A Beginner’s Guide To Mobile Design Patterns For Automation Testing
Sidharth Shukla
Posted On: July 5, 2023
171455 Views
22 Min Read
Mobile applications are growing in popularity, and testing them is becoming more challenging. Mobile automation testing is an important part of the testing process because it can help improve testing efficiency and reliability, resulting in a better user experience.
However, mobile automation testing can be challenging, and the available testing frameworks and tools can be complicated and time-consuming. Mobile Design Patterns can assist in overcoming these obstacles and increasing the effectiveness of mobile automation testing.
In this blog on Mobile Design Patterns, we’ll explore the benefits of using design patterns in mobile automation testing, as well as the various types of design patterns that can be used and how to implement them.
TABLE OF CONTENTS
What are Design Patterns?
Design patterns are similar to a set of instructions that explain how to use various code components to solve a problem. This way, instead of having to figure out how to solve the problem from scratch each time, you can use the same pattern to solve similar problems in the future. Let’s look at a simple example to help us understand.
You can also check out this video to learn Proxy and Adapter Design Patterns.
Assume you have a box of toys and want to play with them in various ways. Sometimes you want to construct a tower, other times a bridge, and still other times a house. Now, whenever you want to build something, you can use the same toys in different ways to make it. If you’re going to build a tower, for example, you can use the same blocks you used to build a bridge but arrange them differently.
Similarly, design patterns are instructions for how to use various pieces of code to solve various problems. In the same way, you can use the same pieces of toys in different ways to solve different problems. You can use the same pieces of code to solve different problems.
Types of Design Patterns for Mobile Automation Testing
There are several types of Mobile Design Patterns that can be applied to automation testing. Here are some of the most common:
Page Object Model (POM)
Page Object Model or POM is a popular design pattern for mobile automation testing. It involves creating a separate class for each page or screen in the mobile application and encapsulating the page-specific logic in that class. This can make tests more readable and maintainable and reduce the risk of code duplication.
Singleton
The Singleton design pattern is an excellent resource management tool for mobile app testing. It enables the construction of a single instance of a class, ensuring that it is accessible across the application. This is especially helpful when working with resources like databases or network connections, where several instances might lead to inefficiencies and potential conflicts. We can ensure that these resources are effectively handled and shared among different test cases or modules by utilizing the Singleton design.
Factory
Factory design patterns play an important part in software development, especially for pattern novices. This includes a separate class for creating objects, which is useful for generating test data and objects for mobile automation testing.
The Factory class simplifies object generation by encapsulating complex logic and giving clients a single interface. This abstraction enables flexibility and extensibility, allowing new creation methods or classes to be added without changing existing code. Mobile automation testers can successfully control object generation using the Factory design, resulting in more organized and maintainable testing operations.
Builder
Builder design patterns are a useful concept in software development, particularly for those new to Mobile Design Patterns. It entails building complex objects step by step. The Builder pattern is especially useful in mobile automation testing for constructing complex objects such as test scenarios or test cases.
It uses a Builder class with methods to set various properties or components of the object to provide a systematic approach. This allows for a fluid interface and method chaining for easy and readable object building.
Decorator
Decorator is a structural design pattern that lets you attach new behaviors to objects by placing these objects inside special wrapper objects that contain the behaviors. Composite and Decorator have similar structure diagrams because both use recursive composition to organize an infinite number of objects.
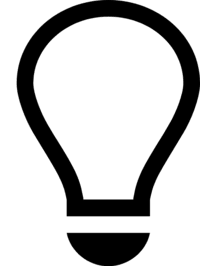
Run mobile automation tests on a real device cloud. Try LambdaTest Today!
Implementing Design Patterns in Mobile Automation Testing
Implementing Mobile Design Patterns in automation testing involves several steps. Here are some of the key steps:
Step 1: Identify the problem
Identifying the problem or challenge that needs to be addressed is the first step in implementing a Mobile Design Pattern. This could range from managing complexity to improving test reliability.
Step 2: Select a design pattern
Following the identification of the problem, the next step is to select the appropriate Mobile Design Pattern. This will be determined by the project’s specific requirements and the nature of the problem.
For instance, consider the scenario where you need to simulate and handle various mobile device configurations, such as screen sizes, resolutions, and orientations, to conduct thorough testing. In such cases, the Builder pattern proves invaluable. You can create device configuration objects with customizable parameters by leveraging the Builder pattern. This empowers you to dynamically generate diverse device configurations during test execution, enabling comprehensive and adaptable mobile testing.
