //Opening window tab in maximize mode driver.manage().window().maximize(); //Opening application driver.get("https://alloyui.com/examples/datatable"); //locating the number of rows of the table List<WebElement> rows= driver.findElements(By.tagName("tr")); //Printing the size of the rows System.out.print(rows.size()); //Closing the driver driver.close(); } } |
Snapshot of Console output:
Conclusion
As you can see, how I have used the tag name locator in Selenium on different scenarios. You can also use the tag name locator in combination with attribute value using XPath or CSS selectors. When it comes to other scenarios of locating elements, I may not suggest you using the tag name locator in Selenium but of-course scenarios mentioned above can really come in handy. Usage of tag name locator in Selenium may be limited, however, if you wish to be a proficient automation tester, then understanding how to use tag name locator and when to use it becomes very critical.
Enhance your Selenium interview proficiency with our meticulously curated compilation of questions and answers. Explore the comprehensive list of Selenium Interview Questions and Answers for valuable insights.
Got Questions? Drop them on LambdaTest Community. Visit now
Related Articles
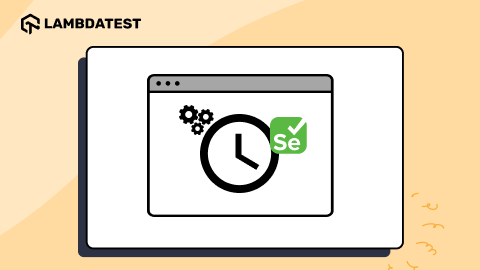
How to Use Thread.sleep() in Selenium
Faisal Khatri
April 25, 2024
95691 Views
20 Min Read
Selenium Java | Automation | Tutorial |
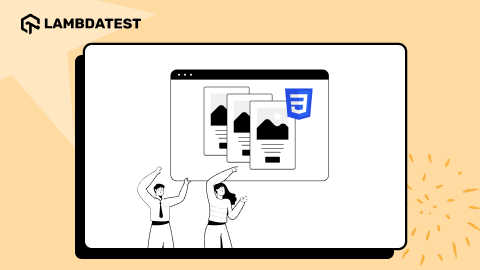
The Ultimate Guide to CSS Keyframes Animation
Adarsh M
April 22, 2024
51369 Views
35 Min Read
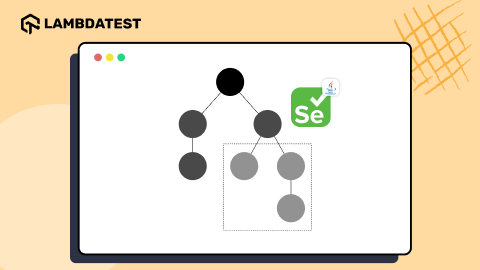
How to Handle Shadow Root in Selenium Java
Faisal Khatri
61082 Views
19 Min Read
Automation | Selenium Java | Tutorial |
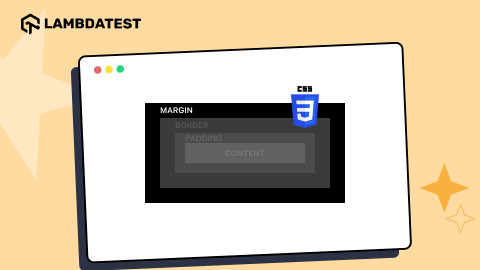
CSS Margins: All You Need to Know
Adarsh M
April 19, 2024
78244 Views
22 Min Read
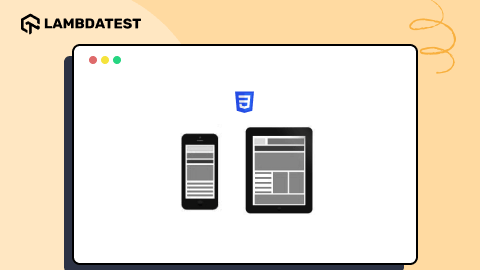
How to Set CSS Media Query For Portrait Orientation
Clinton Joy Fimie
April 18, 2024
89290 Views
23 Min Read
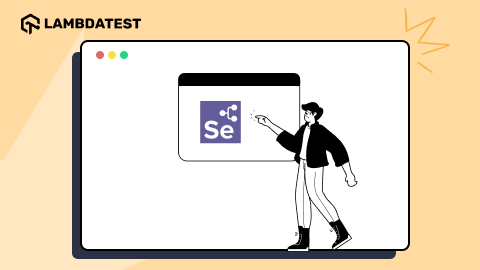
Selenium Grid Tutorial and Its Advantages
Himanshu Sheth
April 16, 2024
222719 Views
37 Min Read