Step 3: Implement the pattern
After deciding on a pattern, the next step is to put it into code. This will entail creating classes and methods that follow the pattern, as well as incorporating the pattern into the existing codebase.
Step 4: Test the pattern
Once the pattern has been implemented, it is critical to ensure that it functions as expected thoroughly. This will entail running automated tests and manually testing the application to ensure the pattern is functioning properly.
Step 5: Refactor the code
After testing and validating the pattern, the next step is to refactor the code to ensure that it is maintainable and scalable.
Page Object Model (POM) Pattern Implementation
The Page Object Model (POM) pattern is a design pattern commonly used in test automation to structure your code. The POM pattern can help you separate the logical components of your app and improve code reusability, maintainability, and scalability in the context of mobile automation. Here’s an example of the POM pattern in action in a Java-based mobile app testing framework:
First, create a Base class to initialize the driver and common methods that can be used by all the pages:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
public class BasePage { protected AppiumDriver<MobileElement> driver; public BasePage(AppiumDriver<MobileElement> driver) { this.driver = driver; } public void click(MobileElement element) { // Click logic here } public void sendKeys(MobileElement element, String value) { // Send keys logic here } // Other common methods here } |
Next, create a separate class for each page of your app, and extend the Base class:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
public class LoginPage extends BasePage { private MobileElement usernameField; private MobileElement passwordField; private MobileElement loginButton; public LoginPage(AppiumDriver<MobileElement> driver) { super(driver); PageFactory.initElements(new AppiumFieldDecorator(driver), this); } public void login(String username, String password) { sendKeys(usernameField, username); sendKeys(passwordField, password); click(loginButton); } // Other page-specific methods here } |
In the provided code example, the usage of PageFactory.initElements() is part of implementing the Page Object Model pattern. It is used to initialize the elements in the page object class (LoginPage) using the provided driver and decorator.
For those unfamiliar with PageFactory, it is essentially a class provided by Selenium WebDriver that facilitates the implementation of the Page Object Model. It offers methods like initElements() to initialize the elements defined in the page classes and associate them with the corresponding elements on the web page. Through the utilization of PageFactory, implementing the POM pattern becomes effortless, and instances of page classes can be created with their elements readily available for use.
In the LoginPage class, we define the specific elements on the page as instance variables and use the PageFactory class to initialize them with the @FindBy annotation. We also define a login method that accepts the username and password as parameters, enters them into the appropriate fields, and clicks the login button.
You can create other page classes similarly to represent other pages in your app.
Finally, you can create a separate class for your test cases and instantiate the appropriate page objects as needed:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
public class LoginTest { private AppiumDriver<MobileElement> driver; private LoginPage loginPage; @BeforeTest public void setUp() { // Set up driver and initialize login page object driver = new AppiumDriver<MobileElement>(...); loginPage = new LoginPage(driver); } @Test public void testLogin() { // Test login functionality loginPage.login("username", "password"); // Assert something here } @AfterTest public void tearDown() { // Quit driver driver.quit(); } } |
In this example, we initialize the LoginPage object in the setUp method and use it to test the login functionality in the testLogin method. Other test classes and methods can be created similarly to test other pages and functionality in your app.
The POM pattern enables you to separate your app’s logical components and improve code reusability, maintainability, and scalability in your mobile automation test framework.
Singleton Pattern Implementation
The Singleton design pattern ensures that a class has only one instance while providing a global point of access to that instance. Implementing the Singleton pattern in the context of mobile test automation can be useful in scenarios where you want to ensure that there is only one instance of a particular object or class throughout your test suite.
Watch this video to learn about the Singleton Design Pattern.
Here’s an example implementation of the Singleton pattern in a mobile test automation framework using Java:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
public class AppiumDriverSingleton { private static AppiumDriverSingleton instance = null; private AppiumDriver<MobileElement> driver; private AppiumDriverSingleton() { // Initialize driver here } public static AppiumDriverSingleton getInstance() { if (instance == null) { instance = new AppiumDriverSingleton(); } return instance; } public AppiumDriver<MobileElement> getDriver() { return driver; } } |
In this example, the AppiumDriverSingleton class represents a Singleton instance of an Appium driver, which can be used throughout the test suite. The getInstance method is used to retrieve the Singleton instance, and it checks if an instance already exists before creating a new one. The getDriver method is used to retrieve the driver instance from the Singleton.
To use the Singleton in your test suite, you can call AppiumDriverSingleton.getInstance().getDriver() to get the driver instance. This ensures that there is only one instance of the driver throughout your test suite and allows you to access the driver from any test case easily.
Factory Pattern Implementation
The Factory pattern is a creational Mobile Design Pattern that provides an interface for creating objects in a superclass while allowing subclasses to modify the type of objects created. This is useful when you need to create different types of objects but want to avoid hard-coding the object creation logic into your test code.
The Factory pattern can be used in a mobile test automation framework to create various types of mobile device objects, such as Android or iOS devices. Here’s an example of a Factory pattern implementation in Java:
- Create an interface or Abstract class for the Device factory, which defines a method for creating a new device object:
- Create concrete implementations of the Device factory for each device type, such as AndroidDeviceFactory and iOSDeviceFactory:
- Define the Device interface or Abstract class, which defines the common methods that all device types will implement:
- Create concrete implementations of the Device interface for each device type, such as AndroidDevice and iOSDevice:
- In your test code, create an instance of the Device factory for the device type you want to use:
- Use the Device factory to create a new device object:
- Call the common methods on the device object, which will be implemented differently depending on the device type:
1 2 3 |
public interface DeviceFactory { Device createDevice(); } |
1 2 3 4 5 6 7 8 9 10 11 12 13 |
public class AndroidDeviceFactory implements DeviceFactory { @Override public Device createDevice() { return new AndroidDevice(); } } public class iOSDeviceFactory implements DeviceFactory { @Override public Device createDevice() { return new iOSDevice(); } } |
1 2 3 4 |
public interface Device { void openApp(String appName); void clickButton(String buttonName); } |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
public class AndroidDevice implements Device { @Override public void openApp(String appName) { // Code to open app on Android device } @Override public void clickButton(String buttonName) { // Code to click button on Android device } } public class iOSDevice implements Device { @Override public void openApp(String appName) { // Code to open app on iOS device } @Override public void clickButton(String buttonName) { // Code to click button on iOS device } } |
1 |
DeviceFactory deviceFactory = new AndroidDeviceFactory(); |
1 |
Device device = deviceFactory.createDevice(); |
1 2 |
device.openApp("MyApp"); device.clickButton("Login"); |
You can easily switch between different types of devices in your test code by using the Factory pattern without having to change the code that creates the device objects or calls their methods. In the long run, this makes your code more flexible and easier to maintain.
Here’s end-to-end video on Repository and Factory Design Patterns, check it out!
Builder Pattern Implementation
The Builder pattern is a creational Mobile Design Pattern that lets you create complex objects by decoupling the construction process from the object’s representation. Implementing the Builder pattern in the context of mobile test automation can be useful in scenarios where you want to create complex objects, such as test data objects, in a flexible and maintainable manner.
Here’s an example of the Builder pattern in action in a Java-based mobile test automation framework:
First, create a Builder class for the object you want to create. In this example, we’ll create a User object:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 |
public class UserBuilder { private String username; private String password; private String firstName; private String lastName; private String email; public UserBuilder setUsername(String username) { this.username = username; return this; } public UserBuilder setPassword(String password) { this.password = password; return this; } public UserBuilder setFirstName(String firstName) { this.firstName = firstName; return this; } public UserBuilder setLastName(String lastName) { this.lastName = lastName; return this; } public UserBuilder setEmail(String email) { this.email = email; return this; } public User build() { return new User(username, password, firstName, lastName, email); } } |
In this example, the UserBuilder class is defined with instance variables for each property of the User object. To allow for method chaining, we define methods to set each property and return the builder object (UserBuilder). Finally, we define a build method that creates and returns a new User object based on the builder’s current state.
Create an object class (in this case, User) with a private constructor that accepts all of the properties as arguments:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
public class User { private String username; private String password; private String firstName; private String lastName; private String email; private User(String username, String password, String firstName, String lastName, String email) { this.username = username; this.password = password; this.firstName = firstName; this.lastName = lastName; this.email = email; } // Getter methods here } |
In this example, we define a private constructor for the User object that takes all of the properties as arguments. We also define getter methods for each property.
Finally, you can use the UserBuilder class to create User objects in a flexible and maintainable way:
1 2 3 4 5 6 7 |
User user = new UserBuilder() .setUsername("johnsmith") .setPassword("password123") .setFirstName("John") .setLastName("Smith") .setEmail("john.smith@example.com") .build(); |
In this example, we use method chaining to set the properties of the User object using the UserBuilder class and call the Build method to create a new User object with the specified properties.
The Builder pattern allows you to create complex objects in a flexible and maintainable way in your mobile test automation framework. You can use the same pattern to create other complex objects, such as test data or configuration objects.
Decorator Pattern Implementation
The Decorator pattern is a structural Mobile Design Pattern that allows you to attach additional responsibilities to an object dynamically. In the context of mobile test automation, implementing the Decorator pattern can be useful when you want to add additional functionality to an existing object without modifying its structure.
Watch this video to learn about the Decorator Design Pattern.
Here’s an example implementation of the Decorator pattern in a mobile test automation framework using Java:
First, create an interface for the object you want to decorate. In this example, we’ll create an interface for a MobileElement:
1 2 3 4 |
public interface MobileElement { void click(); String getText(); } |
In this example, we define the MobileElement interface with two methods: click() and getText().
Next, create a concrete implementation of the interface. In this example, we’ll create a BaseMobileElement class:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
public class BaseMobileElement implements MobileElement { private final WebElement element; public BaseMobileElement(WebElement element) { this.element = element; } @Override public void click() { element.click(); } @Override public String getText() { return element.getText(); } } |
In this example, we define the BaseMobileElement class that implements the MobileElement interface. We also define a constructor that takes a WebElement object and stores it in an instance variable. We then override the click() and getText() methods to delegate to the corresponding methods of the WebElement object.
Next, create a Decorator class that implements the same interface as the original object. In this example, we’ll create a HighlightMobileElement class that highlights the element before clicking it:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
public class HighlightMobileElement implements MobileElement { private final MobileElement element; public HighlightMobileElement(MobileElement element) { this.element = element; } @Override public void click() { highlight(); element.click(); } @Override public String getText() { return element.getText(); } private void highlight() { // Highlight the element here } } |
In this example, we define the HighlightMobileElement class that implements the MobileElement interface. We also define a constructor that takes a MobileElement object and stores it in an instance variable. We then override the click() method to highlight the element before delegating to the click() method of the Decorated element.
Finally, you can use the Decorator to add additional functionality to the original object:
1 2 |
MobileElement element = new HighlightMobileElement(new BaseMobileElement(driver.findElement(By.id("element-id")))); element.click(); |
In this example, we create a MobileElement object using the HighlightMobileElement Decorator, which adds highlighting functionality to the BaseMobileElement. We then call the click() method on the Decorated element.
The Decorator pattern allows you to add additional functionality to an object dynamically without modifying its structure. In your mobile test automation framework, you can use the same pattern to add additional functionality to existing objects, such as custom logging or error handling.
Best Practices of Mobile Design Pattern Implementation
It is critical to follow some best practices when implementing Mobile Design Patterns in a mobile test automation framework to ensure that the code is maintainable, scalable, and reusable. When implementing Mobile Design Patterns in a test automation framework, the following best practices should be followed:
Identify the problem domain
It is critical to understand the problem domain and the requirements of the mobile test automation framework before implementing a Mobile Design Pattern. This will assist you in selecting the appropriate pattern and ensuring that it meets the framework’s requirements.
Assume you have eCommerce features such as product searching, adding goods to the cart, the checkout process, and user account management. These functionalities must be handled efficiently by the framework. Based on these requirements, you may next select the best Mobile Design Pattern for the issue domain.
For example, you may opt for the Page Object Model (POM) pattern to create separate page classes for each eCommerce functionality. This allows for modular and reusable test cases, encapsulating the interactions and assertions within the corresponding page objects.
Make use of well-known patterns
It is critical to use widely used Mobile Design Patterns that have been proven to work in practice. This ensures that your code is robust and dependable, as well as that other developers can easily understand and modify it.
Consistently apply the pattern
Maintaining consistency in the usage of a Mobile Design Pattern within the framework is imperative. This practice guarantees code readability, comprehensibility, and adherence to a uniform coding style, thus fostering a cohesive and maintainable codebase.
Documentation
The Mobile Design Patterns used in the test automation framework must be documented. This documentation should include a description of the pattern, its function, and how it is implemented in the framework.
Test the patterns
It is essential to test the Mobile Design Patterns to ensure that they function as intended and do not introduce new bugs in the code. This testing should be automated and included in the framework for mobile test automation.
Cloud-based digital experience testing platform like LambdaTest lets you perform automated app testing using frameworks like Appium on over 3000+ real browsers and devices. You can test on LambdaTest real device cloud offering thousands of real Android and iOS devices and enabling the ultimate mobile experience. Ensure your website’s mobile responsiveness with LambdaTest’s Mobile-Friendly Test, a handy tool to optimize your user experience.
Subscribe to the LambdaTest YouTube Channel and stay up to date with the latest tutorials around Selenium testing, Cypress testing, and more.
Refactor the code
It is pivotal to refactor the code regularly to ensure that it is maintainable and scalable. This should include removing any duplicate code, simplifying complex code, and ensuring that the code adheres to the SOLID principles.
This entails eliminating duplicate code, streamlining complex code segments, and ensuring adherence to principles like Single Responsibility, Open/Closed, Liskov Substitution, Interface Segregation, and Dependency Inversion. In this article, we explore the significance of code refactoring and its pivotal role in successfully implementing Mobile Design Patterns.
Use version control
Version control is needed for handling the codebase of the mobile test automation framework. This allows you to keep track of code changes, revert to previous versions, and collaborate with other developers while working on different Mobile Design Patterns.
To summarise, implementing Mobile Design Patterns in a test automation framework generally requires a thorough understanding of the problem domain, the use of well-known patterns, the use of the patterns consistently, the documentation of the patterns, the testing of the patterns, the refactoring of the code, and the use of version control.
You can ensure that your mobile test automation framework is robust, reliable, and scalable by following these best practices.
Benefits of Mobile Design Patterns
Applying Mobile Design Patterns to automation testing can have several benefits. These include:
- Standardization
Mobile Design Patterns provide a standardized approach to addressing common problems in mobile automation testing. Teams can avoid reinventing the wheel by using patterns to ensure that their solutions are consistent and reusable.
- Scalability
Testing mobile automation can be complex and time-consuming. Mobile Design Patterns can aid in simplification by providing a framework for managing complexity and promoting scalability.
- Reusability
Mobile Design Patterns enable teams to create reusable code that can be used across multiple projects. In the long run, this can save time and effort while ensuring consistency and quality across projects.
- Maintainability
Mobile Design Patterns can improve code maintainability by encouraging modularity and encapsulation. This can make it easier for teams to manage changes and reduce the risk of introducing bugs or errors.
Note: Discover the secrets to flawless Mobile UI Testing in latest post! Dive into a comprehensive guide filled with expert insights and best practices.
Conclusion
In this mobile app testing tutorial, we learned how integrating Mobile Design Patterns into a mobile test automation framework can provide numerous benefits, including code reusability, maintainability, scalability, adherence to best practices, and consistency. Developers can reduce the time and effort required for test creation and maintenance by using design patterns to create code that is easier to read, understand, and modify.
Understanding the problem domain, selecting appropriate patterns, documenting the patterns, testing the patterns, and using version control are all necessary for successfully implementing design patterns in a mobile test automation framework. Additionally, developers should adhere to best practices such as using well-known patterns, repeating patterns, and refactoring code regularly to ensure maintainability and scalability.
Overall, design patterns can greatly improve the quality and efficiency of mobile test automation framework development, resulting in more reliable and robust test results. As a result, for maximum benefit, developers should become acquainted with commonly used design patterns and incorporate them into their mobile test automation frameworks.
Frequently Asked Questions (FAQs)
What is a mobile design pattern?
A mobile design pattern, also known as a mobile UI design pattern, refers to a recurring solution or approach designers and developers use to solve common design problems in mobile applications. Design patterns provide proven and effective solutions to design challenges, allowing for consistency, usability, and familiarity in mobile user interfaces.
What are design patterns in Android?
Design patterns in Android refer to reusable solutions that address common software design problems specific to Android application development. These patterns help structure code, organize components, and improve the overall architecture of Android applications. Some widely used design patterns in Android development include:
- Model-View-Controller (MVC)
- Model-View-Presenter (MVP)
- Model-View-ViewModel (MVVM)
- Singleton
- Observer/Observable
- Dependency Injection (DI)
- Repository
- ViewHolder
These design patterns help in creating maintainable, scalable, and testable Android applications by providing well-established solutions to common design challenges. It’s important to understand these patterns and choose the most appropriate ones based on your Android application’s specific requirements and architectural considerations.
Got Questions? Drop them on LambdaTest Community. Visit now